WordPress REST API: Extending Functionality with Programming
WordPress has been one of the most popular content management systems (CMS) for decades, powering millions of websites worldwide. Its flexibility, user-friendly interface, and extensive plugin ecosystem have made it a top choice for website owners. However, to truly harness the full potential of WordPress, developers often need to extend its capabilities beyond the built-in features. This is where the WordPress REST API comes into play.
The WordPress REST API allows developers to interact with the core functionalities of WordPress using simple HTTP requests. It opens up a world of possibilities for integrating WordPress with other applications, building custom interfaces, and automating various tasks. In this blog post, we will explore how you can extend WordPress functionality using programming with the WordPress REST API, along with code samples and step-by-step guides to get you started.
Understanding the WordPress REST API
Before diving into the nitty-gritty of extending WordPress functionality, it’s essential to grasp the basics of the REST API and how it works with WordPress.
What is the WordPress REST API?
The WordPress REST API is an interface that allows developers to access WordPress data and perform CRUD (Create, Read, Update, Delete) operations over HTTP. It follows the principles of Representational State Transfer (REST) and enables communication between different software applications.
Key Concepts of the WordPress REST API
- RESTful Endpoints: The WordPress REST API exposes various RESTful endpoints, which are URLs representing different resources within WordPress. For example, /wp-json/wp/v2/posts represents the endpoint for fetching posts.
- HTTP Methods: The API supports standard HTTP methods like GET, POST, PUT, DELETE, etc., to perform different operations on resources. For instance, GET is used to retrieve data, while POST is used to create new resources.
- JSON Data Format: The API uses JSON (JavaScript Object Notation) as its data format, making it lightweight and easy to work with in most programming languages.
- Authentication: To interact with protected endpoints or perform privileged actions, the API requires proper authentication, usually in the form of OAuth tokens or API keys.
Extending WordPress Functionality with the REST API
Now that we have a basic understanding of the WordPress REST API, let’s explore how we can extend WordPress functionality using programming. Below are some powerful ways to leverage the API to supercharge your WordPress website.
1. Create Custom Endpoints
While the WordPress REST API provides several default endpoints for common resources like posts, pages, and users, you might have specific data or functionalities unique to your website. By creating custom endpoints, you can expose and manage these resources programmatically.
Code Sample: Creating a Custom Endpoint
php // functions.php or a custom plugin function custom_api_endpoint() { register_rest_route('custom/v1', '/data/', array( 'methods' => 'GET', 'callback' => 'custom_api_callback', )); } function custom_api_callback($request) { // Custom logic to fetch data or perform actions $data = array('message' => 'Hello from custom endpoint!'); return new WP_REST_Response($data, 200); } add_action('rest_api_init', 'custom_api_endpoint');
In this example, we register a custom endpoint at /wp-json/custom/v1/data/. When accessed with the HTTP GET method, it will trigger the custom_api_callback function, allowing you to execute custom logic and return data in the JSON format.
2. Integrate External Applications
The WordPress REST API enables seamless integration between WordPress and external applications or services. You can use the API to communicate with WordPress from other platforms, mobile apps, or custom web applications.
Code Sample: Retrieving Posts from an External App
javascript // JavaScript Fetch API fetch('https://example.com/wp-json/wp/v2/posts') .then(response => response.json()) .then(data => { // Process the retrieved posts data console.log(data); }) .catch(error => console.error('Error:', error));
This JavaScript code snippet fetches the latest posts from a WordPress website using the REST API. You can use this data to display posts in your external application or perform other operations with it.
3. Build Custom Dashboards
Sometimes, the default WordPress admin dashboard might not offer the exact insights or functionality you need. By utilizing the REST API, you can create custom dashboards that display specific data or statistics relevant to your website.
Code Sample: Custom Dashboard using React
jsx // CustomDashboard.js import React, { useEffect, useState } from 'react'; const CustomDashboard = () => { const [stats, setStats] = useState({}); useEffect(() => { fetch('https://example.com/wp-json/wp/v2/stats') .then(response => response.json()) .then(data => setStats(data)) .catch(error => console.error('Error:', error)); }, []); return ( <div> <h2>Custom Dashboard</h2> <p>Number of posts: {stats.postCount}</p> <p>Number of users: {stats.userCount}</p> {/* Additional custom data and visualizations */} </div> ); }; export default CustomDashboard;
In this example, we create a custom dashboard using React that fetches site statistics from a custom endpoint (/wp-json/wp/v2/stats). This way, you can display essential metrics and enhance the website management experience.
4. Automate Content Management
With the WordPress REST API, you can automate various content management tasks, such as creating new posts, updating existing content, or deleting outdated entries.
Code Sample: Automating Post Creation
javascript // JavaScript with Axios library const axios = require('axios'); const newPost = { title: 'Automating WordPress Posts', content: 'This post was created using the REST API and automated scripting.', status: 'publish', }; axios.post('https://example.com/wp-json/wp/v2/posts', newPost, { headers: { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN', 'Content-Type': 'application/json', }, }) .then(response => console.log('Post created successfully:', response.data)) .catch(error => console.error('Error:', error));
This code snippet demonstrates how to create a new post on your WordPress website programmatically using the Axios library in JavaScript. You can adapt this example to automate other content management tasks as well.
Conclusion
The WordPress REST API is a game-changer when it comes to extending the functionality of your WordPress website with programming. By creating custom endpoints, integrating external applications, building custom dashboards, and automating content management, you can take your WordPress site to new heights.
In this blog post, we explored the fundamentals of the WordPress REST API, its key concepts, and how it can be leveraged for various use cases. We also provided code samples and step-by-step guides to help you get started with extending WordPress functionality through the API.
So, if you want to supercharge your WordPress website and add a touch of your programming magic, the WordPress REST API is the perfect tool to unleash your creativity and take your website to the next level. Happy coding!
Table of Contents
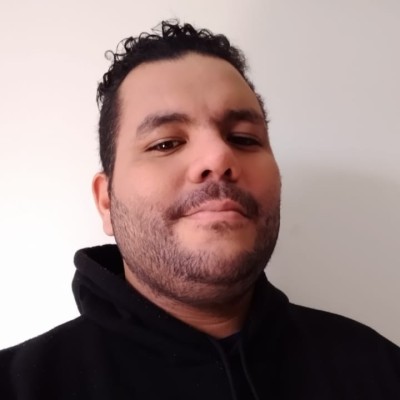
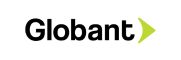