Introduction to Ionic Capacitor: Extending Native Functionality
As the demand for cross-platform mobile app development grows, developers constantly seek efficient ways to bridge the gap between web technologies and native device features. Ionic Capacitor is a powerful solution that enables developers to extend the functionality of their hybrid apps, offering seamless access to native features. In this blog, we will explore what Ionic Capacitor is, how it complements other frameworks like Ionic Framework, and how to leverage its capabilities to create exceptional mobile applications.
1. What is Ionic Capacitor?
Ionic Capacitor is an open-source, cross-platform runtime that allows developers to build web-based applications using technologies like HTML, CSS, and JavaScript, and then deploy them as native apps. Unlike other solutions that rely on a WebView wrapper, Capacitor achieves this by providing a set of APIs to access native features of the device. It acts as a bridge, making it possible to call native functionality through web code.
Originally created by the Ionic team, Capacitor aims to address some limitations of Cordova (a similar framework). It offers improved performance, easier configuration, and a plugin system that simplifies access to native device features. Capacitor is designed to work seamlessly with any frontend framework, but it integrates particularly well with Ionic Framework, taking hybrid app development to a whole new level.
2. Why Choose Ionic Capacitor?
When it comes to hybrid app development, using Ionic Capacitor offers several advantages:
2.1. Native Functionality
Ionic Capacitor allows you to access native device features like camera, geolocation, file system, and more through well-documented JavaScript APIs. This native access enhances the user experience, enabling your app to perform functions that are typically reserved for native apps.
2.2. Cross-Platform Compatibility
With Ionic Capacitor, you can create a single codebase for your app and deploy it across multiple platforms, such as iOS, Android, Electron, and the web. This significantly reduces development time and effort, making maintenance and updates much more manageable.
2.3. Optimal Performance
Capacitor’s architecture ensures that your app runs smoothly with minimal performance overhead. By using a native bridge, Capacitor eliminates the performance issues often associated with traditional WebView-based solutions.
2.4. Simplified Plugin System
Capacitor’s plugin system streamlines the process of adding native features to your app. It comes with a range of core plugins, and you can also create custom plugins if needed, allowing you to tailor your app’s capabilities to your specific requirements.
2.5. Community and Support
As an actively developed open-source project, Capacitor benefits from a thriving community and continuous improvements. You can rely on comprehensive documentation, tutorials, and community support to assist you in your app development journey.
3. Ionic Capacitor vs. Cordova: Key Differences
Ionic Capacitor shares similarities with Apache Cordova in their mission to enable hybrid app development, but there are some critical differences between the two:
3.1. Plugin Architecture
Cordova plugins are typically wrapped WebView components that mediate communication between web code and native code. In contrast, Capacitor plugins are pure native code modules with a JavaScript API, offering better performance and a more streamlined development process.
3.2. WebView vs. Native Bridge
Cordova uses a WebView to render app content, which can lead to performance issues and limitations in accessing native features. Capacitor, on the other hand, employs a native bridge for accessing native functionality, ensuring better performance and a more native-like experience.
3.3. Configuration and Project Structure
Capacitor simplifies project configuration, making it easier to integrate with different frontend frameworks. It uses a standardized project structure, making it more approachable for developers with varying levels of expertise. Cordova, on the other hand, requires additional configuration and has a steeper learning curve.
3.4. UI and User Experience
As Capacitor enables direct access to native features, it allows for a more seamless user experience with a native look and feel. Cordova’s WebView-based approach may sometimes lead to inconsistencies in UI and user experience across different platforms.
4. Integrating Ionic Capacitor with an Ionic App
Now that we understand the benefits and differences between Capacitor and Cordova, let’s see how we can integrate Ionic Capacitor with an Ionic app. The following steps will guide you through the process:
Step 1: Set up an Ionic Project
To begin, ensure you have Node.js and npm (Node Package Manager) installed on your system. Then, create a new Ionic project by running the following command in your terminal or command prompt:
bash $ npm install -g @ionic/cli $ ionic start my-capacitor-app blank $ cd my-capacitor-app
This sets up a new Ionic project named “my-capacitor-app” with a blank template.
Step 2: Install Capacitor
Next, install Capacitor into your Ionic project by running the following command:
bash $ npm install @capacitor/core @capacitor/cli
This will add Capacitor as a dependency to your project.
Step 3: Sync Capacitor with Your Project
After installing Capacitor, you need to initialize it and synchronize it with your project. Use the following commands:
bash $ npx cap init [app-name] [app-id] $ npx cap add [platform]
Replace [app-name] and [app-id] with your app’s name and unique identifier. For [platform], choose either “android” or “ios” to add the respective platforms.
Now, your Ionic project is integrated with Capacitor, and you can start using Capacitor’s native functionality.
