Ionic and Bluetooth: Building Apps for Connected Devices
In the rapidly evolving landscape of technology, the ability to connect and control various devices through mobile applications has become a game-changer. From smart home appliances to wearable fitness trackers, the demand for apps that seamlessly interact with connected devices is on the rise. Enter Ionic and Bluetooth – a powerful duo that enables developers to create feature-rich, connected device apps. In this comprehensive guide, we’ll explore how to leverage Ionic and Bluetooth to build apps that bridge the gap between the digital and physical worlds.
Table of Contents
1. The Significance of Connected Device Apps
Connected device apps, also known as IoT (Internet of Things) apps, have revolutionized the way we interact with technology. These apps enable users to control, monitor, and gather data from various devices through their smartphones or tablets. Whether it’s adjusting the temperature of your smart thermostat while you’re away from home or tracking your daily steps with a wearable fitness device, connected device apps have become an integral part of our daily lives.
2. Ionic and Bluetooth: A Winning Combination
Ionic, a popular open-source framework for building cross-platform mobile applications, and Bluetooth, a wireless communication technology standard, make an excellent combination for developing connected device apps. Ionic allows developers to create apps that work seamlessly on both iOS and Android devices, while Bluetooth provides the means to establish reliable connections with a wide range of devices, including sensors, wearables, and smart appliances.
In the following sections, we’ll dive into the world of Ionic and Bluetooth, guiding you through the process of building your own connected device app.
3. Getting Started with Ionic
Before we can delve into the intricacies of Bluetooth integration, it’s essential to have a basic understanding of Ionic and how to set up your development environment.
3.1. Setting Up Your Development Environment
To begin, ensure you have Node.js and npm (Node Package Manager) installed on your system. You’ll also need the Ionic CLI (Command Line Interface) to create and manage Ionic projects. You can install it globally using the following command:
bash npm install -g @ionic/cli
3.2. Creating a Basic Ionic App
Once the Ionic CLI is installed, you can create a new Ionic app using the following commands:
bash ionic start my-connected-device-app blank cd my-connected-device-app ionic serve
This will create a basic Ionic app template and start a development server, allowing you to preview your app in a web browser.
4. Understanding Bluetooth
4.1. Bluetooth Essentials
Bluetooth is a wireless communication technology that facilitates short-range data transmission between devices. It operates in the 2.4 GHz ISM (Industrial, Scientific, and Medical) band and is known for its low power consumption. Bluetooth technology is classified into various versions, with Bluetooth Low Energy (BLE) being the most commonly used for IoT and connected device applications.
4.2. Types of Bluetooth Connections
Bluetooth connections can be broadly categorized into two types: Central and Peripheral.
- Central: A central device, such as a smartphone or tablet, initiates connections to peripheral devices. It is responsible for scanning for nearby peripherals and establishing connections.
- Peripheral: A peripheral device, like a temperature sensor or smartwatch, advertises its presence and waits for a central device to connect. Once connected, it can transmit data to the central device.
In the context of connected device apps, your mobile device typically acts as the central device, while the devices you want to connect to, such as sensors or wearables, function as peripherals.
5. Ionic and Bluetooth Integration
Now that we have a basic understanding of Ionic and Bluetooth, it’s time to integrate Bluetooth into your Ionic project.
5.1. Adding Bluetooth to Your Ionic Project
To add Bluetooth capabilities to your Ionic app, you’ll need a Bluetooth plugin or library. One popular choice is the Cordova Bluetooth Serial plugin, which provides Bluetooth communication functionality for Cordova-based apps, including Ionic apps.
You can install this plugin by running the following command within your Ionic project directory:
bash ionic cordova plugin add cordova-plugin-bluetooth-serial npm install @ionic-native/bluetooth-serial
Next, you need to configure the plugin and import it into your app’s module. Open your app’s app.module.ts file and make the following additions:
typescript // Import the BluetoothSerial module import { BluetoothSerial } from '@ionic-native/bluetooth-serial/ngx'; // Add BluetoothSerial to the providers array @NgModule({ declarations: [AppComponent], entryComponents: [], imports: [BrowserModule, IonicModule.forRoot(), AppRoutingModule], providers: [ StatusBar, SplashScreen, BluetoothSerial, // Add this line { provide: RouteReuseStrategy, useClass: IonicRouteStrategy }, ], bootstrap: [AppComponent], }) export class AppModule {}
Now that you’ve added and configured the Bluetooth plugin, you’re ready to start working with Bluetooth devices in your Ionic app.
5.2. Establishing a Connection
To establish a Bluetooth connection with a peripheral device, you’ll typically go through the following steps:
- Scanning for Devices: Use the BluetoothSerial plugin to scan for nearby Bluetooth devices. This will return a list of available devices, including their names and unique identifiers (UUIDs).
- Connecting to a Device: Once you’ve identified the device you want to connect to, use the plugin to establish a connection by specifying the device’s UUID.
- Sending and Receiving Data: After a successful connection is established, you can send and receive data to and from the connected device.
Here’s a simplified example of how you can scan for nearby devices in your Ionic app:
typescript import { BluetoothSerial } from '@ionic-native/bluetooth-serial/ngx'; // ... // Function to scan for nearby Bluetooth devices scanForDevices() { this.bluetoothSerial.list().then( (devices) => { // 'devices' is an array of available Bluetooth devices devices.forEach((device) => { console.log(`Device Name: ${device.name}, UUID: ${device.id}`); }); }, (error) => { console.error(`Error scanning for devices: ${error}`); } ); }
In this code snippet, the BluetoothSerial module’s list method is used to scan for nearby devices and log their names and UUIDs to the console.
