Ionic and In-App Purchases: Unlocking Premium Features
In today’s mobile app landscape, offering free apps with the option to unlock premium features through in-app purchases has become a common monetization strategy. Whether you’re a seasoned app developer or just starting, incorporating in-app purchases into your Ionic app can significantly boost your revenue stream.
Table of Contents
In this comprehensive guide, we will explore how to leverage the power of in-app purchases within Ionic apps, taking your user experience to the next level while maximizing your income potential.
1. Understanding In-App Purchases
Before we dive into the implementation details, let’s clarify what in-app purchases are and why they are essential for app developers.
1.1. What Are In-App Purchases?
In-app purchases (IAPs) are a mechanism that allows users to buy additional content or features from within your app. These purchases can take various forms, including:
- Consumable Purchases: Items that can be bought and used multiple times, such as virtual currency or power-ups in a game.
- Non-Consumable Purchases: Items that are bought once and retained permanently, like removing ads or unlocking premium features.
- Subscription Purchases: Users pay a recurring fee to access content or features, typically on a monthly or yearly basis.
- Auto-Renewable Subscriptions: A special type of subscription that renews automatically unless the user cancels it.
1.2. Why Implement In-App Purchases?
In-app purchases offer several advantages for app developers:
- Monetization: Generate revenue by offering additional content or features for a fee.
- User Engagement: Encourage users to spend more time within your app as they unlock new functionalities.
- User Retention: Keep users engaged and subscribed with regularly updated premium content.
- Flexibility: Adapt your monetization strategy based on user preferences and market trends.
Now that we understand the importance of in-app purchases let’s explore how to implement them in your Ionic app.
2. Prerequisites for Implementing In-App Purchases
Before diving into the code, you need to set up a few prerequisites for implementing in-app purchases successfully.
2.1. Apple Developer Account
For iOS apps, you’ll need an Apple Developer account to configure and manage in-app purchases through App Store Connect.
2.2. Google Play Developer Account
For Android apps, a Google Play Developer account is necessary to set up and manage in-app purchases through the Google Play Console.
2.3. Ionic Framework Installed
Ensure you have the Ionic framework installed on your development machine. If not, you can install it by running:
bash npm install -g @ionic/cli
2.4. Ionic Native In-App Purchase Plugin
Ionic offers a plugin called @ionic-native/in-app-purchase that simplifies the implementation of in-app purchases. Install it in your Ionic project by running:
bash ionic cordova plugin add cc.fovea.cordova.purchase npm install @ionic-native/in-app-purchase
With these prerequisites in place, let’s move on to the implementation of in-app purchases in your Ionic app.
3. Implementing In-App Purchases in Your Ionic App
In this section, we’ll guide you through the process of integrating in-app purchases into your Ionic app step by step. We’ll focus on non-consumable purchases, which unlock premium features indefinitely.
3.1. Set Up Your Product(s)
Before you can sell anything in your app, you need to define what users can purchase. In the context of non-consumable purchases, this means specifying the premium features or content users can unlock.
For iOS, you can configure your products in App Store Connect. For Android, use the Google Play Console. Each platform has its own interface for defining products and pricing.
3.2. Initialize the In-App Purchase Plugin
In your Ionic app, you should initialize the in-app purchase plugin. You can do this in your app’s main component or wherever it makes sense in your codebase.
javascript import { Component } from '@angular/core'; import { InAppPurchase } from '@ionic-native/in-app-purchase/ngx'; @Component({ selector: 'app-root', templateUrl: 'app.component.html', }) export class AppComponent { constructor(private iap: InAppPurchase) { this.initializeIAP(); } initializeIAP() { this.iap .register({ id: 'your_product_id_here', alias: 'premium_feature', type: this.iap.PAID_SUBSCRIPTION, }) .then(() => { // Successfully initialized }) .catch((error) => { console.error('Initialization failed: ' + error); }); } }
In this example, we register a non-consumable product with the ID ‘your_product_id_here’ and alias ‘premium_feature.’ We specify the purchase type as PAID_SUBSCRIPTION, which is suitable for non-consumable purchases.
