Building Cross-Platform Apps with Ionic: A Complete Tutorial
In the ever-evolving world of mobile app development, cross-platform solutions have gained significant popularity. They allow developers to create applications that run seamlessly on multiple platforms with a single codebase, saving time and effort. One such framework that has gained immense recognition is Ionic. In this tutorial, we will delve into the world of Ionic and learn how to build powerful cross-platform apps. We’ll explore its benefits, architecture, and provide you with a comprehensive step-by-step guide.
1. Understanding Cross-Platform Development
Cross-platform development allows developers to write code once and deploy it on multiple platforms, such as iOS, Android, and the web. This approach saves time, reduces development efforts, and ensures consistent user experiences across platforms.
1.1 Advantages of Cross-Platform Apps
Cross-platform apps offer several advantages, including:
- Code Reusability: Developers can write a single codebase that can be shared across multiple platforms, reducing development time and effort.
- Cost-Effectiveness: Building a cross-platform app is more cost-effective than developing separate apps for each platform.
- Faster Time to Market: With cross-platform development, apps can be developed and deployed faster, giving businesses a competitive edge.
- Consistent User Experience: Cross-platform frameworks, like Ionic, provide UI components that are designed to look and feel native on each platform.
- Simplified Maintenance: Maintaining a single codebase simplifies updates and bug fixes, as changes are propagated to all platforms.
1.2 Introduction to Ionic
Ionic is an open-source framework that allows developers to build cross-platform mobile applications using web technologies like HTML, CSS, and JavaScript. It utilizes popular web technologies, such as Angular or React, to create powerful and performant apps. Ionic provides a wide range of UI components and tools for building native-like user interfaces and accessing device features.
2. Getting Started with Ionic
Before diving into Ionic development, we need to set up our development environment.
2.1 Installing Ionic CLI
To begin, make sure you have Node.js installed on your machine. Then, open a terminal and install the Ionic CLI globally by running the following command:
bash npm install -g @ionic/cli
This command installs the Ionic CLI, which provides a set of command-line tools for creating and managing Ionic projects.
2.2 Creating a New Ionic Project
Now, let’s create a new Ionic project. In the terminal, navigate to the directory where you want to create your project and run the following command:
sql ionic start myApp blank
This command creates a new Ionic project named “myApp” using the “blank” template. You can choose from various templates like “tabs,” “sidemenu,” or “blank,” depending on your project requirements.
2.3 Ionic Project Structure
Once the project is created, you’ll see a folder structure containing various files and directories. Here’s an overview of the important files and directories:
- src: This directory contains the source code of your Ionic app, including HTML templates, JavaScript files, and stylesheets.
- www: This directory holds the compiled output of your app, ready to be served or deployed.
- ionic.config.json: This file contains configuration settings for your Ionic project.
- package.json: This file lists the dependencies and scripts for your project, allowing you to manage and install additional packages.
3. Building User Interfaces with Ionic
Ionic provides a wide range of UI components and tools for building visually appealing user interfaces.
3.1 Ionic Components and Styling
Ionic offers a comprehensive set of pre-designed UI components that look and feel native on different platforms. These components include buttons, cards, lists, forms, and more. You can leverage these components to create interactive and responsive user interfaces.
Let’s take a look at an example of using an Ionic button component:
html <ion-button> Click Me </ion-button>
In addition to components, Ionic also provides a powerful theming system that allows you to customize the appearance of your app. You can easily change colors, fonts, and other visual aspects to match your app’s branding.
3.2 Layouts and Navigation
Ionic offers various layout options to structure your app’s content, such as grids, flexboxes, and predefined templates. These layouts help in creating responsive designs that adapt to different screen sizes and orientations.
To enable navigation between different pages or screens, Ionic provides a routing system. You can define routes for each page and use navigation methods to navigate between them. Here’s an example of setting up routing in an Ionic app:
typescript const routes: Routes = [ { path: '', redirectTo: 'home', pathMatch: 'full' }, { path: 'home', component: HomePage }, { path: 'about', component: AboutPage }, // ... ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
3.3 Theming and Customization
Ionic’s theming capabilities allow you to customize the appearance of your app by changing variables like colors, typography, and spacing. You can override default styles or create your own themes to match your app’s branding.
