Unlocking the Potential: WordPress Programming Demystified
WordPress is one of the most popular content management systems (CMS) in the world, powering millions of websites. While it offers a user-friendly interface for managing content, unlocking its full potential requires diving into WordPress programming. In this blog, we will demystify the process and provide code samples to help you enhance your WordPress website.
1. Understanding WordPress Programming Basics
To unlock the potential of WordPress, it’s essential to grasp the basics of WordPress programming. Here are some key concepts to get you started:
1.1 Theme Development
A WordPress theme controls the visual appearance of your website. Learn how to create custom themes, modify existing ones, and use template tags effectively. Here’s an example of a basic theme structure:
Perl my-theme/ ??? style.css ??? index.php ??? functions.php
1.2 Theme Development
Plugins extend the functionality of WordPress. Discover how to develop custom plugins and leverage hooks, filters, and actions to add new features. Here’s a simple example of a plugin file structure:
Perl my-plugin/ ??? my-plugin.php ??? includes/ ??? functions.php ??? my-class.php
2. Exploring Advanced WordPress Programming Techniques
Once you have a solid understanding of the basics, it’s time to explore advanced techniques that can unlock even more potential:
2.1 Custom Post Types
Custom post types allow you to create unique content types beyond the standard posts and pages. Learn how to register and display custom post types using code like this:
Php function create_custom_post_type() { $args = array( 'public' => true, 'label' => 'Books', 'supports' => array( 'title', 'editor', 'thumbnail' ), ); register_post_type( 'book', $args ); } add_action( 'init', 'create_custom_post_type' );
2.2 Custom Taxonomies
Taxonomies help categorize and organize your content. Create custom taxonomies and associate them with your custom post types using code like this:
Php function create_custom_taxonomy() { $args = array( 'hierarchical' => true, 'label' => 'Genres', ); register_taxonomy( 'genre', 'book', $args ); } add_action( 'init', 'create_custom_taxonomy' );
3. Enhancing User Experience with WordPress Programming
Taking your website to the next level involves enhancing the user experience. Here are some examples of how WordPress programming can achieve that:
3.1 Custom Shortcodes
Shortcodes allow you to create dynamic content and reusable elements. Develop custom shortcodes to add interactive features to your posts and pages:
Php function my_custom_shortcode( $atts ) { $atts = shortcode_atts( array( 'param' => 'default_value', ), $atts ); // Process the shortcode logic return $output; } add_shortcode( 'my_shortcode', 'my_custom_shortcode' );
3.2 AJAX Integration
Integrate AJAX to create smooth and dynamic interactions on your website without reloading the entire page. Here’s a simplified example of an AJAX request in WordPress:
Javascript jQuery(document).ready(function($) { $('#my-button').click(function(e) { e.preventDefault(); $.ajax({ url: ajaxurl, type: 'POST', data: { action: 'my_ajax_action', // Add additional parameters if needed }, success: function(response) { // Process the response } }); }); });
Conclusion
WordPress programming can unlock the full potential of your website, enabling you to create custom themes, plugins, and enhance the user experience. By understanding the basics, exploring advanced techniques, and utilizing code samples, you can demystify WordPress programming and take your website to new heights. Dive in, experiment, and watch your WordPress site thrive!
Table of Contents
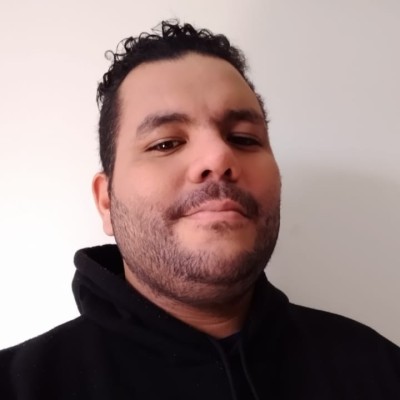
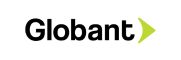