3.3. Triggering the Purchase
Now, let’s implement a mechanism in your app to allow users to trigger the purchase of the premium feature. This can be done through a button or a menu item, depending on your app’s design.
javascript import { Component } from '@angular/core'; import { InAppPurchase } from '@ionic-native/in-app-purchase/ngx'; @Component({ selector: 'app-root', templateUrl: 'app.component.html', }) export class AppComponent { constructor(private iap: InAppPurchase) { this.initializeIAP(); } initializeIAP() { // Initialization code here... } purchasePremiumFeature() { this.iap .buy('premium_feature') .then((result) => { console.log('Purchase successful: ' + JSON.stringify(result)); // Unlock premium feature for the user }) .catch((error) => { console.error('Purchase failed: ' + JSON.stringify(error)); }); } }
In the purchasePremiumFeature method, we call iap.buy(‘premium_feature’) to initiate the purchase process. When the purchase is successful, you can unlock the premium feature for the user.
3.4. Handling Purchases
After the user makes a purchase, you need to validate and process it. This usually involves verifying the purchase with your server or a backend service to ensure its legitimacy. Here’s a simplified example:
javascript import { Component } from '@angular/core'; import { InAppPurchase } from '@ionic-native/in-app-purchase/ngx'; @Component({ selector: 'app-root', templateUrl: 'app.component.html', }) export class AppComponent { constructor(private iap: InAppPurchase) { this.initializeIAP(); } initializeIAP() { // Initialization code here... } purchasePremiumFeature() { this.iap .buy('premium_feature') .then((result) => { console.log('Purchase successful: ' + JSON.stringify(result)); // Call your server to validate the purchase this.validatePurchase(result.transaction, result.productType); }) .catch((error) => { console.error('Purchase failed: ' + JSON.stringify(error)); }); } validatePurchase(transaction: any, productType: string) { // Send the transaction data to your server for validation // If the purchase is valid, unlock the premium feature // Otherwise, handle the error appropriately } }
In the validatePurchase method, you should send the purchase transaction data to your server for validation. If the purchase is valid, you can unlock the premium feature for the user. Otherwise, handle the error gracefully.
3.5. Restoring Purchases
It’s essential to provide a way for users to restore their purchases if they reinstall the app or switch devices. Implement a “Restore Purchases” button or menu option to enable this functionality.
javascript import { Component } from '@angular/core'; import { InAppPurchase } from '@ionic-native/in-app-purchase/ngx'; @Component({ selector: 'app-root', templateUrl: 'app.component.html', }) export class AppComponent { constructor(private iap: InAppPurchase) { this.initializeIAP(); } initializeIAP() { // Initialization code here... } purchasePremiumFeature() { // Purchase code here... } restorePurchases() { this.iap .restorePurchases() .then((purchases) => { console.log('Restored purchases: ' + JSON.stringify(purchases)); // Check and unlock premium features for the user }) .catch((error) => { console.error('Restore failed: ' + JSON.stringify(error)); }); } }
In the restorePurchases method, calling iap.restorePurchases() will retrieve the user’s previous purchases, allowing you to check and unlock any premium features they’ve purchased.
Conclusion
Implementing in-app purchases in your Ionic app is a powerful way to monetize your hard work while offering valuable premium features to your users. By following the steps outlined in this guide, you can integrate in-app purchases seamlessly and maximize your app’s revenue potential.
Remember that a smooth and user-friendly purchase experience is crucial for retaining users and encouraging them to invest in your app. Test your in-app purchase flow thoroughly to ensure a seamless experience, and always provide excellent customer support to address any issues users may encounter during the purchase process.
So, go ahead and unlock the full potential of your Ionic app by implementing in-app purchases and watch your revenue grow as your users enjoy premium features.
Happy coding!
Table of Contents
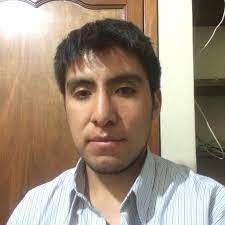
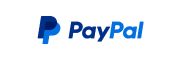