5. Understanding Capacitor Plugins
One of the key strengths of Ionic Capacitor is its plugin architecture, which provides an easy way to access native device features. Capacitor offers a range of core plugins that cover common functionalities like camera, geolocation, and storage. These plugins can be easily integrated into your project, expanding your app’s capabilities. Additionally, you have the flexibility to create your custom plugins when necessary. Let’s explore some of the core plugins provided by Capacitor:
5.1 Camera Plugin
The Camera plugin allows you to capture photos and videos using the device’s camera. It provides a simple API to take photos, record videos, and access the photo library. Here’s an example of how to use the Camera plugin:
typescript import { Plugins, CameraResultType } from '@capacitor/core'; async function takePhoto() { const { Camera } = Plugins; const image = await Camera.getPhoto({ quality: 90, allowEditing: true, resultType: CameraResultType.Uri }); // Do something with the image URI }
In this example, the getPhoto method is used to capture a photo, and the result is returned as a URI that can be used to display the image.
5.2 Geolocation Plugin
The Geolocation plugin enables you to retrieve the device’s current geolocation coordinates. It provides methods to request the user’s location and listen for location updates. Here’s a code sample for using the Geolocation plugin:
typescript import { Plugins } from '@capacitor/core'; async function getCurrentLocation() { const { Geolocation } = Plugins; const position = await Geolocation.getCurrentPosition(); const latitude = position.coords.latitude; const longitude = position.coords.longitude; // Do something with the coordinates }
By calling the getCurrentPosition method, you can retrieve the user’s current latitude and longitude.
5.3 Storage Plugin
The Storage plugin allows you to store data locally on the device. It provides methods to set, get, and remove data, making it ideal for managing app preferences or caching data. Here’s an example of using the Storage plugin:
typescript import { Plugins } from '@capacitor/core'; async function storeData(key: string, value: any) { const { Storage } = Plugins; await Storage.set({ key: key, value: JSON.stringify(value) }); } async function retrieveData(key: string) { const { Storage } = Plugins; const item = await Storage.get({ key: key }); return JSON.parse(item.value); }
In this code snippet, the storeData function stores data by converting it to a JSON string, and the retrieveData function retrieves and parses the stored data.
6. Using Capacitor Core Plugins
Now that you have an understanding of some core Capacitor plugins, let’s explore how they can be utilized to enhance your app’s functionality.
6.1 Camera Plugin: Adding Image Upload Feature
Adding image upload functionality to your app is a common requirement. By leveraging the Camera plugin, you can allow users to capture or select images from their device’s gallery. Here’s a step-by-step guide:
1. Install the Camera Plugin: If you haven’t already installed the Camera plugin, do it by running the following command:
bash $ npm install @capacitor/camera
2. Import and Initialize the Camera Plugin: Import the Camera plugin in your TypeScript file and initialize it within your function.
typescript import { Plugins, CameraResultType } from '@capacitor/core'; async function takePhoto() { const { Camera } = Plugins; const image = await Camera.getPhoto({ quality: 90, allowEditing: true, resultType: CameraResultType.Uri }); // Do something with the image URI (e.g., display the image or upload it to a server) }
3. HTML and UI Integration: Create a button or an interface that triggers the takePhoto function.
html <ion-button (click)="takePhoto()">Take a Photo</ion-button>
4. Displaying the Captured Image: You can display the captured image by binding the image URI to an <img> tag in your HTML.
html <img [src]="image" *ngIf="image">
By implementing these steps, you have successfully added the image upload feature to your app using the Camera plugin. Users can now take photos or select images from their device and view them directly within the app.
6.2 Geolocation Plugin: Enhancing User Experience with Location-Based Features
Integrating the Geolocation plugin can enhance your app with location-based features, such as displaying nearby points of interest, providing directions, or geotagging user-generated content. Here’s how to use the Geolocation plugin:
1. Install the Geolocation Plugin: If you haven’t already installed the Geolocation plugin, do it by running the following command:
bash $ npm install @capacitor/geolocation
2. Import and Retrieve the User’s Location: Import the Geolocation plugin and use the getCurrentPosition method to retrieve the user’s current location.
typescript import { Plugins } from '@capacitor/core'; async function getCurrentLocation() { const { Geolocation } = Plugins; const position = await Geolocation.getCurrentPosition(); const latitude = position.coords.latitude; const longitude = position.coords.longitude; // Do something with the coordinates (e.g., display a map or find nearby points of interest) }
3. HTML and UI Integration: Trigger the getCurrentLocation function when appropriate (e.g., on a button click) and display the results to the user.
html <ion-button (click)="getCurrentLocation()">Get Current Location</ion-button>
- Use the Coordinates: You can use the retrieved latitude and longitude to perform various location-based tasks, such as displaying a map centered on the user’s location or finding nearby places using APIs like Google Places or OpenStreetMap.
By incorporating these steps, you have successfully added location-based features to your app using the Geolocation plugin. Users can now access their current location, and you can use this data to create personalized and relevant experiences.
