Ionic and Firebase Integration: Building Real-time Apps
In today’s fast-paced world, real-time applications have become the norm. Users expect instant updates and live interactions within the apps they use. To meet this demand, developers need robust tools and technologies that can handle real-time data synchronization effortlessly. In this blog post, we will explore the integration of Ionic, a popular framework for building cross-platform mobile applications, with Firebase, a powerful real-time backend platform provided by Google. We will dive into the steps, benefits, and code samples to help you seamlessly integrate Ionic and Firebase and build real-time apps.
What is Ionic?
Ionic is a popular open-source framework for building cross-platform mobile applications. It utilizes web technologies such as HTML, CSS, and JavaScript to develop mobile apps that can run on multiple platforms, including iOS, Android, and the web. Ionic provides a rich set of UI components, plugins, and tools to simplify the development process and deliver high-quality mobile apps.
What is Firebase?
Firebase is a comprehensive development platform that provides a wide range of tools and services for building scalable and real-time applications. It offers features such as authentication, real-time database, cloud storage, cloud functions, and more. Firebase’s real-time database and Cloud Firestore enable developers to synchronize data across devices in real-time, making it an ideal choice for building real-time applications.
Why integrate Ionic with Firebase?
Integrating Ionic with Firebase offers several benefits for building real-time apps:
- Real-time Data Sync: Firebase’s real-time database and Cloud Firestore provide seamless data synchronization across devices, allowing users to experience real-time updates within the app.
- Authentication: Firebase Authentication offers easy-to-use APIs for user authentication, including social logins, email/password authentication, and more. This enables developers to add secure user authentication to their Ionic apps effortlessly.
- Cloud Functions: Firebase allows developers to extend app functionality with serverless backend logic using Cloud Functions. This enables performing complex operations and integrating with external services without the need for managing server infrastructure.
- Scalability: Firebase handles the scalability aspect, ensuring that your app can handle a large number of concurrent users and real-time updates without compromising performance.
Prerequisites:
Before we begin, make sure you have the following:
Node.js and npm installed on your machine
Ionic CLI installed globally
A Google account to create a Firebase project
Step 1: Set up an Ionic project:
To get started, let’s create a new Ionic project. Open your terminal or command prompt and run the following commands:
bash # Install Ionic CLI globally (if not already installed) npm install -g @ionic/cli # Create a new Ionic project ionic start myApp blank cd myApp
Step 2: Create a Firebase project:
To integrate Firebase with your Ionic app, you need to create a Firebase project. Follow these steps:
Go to the Firebase Console (https://console.firebase.google.com/) and sign in with your Google account.
Click on “Add project” and provide a name for your project.
Follow the on-screen instructions to set up your project.
Once the project is created, you will be redirected to the project dashboard.
Step 3: Add Firebase to your Ionic project:
To add Firebase to your Ionic project, you need to install the Firebase and AngularFire packages. Run the following commands in your project directory:
bash # Install Firebase and AngularFire npm install firebase @angular/fire
Open the src/environments/environment.ts file and add your Firebase configuration details. You can find these details in the Firebase project settings under the “General” tab. Your environment.ts file should look like this:
typescript export const environment = { production: false, firebase: { apiKey: 'YOUR_API_KEY', authDomain: 'YOUR_AUTH_DOMAIN', databaseURL: 'YOUR_DATABASE_URL', projectId: 'YOUR_PROJECT_ID', storageBucket: 'YOUR_STORAGE_BUCKET', messagingSenderId: 'YOUR_MESSAGING_SENDER_ID', appId: 'YOUR_APP_ID', }, };
Step 4: Firebase Authentication:
Firebase Authentication provides secure user authentication for your Ionic app. Let’s explore how to enable authentication, allow user registration, and implement user login functionality.
Enable Authentication:
Go to the Firebase console and navigate to “Authentication.” In the “Sign-in method” tab, enable the desired sign-in providers (e.g., Email/Password, Google, etc.) and configure them accordingly.
