WordPress Theme Performance Optimization with Programming
WordPress has established itself as one of the most popular content management systems, powering a significant portion of websites across the internet. While its user-friendly interface and vast plugin ecosystem make it an appealing choice, ensuring optimal performance for your WordPress website, especially when using custom themes, is crucial. A slow-loading website can lead to higher bounce rates, reduced user engagement, and diminished search engine rankings. In this guide, we’ll delve into the world of WordPress theme performance optimization through effective programming practices.
Table of Contents
1. Introduction to Theme Performance
Website visitors have come to expect fast-loading pages, and studies have shown that users tend to abandon sites that take more than a couple of seconds to load. This is where performance optimization comes into play. The theme you choose plays a significant role, but even more important are the programming techniques and optimizations you implement within that theme.
2. The Impact of Performance on User Experience
Before we dive into the nitty-gritty of programming optimizations, let’s explore why performance matters so much. A website that loads quickly not only keeps users engaged but also positively impacts conversion rates. It’s all about providing a seamless user experience. From a programming perspective, here are some key factors that influence performance:
2.1. Minimizing HTTP Requests
Every HTTP request made by a browser to fetch resources (CSS, JavaScript, images, etc.) adds to the load time. As a programmer, you can significantly optimize performance by reducing the number of requests. Combine CSS and JavaScript files whenever possible and utilize sprites for images.
2.2. Efficient Database Queries
WordPress heavily relies on its database to store content, settings, and more. Inefficient database queries can slow down your website. Use caching mechanisms and ensure that your theme’s code makes use of optimized database queries.
2.3. Optimizing Images
Images are often the largest assets on a webpage. By compressing and properly formatting images, you can reduce load times considerably. Leverage modern image formats like WebP and use responsive images to cater to different device sizes.
2.4. Caching for Speed
Caching involves storing static versions of your website’s pages so they can be quickly served to users. Plugins like W3 Total Cache and WP Super Cache can help, but as a programmer, you can implement caching directly into your theme through code.
3. Effective Programming Practices for WordPress Theme Optimization
Now that we’ve outlined the importance of performance optimization, let’s delve into specific programming practices that can take your WordPress theme’s speed and efficiency to the next level.
3.1. Use a Lightweight Base Theme
When starting your theme development journey, begin with a lightweight base theme. This provides a solid foundation with minimal code bloat. Popular choices include Underscores (_s) and Sage. Building upon a lightweight base ensures that you’re only adding the necessary code for your website’s specific functionality.
3.2. Opt for a Minimalistic Design Approach
Simplicity not only enhances user experience but also contributes to better performance. Avoid overloading your theme with excessive design elements and features. Every line of code you include should serve a purpose.
3.3. Implement Lazy Loading
Lazy loading is a technique that defers the loading of non-critical resources, such as images below the fold, until they are about to come into the user’s view. This reduces initial page load time and saves bandwidth. Here’s a code snippet to implement lazy loading for images:
html <img src="placeholder.jpg" data-src="image.jpg" alt="A lazy-loaded image"> <script> document.addEventListener("DOMContentLoaded", function() { var lazyImages = [].slice.call(document.querySelectorAll("img[data-src]")); if ("IntersectionObserver" in window) { var lazyImageObserver = new IntersectionObserver(function(entries, observer) { entries.forEach(function(entry) { if (entry.isIntersecting) { var lazyImage = entry.target; lazyImage.src = lazyImage.dataset.src; lazyImage.classList.remove("lazy"); lazyImageObserver.unobserve(lazyImage); } }); }); lazyImages.forEach(function(lazyImage) { lazyImageObserver.observe(lazyImage); }); } }); </script>
3.4. Enqueue Scripts and Stylesheets Properly
WordPress provides the wp_enqueue_script() and wp_enqueue_style() functions to add scripts and stylesheets to your theme. Utilize these functions to ensure proper dependency management and to prevent loading unnecessary assets on every page.
3.5. Implement Gzip Compression
Gzip compression reduces the size of your website’s files, making them quicker to transfer. Most modern web servers support Gzip. To enable Gzip compression for your theme, add the following code to your .htaccess file:
apache <IfModule mod_deflate.c> AddOutputFilterByType DEFLATE text/text text/html text/plain text/xml text/css application/x-javascript application/javascript </IfModule>
3.6. Minify Your Code
Minification involves removing unnecessary characters from your code, such as whitespace and comments, to reduce its size. Smaller files load faster. Several online tools and WordPress plugins can help with code minification.
Conclusion
In the fast-paced digital landscape, optimizing your WordPress theme’s performance is not just an option – it’s a necessity. Through mindful programming practices and optimizations, you can ensure that your website loads quickly, provides an excellent user experience, and maintains high search engine rankings. Remember that every line of code has an impact, and by following the strategies outlined in this guide, you’ll be well on your way to crafting a lightning-fast WordPress theme.
Incorporate these techniques, stay updated with the latest best practices, and continuously monitor your website’s performance to identify areas for improvement. By prioritizing performance optimization in your theme development process, you’ll create a website that not only looks great but also delivers an exceptional user experience.
Table of Contents
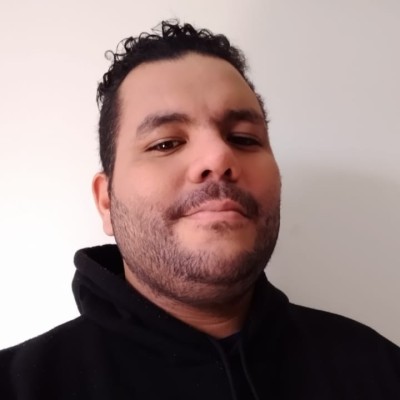
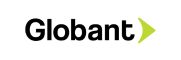