6. Building a Connected Device App
With the basics of Bluetooth integration covered, let’s move on to building a connected device app using Ionic.
6.1. Designing the User Interface
The user interface of your connected device app should provide a user-friendly way to interact with the connected devices. This includes displaying device information, status updates, and controls. Ionic offers a wide range of UI components and styling options to create a polished and responsive interface.
For example, you can use Ionic’s ion-list, ion-card, and ion-button components to design a simple interface for displaying connected devices and controlling them.
Here’s a snippet of Ionic HTML code for displaying a list of connected devices:
html <ion-content> <ion-list> <ion-item *ngFor="let device of devices"> <ion-label>{{ device.name }}</ion-label> <ion-button (click)="connectToDevice(device)">Connect</ion-button> </ion-item> </ion-list> </ion-content>
In this code, the ngFor directive is used to iterate through the devices array and display each device’s name along with a “Connect” button.
6.2. Sending and Receiving Data
Once you’ve established a connection with a Bluetooth device, you can start sending and receiving data. The specific data and commands will depend on the type of device you’re working with.
Here’s a simplified example of sending data to a connected Bluetooth device in your Ionic app:
typescript import { BluetoothSerial } from '@ionic-native/bluetooth-serial/ngx'; // ... // Function to send data to a connected device sendDataToDevice(device: any, data: string) { this.bluetoothSerial.write(data, device.id).then( (success) => { console.log(`Data sent successfully: ${success}`); }, (error) => { console.error(`Error sending data: ${error}`); } ); }
In this code, the BluetoothSerial module’s write method is used to send data to the connected device identified by its UUID.
7. Advanced Features
As you become more comfortable with building connected device apps using Ionic and Bluetooth, you can explore advanced features and functionalities.
7.1. Handling Multiple Devices
In real-world scenarios, you may need to connect and interact with multiple Bluetooth devices simultaneously. This can be achieved by managing multiple connections and data streams in your app. Keep in mind that the complexity of handling multiple devices may require careful design and resource management.
7.2. Real-time Data Updates
Many connected devices provide real-time data, such as sensor readings or status updates. To display and react to real-time data in your app, you can implement event listeners and callbacks that update the user interface whenever new data is received from connected devices.
8. Testing and Debugging
Testing and debugging are crucial steps in the app development process. Ensuring the compatibility of your connected device app across various devices and platforms is essential for a seamless user experience.
8.1. Ensuring Compatibility
Test your app on different iOS and Android devices to ensure compatibility. Ionic provides tools for previewing your app on various emulators and real devices. Additionally, consider using platform-specific testing frameworks and tools to identify and address any platform-specific issues.
8.2. Troubleshooting Common Issues
Bluetooth communication can be challenging, and you may encounter various issues during development. Some common issues include connectivity problems, data synchronization issues, and compatibility issues with specific Bluetooth devices. To troubleshoot these issues, consult documentation, seek help from developer communities, and use debugging tools to diagnose problems.
9. Security Considerations
Security is a paramount concern when building connected device apps, as they often involve the transmission of sensitive data between devices. Here are some key security considerations:
9.1. Protecting User Data
Implement encryption and secure authentication mechanisms to protect user data during Bluetooth communication. Ensure that data sent between the app and connected devices is encrypted to prevent eavesdropping and tampering.
9.2. Securing Bluetooth Communication
Bluetooth security standards have evolved over time, and it’s essential to stay updated with the latest security best practices. Be aware of security vulnerabilities and take measures to secure your app against potential threats.
10. Deployment and Distribution
Once you’ve developed and thoroughly tested your connected device app, it’s time to prepare it for deployment and distribution to users.
10.1. Preparing Your App for Release
Before releasing your app, make sure it meets all the necessary requirements for each platform (iOS and Android). This includes creating app icons, splash screens, and app store listings. You’ll also need to configure app permissions and ensure a smooth user experience.
10.2. Distribution Platforms
You can distribute your connected device app through various platforms, including the Apple App Store and Google Play Store. Follow the respective platform’s guidelines for app submission and review to ensure your app gets published successfully.
11. Future Trends
The world of connected device apps is continually evolving, with exciting trends and technologies on the horizon. As you embark on your journey to build connected device apps with Ionic and Bluetooth, keep an eye on emerging trends such as 5G connectivity, edge computing, and augmented reality (AR). These technologies are poised to further enhance the capabilities of connected device apps and open up new possibilities for innovation.
Conclusion
In this comprehensive guide, we’ve explored the powerful combination of Ionic and Bluetooth for building connected device apps. From setting up your development environment to integrating Bluetooth, designing user interfaces, and addressing security concerns, you now have the knowledge and tools to create seamless and secure connected device experiences.
As you venture into the world of connected devices, remember that innovation knows no bounds. With Ionic and Bluetooth at your disposal, you have the potential to bring groundbreaking connected device apps to life. So, get started today and be part of the ever-expanding ecosystem of connected devices that are shaping the future of technology. Happy coding!
In conclusion, Ionic and Bluetooth offer a compelling platform for building connected device apps, enabling developers to create innovative solutions that bridge the gap between the digital and physical worlds. By following the steps and best practices outlined in this guide, you can embark on your journey to create your own connected device app and contribute to the exciting landscape of IoT and smart devices.
Table of Contents
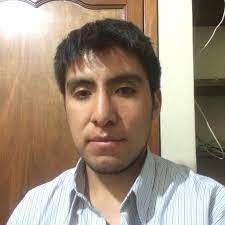
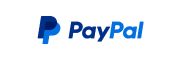