User Registration:
In your Ionic app, create a registration form and implement the registration logic using the AngularFire library. Here’s an example of a registration component:
typescript import { Component } from '@angular/core'; import { AngularFireAuth } from '@angular/fire/auth'; @Component({ selector: 'app-registration', templateUrl: 'registration.component.html', styleUrls: ['registration.component.scss'], }) export class RegistrationComponent { email: string; password: string; constructor(private afAuth: AngularFireAuth) {} register() { this.afAuth.createUserWithEmailAndPassword(this.email, this.password) .then((userCredential) => { // Registration successful }) .catch((error) => { // Registration failed }); } }
User Login:
Implementing user login functionality is similar to the registration process. Create a login component and use the signInWithEmailAndPassword method to authenticate the user. Here’s an example:
typescript import { Component } from '@angular/core'; import { AngularFireAuth } from '@angular/fire/auth'; @Component({ selector: 'app-login', templateUrl: 'login.component.html', styleUrls: ['login.component.scss'], }) export class LoginComponent { email: string; password: string; constructor(private afAuth: AngularFireAuth) {} login() { this.afAuth.signInWithEmailAndPassword(this.email, this.password) .then((userCredential) => { // Login successful }) .catch((error) => { // Login failed }); } }
Step 5: Real-time Database:
Firebase’s real-time database allows you to store and sync data in real-time. Let’s explore how to set up the real-time database, read and write data, and enable real-time data synchronization.
Set up Real-time Database:
Go to the Firebase console, navigate to “Database,” and click on “Create Database.” Choose “Start in test mode” and click “Next.” Select the desired location for your database and click “Done.”
Read and Write Data:
To read and write data in the real-time database, import the AngularFireDatabase and use its methods. Here’s an example of reading and writing data:
typescript import { Component } from '@angular/core'; import { AngularFireDatabase } from '@angular/fire/database'; @Component({ selector: 'app-data', templateUrl: 'data.component.html', styleUrls: ['data.component.scss'], }) export class DataComponent { constructor(private db: AngularFireDatabase) {} getData() { this.db.object('path/to/data').valueChanges().subscribe((data) => { // Data retrieved }); } setData() { this.db.object('path/to/data').set({ key: 'value' }) .then(() => { // Data saved successfully }) .catch((error) => { // Error occurred while saving data }); } }
Real-time Data Synchronization:
Real-time data synchronization allows your app to receive updates automatically whenever data changes. Use the valueChanges() method to listen for changes in real-time. Here’s an example:
typescript import { Component } from '@angular/core'; import { AngularFireDatabase } from '@angular/fire/database'; @Component({ selector: 'app-sync', templateUrl: 'sync.component.html', styleUrls: ['sync.component.scss'], }) export class SyncComponent { constructor(private db: AngularFireDatabase) {} syncData() { this.db.object('path/to/data').valueChanges().subscribe((data) => { // Data synchronized in real-time }); } }
Step 6: Cloud Firestore:
Cloud Firestore is Firebase’s flexible and scalable NoSQL document database. Let’s explore how to set up Cloud Firestore, add, retrieve, and update data.
Set up Cloud Firestore:
Go to the Firebase console, navigate to “Firestore Database,” and click on “Create Database.” Choose “Start in production mode” and click “Next.” Select the desired location for your database and click “Enable.”
Add, Retrieve, and Update Data:
To interact with Cloud Firestore, import the AngularFirestore and use its methods. Here’s an example:
typescript import { Component } from '@angular/core'; import { AngularFirestore } from '@angular/fire/firestore'; @Component({ selector: 'app-firestore', templateUrl: 'firestore.component.html', styleUrls: ['firestore.component.scss'], }) export class FirestoreComponent { constructor(private firestore: AngularFirestore) {} addData() { this.firestore.collection('collectionName').add({ key: 'value' }) .then(() => { // Data added successfully }) .catch((error) => { // Error occurred while adding data }); } getData() { this.firestore.collection('collectionName').valueChanges().subscribe((data) => { // Data retrieved }); } updateData() { this.firestore.collection('collectionName').doc('documentId').update({ key: 'value' }) .then(() => { // Data updated successfully }) .catch((error) => { // Error occurred while updating data }); } }
Conclusion:
Integrating Ionic with Firebase opens up a world of possibilities for building real-time apps. In this blog post, we explored the steps to integrate Ionic with Firebase, including setting up an Ionic project, creating a Firebase project, adding Firebase to the Ionic project, and implementing features such as authentication, real-time database, and Cloud Firestore. By combining the power of Ionic’s cross-platform development capabilities with Firebase’s real-time data synchronization, you can build responsive and interactive mobile apps that keep users engaged. Start integrating Ionic with Firebase today and unlock the potential of real-time app development.
Table of Contents
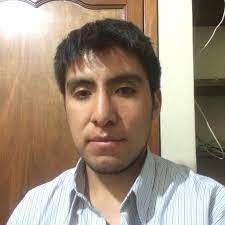
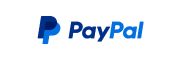