Mastering WordPress Template Hierarchy with Programming
WordPress, with its extensive ecosystem and user-friendly interface, has been the go-to choice for creating websites and blogs for years. Its flexibility lies not only in its user interface but also in the underlying structure that defines how content is displayed – the Template Hierarchy. If you’re a developer looking to take your WordPress skills to the next level, understanding and mastering the Template Hierarchy through programming is essential. In this guide, we’ll dive deep into the WordPress Template Hierarchy, explore its various components, and learn how to harness its potential to create highly customized websites.
Table of Contents
1. Introduction to WordPress Template Hierarchy
1.1. What is Template Hierarchy?
At its core, the WordPress Template Hierarchy is a system that dictates how WordPress chooses which template file to use when rendering different types of content. This hierarchy is defined by a set of rules that prioritize template files based on factors such as content type, page hierarchy, and available files in the theme.
1.2. Why is it important for developers?
For developers, mastering the Template Hierarchy unlocks a world of customization possibilities. By understanding how WordPress selects template files, you can control the layout and presentation of your site with precision. This knowledge is crucial when you want to create unique designs, optimize content display, or add specialized templates for specific sections.
2. Exploring the Core Template Files
2.1. The role of header.php and footer.php
The header.php and footer.php files are foundational to any WordPress theme. They provide the structure for the header and footer sections of your site, ensuring consistency across all pages. By editing these files, developers can include branding elements, navigation menus, and other common components.
php // header.php <!DOCTYPE html> <html <?php language_attributes(); ?>> <head> <meta charset="<?php bloginfo('charset'); ?>"> <title><?php wp_title(); ?></title> <?php wp_head(); ?> </head> <body <?php body_class(); ?>> <header> <!-- Your header content here --> </header> php // footer.php <footer> <!-- Your footer content here --> </footer> <?php wp_footer(); ?> </body> </html>
2.2. Understanding single.php and page.php
The single.php and page.php templates are responsible for rendering individual posts and static pages, respectively. These templates are vital for displaying content consistently and can be customized to match the overall theme design.
php // single.php while (have_posts()) : the_post(); get_template_part('content', get_post_format()); endwhile; php Copy code // page.php while (have_posts()) : the_post(); // Page content here endwhile;
3. Creating Custom Templates
3.1. Utilizing template files for specific content types
WordPress allows you to create custom templates for different content types. For example, if you want to style posts in a specific category differently, you can create a template file named category-{slug}.php.
php // category-sports.php while (have_posts()) : the_post(); // Custom layout for sports category endwhile;
3.2. The power of custom post type templates
Custom post types enable you to create content with unique structures. By creating custom templates for these post types, you can control how they are displayed. To create a template for a custom post type named “portfolio,” create a file named single-portfolio.php.
php // single-portfolio.php while (have_posts()) : the_post(); // Custom layout for portfolio items endwhile;
4. Leveraging Template Parts
4.1. Breaking down complex templates into parts
Template parts are reusable components that can be included in multiple templates. This promotes code reusability and maintainability.
php // content.php <article> <h2><?php the_title(); ?></h2> <div class="entry-content"> <?php the_content(); ?> </div> </article>
4.2. Dynamic loading of template parts
Template parts can be loaded dynamically based on conditions, offering a dynamic and flexible way to structure your templates.
php // single.php while (have_posts()) : the_post(); get_template_part('content', get_post_format()); endwhile;
5. Conditional Template Loading
5.1. Using conditional tags for targeted templates
WordPress provides conditional tags that allow you to load specific templates for certain scenarios.
php // archive.php if (is_category()) { get_template_part('category', 'sports'); } elseif (is_tag()) { get_template_part('tag', 'news'); } else { // Default content }
5.2. Implementing fallback templates gracefully
When a specific template is not available, WordPress falls back to more general templates. For instance, if a custom taxonomy template doesn’t exist, WordPress will use taxonomy.php.
6. Mastering Template Inheritance
6.1. Building parent and child themes
Parent and child themes enable you to separate the core functionality from the design. The parent theme contains the essential templates, while the child theme handles customizations.
php // style.css (Child Theme) /* Theme Name: My Child Theme Template: parent-theme-folder */
6.2. Overriding and extending parent theme templates
You can override specific templates from the parent theme in the child theme, allowing for targeted modifications without altering the parent’s core files.
7. Advanced Template Customization with Hooks
7.1. The role of action and filter hooks
Hooks provide entry points for injecting custom code into various parts of template rendering. Actions execute at specific points, while filters modify data before it’s displayed.
php // functions.php function custom_header_content() { echo '<div class="custom-header">Welcome to our site!</div>'; } add_action('my_header_hook', 'custom_header_content');
7.2. Injecting custom code into template parts
Hooks allow you to inject code directly into template parts, keeping your modifications organized and easily maintainable.
php // header.php <header> <?php do_action('my_header_hook'); ?> </header>
8. Programming Dynamic Template Hierarchy
8.1. Implementing context-aware template loading
With programming, you can dynamically load templates based on contextual information, such as user roles, device types, or custom conditions.
php // functions.php function load_custom_template() { if (is_user_logged_in()) { get_template_part('logged-in'); } else { get_template_part('logged-out'); } }
8.2. Creating dynamic layouts with programming
Programmatically adjusting template hierarchy allows you to create dynamic layouts that adapt to different scenarios.
9. Debugging and Troubleshooting
9.1. Tools for diagnosing template issues
Plugins like Query Monitor and Debug Bar provide insights into template loading, helping you identify and resolve template-related problems.
9.2. Common pitfalls and how to avoid them
Misplaced files, incorrect template names, and improper use of conditional tags can lead to unexpected template behavior. Regular testing and attention to detail can prevent such issues.
10. Best Practices for Efficient Development
10.1. Keeping your theme organized
A well-organized theme structure with appropriately named template files makes development and maintenance smoother.
10.2. Performance considerations in template hierarchy
Complex template hierarchies can impact performance. Minimize redundant template parts, optimize database queries, and leverage caching mechanisms for a faster site.
Conclusion
In conclusion, the WordPress Template Hierarchy is a powerful tool that empowers developers to craft unique and tailored website designs. By mastering the intricacies of this hierarchy through programming techniques, you can take your WordPress skills to new heights. Whether it’s creating custom templates, leveraging hooks for advanced customization, or implementing dynamic template loading, this guide has equipped you with the knowledge to excel in WordPress development. So go ahead, experiment, and create WordPress websites that stand out with a touch of programming mastery.
Table of Contents
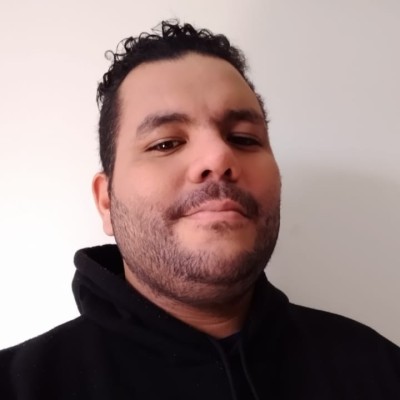
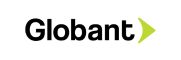