Integrating Third-Party APIs with WordPress Programming
WordPress, with its flexibility and ease of use, has become one of the most popular content management systems (CMS) for building websites and blogs. But what happens when you want to extend your website’s capabilities beyond its core features? This is where third-party APIs (Application Programming Interfaces) come into play. Integrating external APIs into your WordPress site can add a new layer of functionality, providing access to a wide range of services and data sources. In this guide, we’ll explore the process of integrating third-party APIs with WordPress programming, complete with code samples and step-by-step instructions.
Table of Contents
1. Why Integrate Third-Party APIs with WordPress?
Third-party APIs open up a world of possibilities for your WordPress website. Instead of reinventing the wheel, you can leverage existing services and data to enhance user experiences and streamline processes. Here are some compelling reasons to consider integrating third-party APIs:
1.1. Access to Rich Data:
By integrating APIs, you can tap into vast databases and resources, obtaining data that can be used to display real-time information on your site. For instance, weather forecasts, currency exchange rates, or social media feeds can enrich your content.
1.2. Enhanced Functionality:
APIs offer functionalities that might be complex to build from scratch. You can easily integrate payment gateways, map services, language translation, and more, extending your website’s capabilities without extensive coding.
1.3. Time and Cost Efficiency:
Integrating third-party APIs can significantly reduce development time and costs. Instead of writing complex algorithms, you can utilize pre-built solutions that are thoroughly tested and optimized.
1.4. User Engagement:
APIs can help you engage users more effectively. For example, integrating social media APIs allows users to share content seamlessly, enhancing your site’s reach and user interaction.
1.5. Real-Time Updates:
APIs provide real-time data updates. This is crucial for applications that require up-to-date information, such as stock market trackers, news websites, and live event coverage.
2. Exploring the Integration Process
Integrating third-party APIs with WordPress can be broken down into several key steps. Let’s walk through the process, from selecting an API to implementing it on your site.
Step 1: API Selection
The first step is to choose a suitable API for your project. Consider your website’s needs and objectives. Do you need a weather API, a payment gateway API, or perhaps a social media sharing API? Ensure the API you choose offers comprehensive documentation and support.
Step 2: Register and Obtain API Credentials
Most APIs require registration and provide unique credentials (API key, token, or secret) to access their services. Visit the API provider’s website, sign up, and generate the necessary credentials. Keep these credentials secure.
Step 3: Understanding API Endpoints
APIs provide endpoints – URLs where you can send requests to access specific data or services. Study the API documentation to understand the available endpoints, required parameters, and response formats.
Step 4: Implementing API Calls
WordPress provides several ways to make API calls, including the wp_remote_get() and wp_remote_post() functions. Here’s an example of how you can use these functions to make a simple API request:
php $response = wp_remote_get('https://api.example.com/data'); if (is_array($response)) { $data = json_decode($response['body']); // Process the data as needed }
In this example, we’re sending a GET request to the API’s endpoint and handling the response accordingly.
Step 5: Data Parsing and Display
Once you receive the API response, parse the data and display it on your WordPress site. This could involve creating custom templates or using hooks to insert data into existing pages or posts.
Step 6: Error Handling and Security
Implement proper error handling mechanisms to gracefully handle cases where the API is unavailable or returns errors. Additionally, ensure your API credentials are stored securely, preferably in the WordPress configuration file, away from public access.
3. Real-World Examples and Use Cases
Let’s delve into some real-world examples of integrating third-party APIs with WordPress.
Example 1: Weather Forecast
Imagine you run a travel blog and want to display the current weather of popular travel destinations. You can integrate a weather API like OpenWeatherMap. After obtaining your API key, you can create a shortcode that users can add to their posts to display the current weather:
php function weather_shortcode($atts) { $atts = shortcode_atts(array( 'city' => 'New York', ), $atts); $api_key = 'YOUR_API_KEY'; $url = "https://api.openweathermap.org/data/2.5/weather?q={$atts['city']}&appid={$api_key}"; $response = wp_remote_get($url); if (is_array($response)) { $data = json_decode($response['body']); $weather = $data->weather[0]->description; return "Current weather in {$atts['city']}: {$weather}"; } } add_shortcode('weather', 'weather_shortcode');
Example 2: Social Media Integration
Integrating social media sharing buttons on your blog posts can boost engagement. You can use the AddToAny sharing platform to achieve this. After registering and obtaining your sharing plugin code, you can add it to your WordPress theme’s template files for posts:
php // Inside single.php or content-single.php <div class="social-sharing"> <?php echo do_shortcode('[addtoany]'); ?> </div>
4. Tips for Successful API Integration
While integrating APIs can greatly enhance your WordPress website, here are some tips to ensure a smooth process:
4.1. Thorough Testing:
Before deploying API integrations to your live site, thoroughly test them on a staging environment. This helps identify and fix any issues before they affect user experience.
4.2. Caching and Performance:
API requests can impact page loading times. Implement caching mechanisms to store API responses temporarily and minimize the number of requests.
4.3. API Documentation:
Carefully read and understand the API documentation to ensure you’re using the correct endpoints, parameters, and authentication methods.
4.4. Update Management:
APIs might change over time, so ensure your integration remains functional by staying updated with changes made by the API provider.
4.5. Security Considerations:
Always prioritize the security of your API credentials. Keep them hidden and never expose them in your code repositories or public-facing files.
Conclusion
Integrating third-party APIs with WordPress can significantly enhance your website’s capabilities, delivering real-time data, enhanced functionalities, and improved user engagement. By following the steps outlined in this guide and taking into account best practices, you can seamlessly integrate APIs and provide a richer experience for your users. From weather forecasts to social media sharing, the possibilities are endless when you tap into the power of APIs. So go ahead, explore the APIs that align with your website’s goals, and take your WordPress site to the next level. Happy coding!
Remember, APIs can turn your WordPress website into a dynamic and feature-rich platform. By following the steps outlined in this guide and paying attention to best practices, you can seamlessly integrate APIs and provide a richer experience for your users. So go ahead, explore the APIs that align with your website’s goals, and take your WordPress site to the next level. Happy coding!
Table of Contents
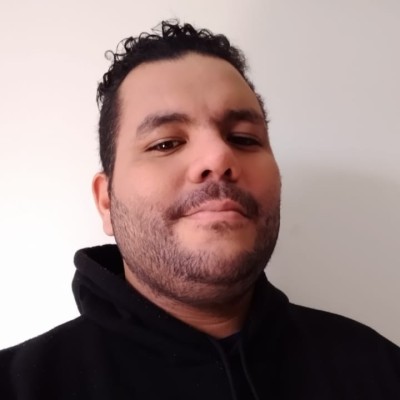
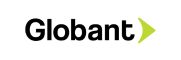