Ionic themes are defined using CSS variables, which can be easily modified in your app’s global CSS file. Here’s an example of customizing the primary color:
css :root { --ion-color-primary: #ff4081; }
By modifying these variables, you can quickly create unique and visually appealing user interfaces.
4. Working with Ionic Services
Ionic integrates with various services and tools to provide additional functionality to your app.
4.1 Integrating Cordova Plugins
Cordova plugins allow you to access native device features, such as the camera, geolocation, or device sensors, in your Ionic app. Ionic seamlessly integrates with Cordova, making it easy to add these plugins to your project.
To install a Cordova plugin, use the Cordova CLI or Ionic CLI. Here’s an example of installing the Camera plugin:
java ionic cordova plugin add cordova-plugin-camera npm install @ionic-native/camera
After installation, you can import and use the plugin in your code. Here’s an example of using the Camera plugin to capture a photo:
typescript import { Camera } from '@ionic-native/camera/ngx'; constructor(private camera: Camera) {} takePhoto() { this.camera.getPicture().then(imageData => { // Process the image data }).catch(error => { // Handle the error }); }
4.2 Using Capacitor for Native Functionality
Capacitor is another native runtime for building cross-platform apps with Ionic. It offers a modern alternative to Cordova, providing improved performance and a simpler plugin development experience.
To add a Capacitor plugin, use the following command:
bash npm install @capacitor/{plugin-name} npx cap sync
After installing the plugin, you can import and use it in your code. Capacitor plugins provide TypeScript APIs that make it easy to work with native functionality.
4.3 Accessing Device Features
With Ionic and its integration with Cordova or Capacitor, you can access various device features, including the camera, contacts, geolocation, notifications, and more. By leveraging these features, you can create powerful and interactive mobile experiences.
5. Managing Data with Ionic
To create robust applications, you’ll need to handle data storage, integrate with APIs, and provide offline capabilities.
5.1 Data Storage Options
Ionic offers several options for storing data in your app. You can use local storage, SQLite databases, or even leverage cloud-based storage services like Firebase.
For example, to use local storage in your Ionic app, you can use the Ionic Storage module. Here’s an example of saving and retrieving data from local storage:
typescript import { Storage } from '@ionic/storage-angular'; constructor(private storage: Storage) {} saveData(key: string, value: any) { this.storage.set(key, value); } getData(key: string): Promise<any> { return this.storage.get(key); }
5.2 Working with APIs
To fetch data from external APIs, you can use Angular’s HttpClient module in conjunction with Ionic’s HTTP client wrapper. This allows you to make HTTP requests and process the responses in your Ionic app.
Here’s an example of making an API call using Ionic’s HTTP client:
typescript import { HttpClient } from '@angular/common/http'; constructor(private http: HttpClient) {} fetchData() { const apiUrl = 'https://api.example.com/data'; this.http.get(apiUrl).subscribe(data => { // Process the received data }, error => { // Handle the error }); }
5.3 Offline Capabilities
Ionic offers tools and plugins to enable offline capabilities in your app. By caching data locally, you can provide users with access to essential functionality even when they’re offline.
Ionic’s Offline Storage module allows you to cache API responses and serve them when the device is offline. You can also leverage tools like Service Workers to cache static assets and enable offline access.
6. Testing and Debugging Ionic Apps
Testing and debugging are crucial steps in app development. Ionic provides various tools and techniques to test and debug your app effectively.
6.1 Running the App in the Browser
During development, you can run your Ionic app in the browser to quickly iterate and test your code. The Ionic CLI provides a command to serve your app locally, allowing you to preview it in the browser:
ionic serve
This command starts a local development server and opens your app in the browser. Any changes you make to your code will trigger an automatic reload, making the development process smooth and efficient.