6.3 Storage Plugin: Saving User Preferences
The Storage plugin is handy for managing app preferences and storing small amounts of data locally. Let’s use it to save and retrieve user preferences such as theme settings:
1. Install the Storage Plugin: If you haven’t already installed the Storage plugin, do it by running the following command:
bash $ npm install @capacitor/storage
2. Import and Set User Preferences: Import the Storage plugin and use the set method to store the user’s theme preference.
typescript import { Plugins } from '@capacitor/core'; async function setThemePreference(theme: string) { const { Storage } = Plugins; await Storage.set({ key: 'theme', value: theme }); }
3. HTML and UI Integration: Provide users with a way to change their theme preference (e.g., through a dropdown or toggle).
html <ion-select (ionChange)="setThemePreference($event.target.value)"> <ion-select-option value="light">Light Theme</ion-select-option> <ion-select-option value="dark">Dark Theme</ion-select-option> </ion-select>
4. Retrieve and Apply the Stored Preference: When the app starts, retrieve the stored theme preference and apply it to the user interface.
typescript import { Plugins } from '@capacitor/core'; async function applyStoredTheme() { const { Storage } = Plugins; const themePreference = await Storage.get({ key: 'theme' }); const selectedTheme = themePreference.value || 'light'; document.body.classList.add(selectedTheme); }
By following these steps, you have added a simple user preference feature to your app using the Storage plugin. Users can now choose their preferred theme, and the app will remember and apply their selection.
7. Creating Custom Capacitor Plugins
While Capacitor comes with a rich set of core plugins, there might be scenarios where you need custom functionality for your app. In such cases, you can create your own Capacitor plugins. Custom plugins allow you to access native device features that are not available in the core set. Creating a custom plugin involves a few key steps:
7.1 Plugin Structure
A Capacitor plugin consists of two parts: a native part written in the platform’s native language (Java/Kotlin for Android, Objective-C/Swift for iOS) and a web part that exposes the plugin API to the web code. The structure typically looks like this:
plaintext |- my-custom-plugin/ |- src/ |- android/ // Android native implementation |- ios/ // iOS native implementation |- web/ // Web implementation (TypeScript) |- package.json // Plugin package metadata |- capacitor.config.json // Plugin configuration
7.2 Bridging Native Code
In the native part of the plugin, you’ll implement the functionality using the native language and expose it as methods that can be called from the web code. For instance, if you want to create a custom plugin to handle barcode scanning, you’ll write native code that interfaces with the device’s camera and barcode scanning capabilities.
7.3 TypeScript API
In the web part of the plugin, you’ll create a TypeScript API that represents the native methods. This API will serve as the interface through which your web code interacts with the native functionality. Here’s a simplified example of a barcode scanner plugin:
typescript // src/web/barcode-scanner.ts import { Plugins } from '@capacitor/core'; export interface BarcodeScannerPlugin { scan(): Promise<ScanResult>; } export interface ScanResult { text: string; } const BarcodeScanner = Plugins.BarcodeScanner as BarcodeScannerPlugin; export default BarcodeScanner;
7.4 Testing the Custom Plugin
To test your custom plugin, you’ll need to build it and add it to your Ionic project. Here’s how:
1. Build the plugin:
bash $ npm run build
2. Link the plugin to your Ionic project:
bash $ npm link $ cd my-capacitor-app $ npm link my-custom-plugin
3. Use the custom plugin in your Ionic project:
typescript import BarcodeScanner from 'my-custom-plugin'; async function scanBarcode() { try { const result = await BarcodeScanner.scan(); console.log('Scanned barcode:', result.text); } catch (error) { console.error('Barcode scanning failed:', error); } }
By creating a custom Capacitor plugin, you can extend your app’s capabilities beyond what the core plugins offer. This flexibility allows you to cater to unique use cases and create more sophisticated apps.
8. Capacitor Community: A Growing Ecosystem
The power of any development framework lies not only in its core capabilities but also in the strength of its community. Capacitor has gained substantial traction, and its community is continuously growing. The community-driven development approach ensures that Capacitor stays up-to-date with the latest advancements in mobile development and native features. Developers can benefit from an active community forum, extensive documentation, and a rich collection of community-contributed plugins.
Conclusion
Ionic Capacitor is a game-changer for hybrid app development, offering a bridge between web technologies and native device features. By leveraging Capacitor, developers can create cross-platform apps with enhanced native functionality, optimal performance, and a streamlined development process. The combination of core plugins and the flexibility to create custom plugins empowers developers to craft exceptional mobile experiences. As the Capacitor community continues to grow, this innovative runtime is set to be a top choice for cross-platform app development for years to come.
With Ionic Capacitor at your disposal, you can take your hybrid app development to new heights and create powerful and feature-rich applications for a wide range of devices and platforms. So, why not embrace the power of Capacitor and extend your app’s potential today? Happy coding!
Table of Contents
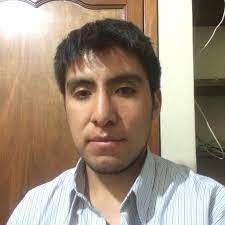
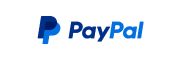