Ionic and Ad Networks: Integrating Ads in Your App
In the competitive world of mobile app development, generating revenue is a crucial aspect of success. One effective way to monetize your app is by integrating advertisements. By strategically incorporating ads into your Ionic app, you can increase your revenue stream while offering a seamless user experience. In this comprehensive guide, we will explore the integration of ad networks with Ionic, providing you with step-by-step instructions, code samples, and valuable insights to maximize your ad revenue potential.
Table of Contents
1. Choosing the Right Ad Network
Before diving into the technical details, you need to select the ad network that best suits your app and target audience. Here are three popular ad networks to consider:
1.1 AdMob
AdMob, owned by Google, is one of the most widely used ad networks for mobile apps. It offers a wide range of ad formats, including banners, interstitials, and rewarded ads. AdMob’s extensive advertiser network ensures a steady flow of ads and competitive earnings. To get started with AdMob, follow their documentation for Ionic integration.
1.2 Facebook Audience Network
If you’re looking for another powerhouse in the ad space, consider the Facebook Audience Network. It provides access to a vast pool of advertisers and offers high-quality ads that blend seamlessly with your app’s content. To integrate the Facebook Audience Network with your Ionic app, consult their official documentation.
1.3 Unity Ads
Unity Ads is an excellent choice if you’re developing mobile games with the Unity engine. It allows you to monetize your games through video and rewarded ads, offering a seamless integration process. To integrate Unity Ads into your Ionic game, follow the provided instructions in their documentation.
Once you’ve chosen the ad network that aligns with your app’s goals, you can proceed with the integration process.
2. Setting Up Your Ionic Project
Assuming you have an existing Ionic project or are starting from scratch, make sure you have the Ionic CLI installed. If not, you can install it globally using npm:
bash npm install -g @ionic/cli
Next, create a new Ionic project by running the following command:
bash ionic start YourAppName blank
Replace YourAppName with the desired name for your project. This command will create a new Ionic project with a blank template.
3. Installing Required Plugins
To integrate ads into your Ionic app, you’ll need to install the respective Cordova plugins for your chosen ad network. Let’s take AdMob as an example. To install the AdMob plugin, use the following command:
bash ionic cordova plugin add cordova-plugin-admob-free npm install @ionic-native/admob-free
This command adds the AdMob plugin to your project and installs the Ionic Native wrapper, which allows you to use the plugin’s functionality seamlessly in your Ionic app.
Repeat a similar process for other ad networks, ensuring you follow their specific installation instructions.
4. Implementing Banner Ads
Banner ads are one of the most common ad formats used in mobile apps. They are displayed at the top or bottom of the screen and are typically less intrusive than other ad types. To implement banner ads in your Ionic app, follow these steps:
4.1 Initialize the AdMob Plugin
In your app’s root component (usually app.component.ts), import the AdMob plugin and initialize it within the platform.ready() block:
javascript import { AdMobFree, AdMobFreeBannerConfig } from '@ionic-native/admob-free'; // ... constructor(platform: Platform, private admob: AdMobFree) { platform.ready().then(() => { // Initialize AdMob const bannerConfig: AdMobFreeBannerConfig = { id: 'your-admob-banner-id', autoShow: true, }; this.admob.banner.config(bannerConfig); }); }
Replace ‘your-admob-banner-id’ with the actual AdMob banner unit ID you obtained from your AdMob account.
4.2 Display the Banner Ad
Now, you can display the banner ad in your app. For instance, you can place it in your home page’s template (home.page.html):
html <ion-header> <ion-toolbar> <ion-title> Ionic Ad Example </ion-title> </ion-toolbar> </ion-header> <ion-content> <!-- Your app content goes here --> <!-- AdMob banner --> <div id="admob-banner"> <!-- AdMob banner will be displayed here --> </div> </ion-content>
In your home page’s TypeScript file (home.page.ts), show the banner ad when the page loads:
javascript import { Component } from '@angular/core'; import { AdMobFree } from '@ionic-native/admob-free'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage { constructor(private admob: AdMobFree) {} ionViewDidLoad() { // Show the banner ad this.admob.banner.show(); } // ... rest of your page logic ... }
With these steps, you’ve successfully integrated a banner ad into your Ionic app.
5. Integrating Interstitial Ads
Interstitial ads are fullscreen ads that appear at natural breaks in your app’s content, such as between game levels or when transitioning between app screens. Here’s how to integrate interstitial ads into your Ionic app:
5.1 Initialize the Interstitial Ad
In your app’s root component, initialize the interstitial ad similarly to how you did with the banner ad:
javascript // Import necessary modules import { AdMobFree, AdMobFreeInterstitialConfig } from '@ionic-native/admob-free'; // ... constructor(platform: Platform, private admob: AdMobFree) { platform.ready().then(() => { // Initialize AdMob const interstitialConfig: AdMobFreeInterstitialConfig = { id: 'your-admob-interstitial-id', autoShow: false, // We'll show it later manually }; this.admob.interstitial.config(interstitialConfig); }); }
Replace ‘your-admob-interstitial-id’ with the actual AdMob interstitial unit ID from your AdMob account.