6.2 Emulating and Debugging on Devices
To test your app on physical devices or emulators, you can use tools like the Android Emulator or Xcode’s iOS Simulator. Ionic CLI provides commands to build and run your app on various platforms.
For example, to build and emulate your app on an Android device or emulator, you can use the following commands:
arduino ionic build ionic capacitor build android ionic capacitor run android --livereload
These commands generate the necessary build files and launch your app on the connected device or emulator, allowing you to test and debug the app in a native environment.
6.3 Testing and Debugging Tools
Ionic apps can be tested using popular testing frameworks like Jasmine and Karma. Ionic provides a testing harness and utilities that simplify the testing process. You can write unit tests for your components, services, and other parts of your app.
For debugging purposes, Ionic supports tools like Chrome DevTools, which can be used to inspect and debug Ionic apps running in the browser or on devices. You can leverage these tools to identify and fix issues during development.
7. Publishing and Distributing Ionic Apps
Once you’ve built and tested your Ionic app, it’s time to publish and distribute it to your users.
7.1 Preparing for Release
Before publishing, make sure to configure your app’s metadata, such as the app name, description, icon, and version number. Additionally, ensure your app complies with platform-specific guidelines and policies.
For example, for Android, you’ll need to generate a signing key and sign your app’s APK file. On iOS, you’ll need to create a distribution certificate and provisioning profile.
7.2 Generating App Builds
To generate app builds for different platforms, Ionic CLI provides specific commands. For example, to build an Android APK, use the following command:
css ionic capacitor build android --prod
This command generates an optimized production build of your app for the Android platform.
7.3 Publishing to App Stores
To publish your app to the respective app stores, you need to follow the guidelines and procedures provided by Apple’s App Store and Google Play Store.
For iOS, you’ll need to create an App Store Connect account, submit your app for review, and adhere to Apple’s submission guidelines.
For Android, you’ll need a Google Play Developer account. After creating the account, you can create a new app listing, upload the APK file, provide the required details, and submit it for review.
8. Ionic Best Practices and Performance Optimization
To ensure the quality and performance of your Ionic app, it’s important to follow best practices and optimize your code.
8.1 Code Organization and Modularity
Maintaining a well-organized codebase is essential for long-term project maintainability. Follow recommended folder structures and code organization practices, such as separating components, services, and modules.
You can leverage Angular’s modularity concepts like lazy loading to optimize your app’s performance. Lazy loading loads modules and components on-demand, reducing the initial loading time of your app.
8.2 Performance Optimization Techniques
To optimize the performance of your Ionic app, consider the following techniques:
- Use lazy loading to load modules and components on-demand.
- Optimize image assets by compressing them and using lazy loading techniques.
- Minimize the usage of expensive operations in critical sections of your code.
- Implement efficient data binding and change detection strategies.
- Reduce HTTP requests by bundling and compressing your assets.
8.3 Maintaining Code Quality
Maintaining code quality is crucial for long-term project success. Use tools like TypeScript, linters, and automated testing to enforce coding standards and catch potential issues early on.
You can use tools like ESLint or TSLint to perform static code analysis and identify potential errors, style violations, or code smells. Additionally, write unit tests to ensure the correctness and stability of your codebase.
Conclusion
In this tutorial, we explored the world of Ionic and learned how to build cross-platform apps using this powerful framework. We covered the benefits of cross-platform development, the fundamentals of Ionic, and walked through the process of creating an Ionic app from scratch. We also discussed UI development, working with services and data, testing, debugging, and publishing your app to app stores.
Ionic provides a flexible and robust platform for building cross-platform apps with web technologies. By following the steps and best practices outlined in this tutorial, you are well-equipped to embark on your journey of building powerful, visually appealing, and performant cross-platform apps with Ionic.
Table of Contents
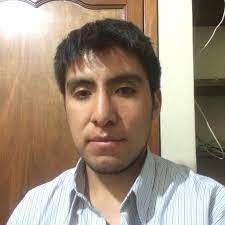
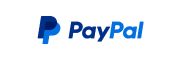