WordPress Security Plugins: Enhancing Protection with Programming
In the fast-paced world of the internet, where websites play a crucial role in personal and business communication, security is of paramount importance. With over 40% of websites powered by WordPress, it’s no wonder that this popular platform becomes a prime target for cyberattacks. Fortunately, WordPress security plugins come to the rescue, providing essential layers of protection against malicious activities. In this article, we’ll delve into the world of WordPress security plugins, and how you can further enhance your site’s protection through programming techniques.
Table of Contents
1. Understanding the Need for Security Plugins
WordPress, with its extensive plugin ecosystem, offers a multitude of options for enhancing security. From firewalls to malware scanners, security plugins act as a digital shield, safeguarding your website from various online threats. These threats include hacking attempts, DDoS attacks, malware injections, and more. Security plugins are like virtual guards that not only detect and prevent these attacks but also provide real-time monitoring and reporting.
2. Essential Security Plugins for WordPress
- Wordfence Security: One of the most popular and comprehensive security plugins, Wordfence Security offers features like firewall protection, malware scanning, login security, and real-time monitoring. It also includes an IP blacklist feature to block malicious IP addresses.
- Sucuri Security: Sucuri offers a website firewall, security activity auditing, malware scanning, and DDoS protection. It is known for its ability to clean and restore hacked websites effectively.
- iThemes Security: Formerly known as Better WP Security, this plugin provides a range of features, including brute force protection, file change detection, and two-factor authentication. It also offers database backups to ensure your data’s safety.
3. The Power of Programming: Customizing Security Measures
While security plugins offer excellent out-of-the-box protection, you can take your site’s security to the next level by incorporating programming techniques. Let’s explore how coding can enhance your site’s defense mechanisms.
3.1. Implementing Two-Factor Authentication (2FA)
Two-factor authentication adds an extra layer of security by requiring users to provide two different authentication factors. This could include something they know (password) and something they have (authentication code sent to their phone). While many security plugins offer 2FA as a feature, you can implement it programmatically as well.
php function custom_2fa_verification() { // Implement your custom 2FA logic here // Send authentication code to user's device // Verify the provided code // Grant access upon successful verification } add_action('wp_login', 'custom_2fa_verification');
3.2. Restricting Access with IP Whitelisting
Limiting access to your WordPress admin panel from specific IP addresses can significantly reduce the risk of unauthorized logins. While security plugins can help with this, you can also implement IP whitelisting through code.
php function restrict_admin_access() { $allowed_ips = array('192.168.1.1', '123.456.789.0'); // Add your allowed IP addresses $user_ip = $_SERVER['REMOTE_ADDR']; if (!in_array($user_ip, $allowed_ips)) { wp_die('Access denied.'); } } add_action('admin_init', 'restrict_admin_access');
3.3. Securing Database Access
Protecting your WordPress database is crucial to prevent data breaches. You can enhance database security by using prepared statements when querying the database.
php global $wpdb; $username = 'username'; $password = 'password'; $sql = $wpdb->prepare("SELECT * FROM wp_users WHERE user_login = %s AND user_pass = %s", $username, $password); $results = $wpdb->get_results($sql);
3.4. Enforcing Content Security Policies (CSP)
Content Security Policies help prevent cross-site scripting (XSS) attacks by specifying which sources of content are allowed to be loaded on a web page. You can add CSP headers to your site’s HTTP response through programming.
php function add_csp_header() { header("Content-Security-Policy: default-src 'self'; script-src 'self' 'unsafe-inline' cdn.example.com;"); } add_action('send_headers', 'add_csp_header');
4. Continuous Vigilance: Security Auditing and Monitoring
Programming techniques not only bolster your site’s protection but also enable continuous monitoring and auditing. By setting up automated security checks and monitoring scripts, you can quickly detect and respond to any anomalies.
php function security_audit() { // Perform regular security checks // Scan for file changes, suspicious activities, and vulnerabilities // Send alerts or notifications upon detection } // Schedule the security audit to run regularly if (!wp_next_scheduled('security_audit_event')) { wp_schedule_event(time(), 'daily', 'security_audit_event'); } add_action('security_audit_event', 'security_audit');
Conclusion
WordPress security plugins are invaluable tools in fortifying your website against the ever-evolving landscape of cyber threats. However, their effectiveness can be amplified through programming techniques that cater to your site’s unique security requirements. By implementing features like 2FA, IP whitelisting, database security, and CSP headers, you establish a robust defense mechanism. Additionally, continuous monitoring through programming ensures that your site remains safeguarded in real-time.
Remember, the digital realm is constantly changing, and so are the methods employed by hackers. Regular updates, a proactive approach, and a combination of security plugins and programming expertise will go a long way in maintaining the integrity of your WordPress website. Strengthen your site’s defenses today and enjoy a safer online presence tomorrow.
Table of Contents
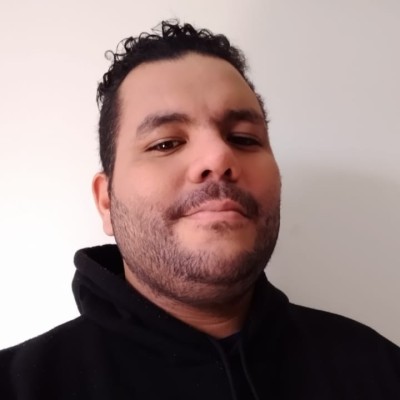
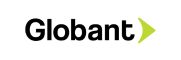