5.2 Show the Interstitial Ad
You can display the interstitial ad at a suitable point in your app, such as when the user completes a game level. To do this, call the show() method:
javascript import { Component } from '@angular/core'; import { AdMobFree } from '@ionic-native/admob-free'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage { constructor(private admob: AdMobFree) {} // ... // Show the interstitial ad showInterstitialAd() { this.admob.interstitial.show().then(() => { // Interstitial ad displayed }).catch((error) => { console.error('Error displaying interstitial ad:', error); }); } }
This code snippet demonstrates how to integrate interstitial ads into your Ionic app. Remember to call showInterstitialAd() at an appropriate moment in your app’s flow.
6. Handling Rewarded Ads
Rewarded ads are an excellent way to engage users and boost revenue by offering them incentives for watching ads. To integrate rewarded ads into your Ionic app, follow these steps:
6.1 Initialize the Rewarded Ad
Initialize the rewarded ad in your root component, similar to the previous examples:
javascript // Import necessary modules import { AdMobFree, AdMobFreeRewardVideoConfig } from '@ionic-native/admob-free'; // ... constructor(platform: Platform, private admob: AdMobFree) { platform.ready().then(() => { // Initialize AdMob const rewardedConfig: AdMobFreeRewardVideoConfig = { id: 'your-admob-rewarded-id', autoShow: false, // We'll show it later manually }; this.admob.rewardVideo.config(rewardedConfig); }); }
Replace ‘your-admob-rewarded-id’ with the actual AdMob rewarded ad unit ID from your AdMob account.
6.2 Show the Rewarded Ad
To display the rewarded ad when a user chooses to watch it, create a function in your page’s TypeScript file:
javascript import { Component } from '@angular/core'; import { AdMobFree } from '@ionic-native/admob-free'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage { constructor(private admob: AdMobFree) {} // ... // Show the rewarded ad showRewardedAd() { this.admob.rewardVideo.show().then(() => { // Rewarded ad displayed }).catch((error) => { console.error('Error displaying rewarded ad:', error); }); } }
Now you have a function to show the rewarded ad when needed in your Ionic app.
7. Ad Placement Strategies
Effectively placing ads within your app can significantly impact your revenue and user experience. Here are some ad placement strategies to consider:
7.1 Balance User Experience
While ads can generate revenue, an excessive number of ads can annoy users. Strive for a balance between ads and user experience. Ensure that ads do not disrupt the app’s core functionality.
7.2 Offer Rewarded Ads
Consider offering users the option to watch rewarded ads voluntarily in exchange for in-app rewards or premium content. This provides value to users and boosts ad engagement.
7.3 Optimize Ad Timing
Place interstitial ads at natural breakpoints in your app, such as between levels or when transitioning between screens. Avoid interrupting critical user actions.
7.4 A/B Testing
Experiment with different ad formats, placements, and frequency to identify what works best for your specific audience. A/B testing can help you refine your ad strategy.
8. Monetization Tips and Best Practices
To maximize your app’s ad revenue, keep these tips and best practices in mind:
- Frequent Updates: Regularly update your app to fix bugs and introduce new features. Fresh content attracts users and keeps them engaged with your app and its ads.
- Optimize for Performance: Ensure that your app runs smoothly even with ads. Slow or buggy apps can lead to user dissatisfaction.
- Ad Mediation: If you use multiple ad networks, consider using ad mediation platforms like AdMob Mediation to optimize your ad inventory and maximize revenue.
- Comply with Policies: Adhere to the ad network’s policies and guidelines to prevent your app from being suspended or banned.
- Analyze User Behavior: Use analytics tools to understand user behavior and preferences. This data can help you tailor your ad strategy.
Conclusion
Integrating ads into your Ionic app can be a lucrative way to generate revenue while offering your users a free or low-cost experience. By carefully selecting the right ad network, implementing various ad formats, and following best practices, you can strike a balance between profitability and user satisfaction. Remember to continually monitor and optimize your ad strategy to ensure long-term success in the competitive mobile app market.
In this blog post, we’ve covered the basics of integrating ads into your Ionic app, from choosing the right ad network to implementing banner, interstitial, and rewarded ads. Use the provided code samples and guidelines to kickstart your ad integration journey and start monetizing your Ionic app effectively.
Table of Contents
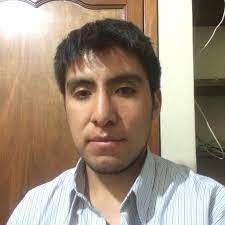
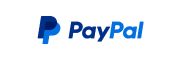