Ionic and Gaming: Creating Immersive Mobile Game Experiences
Mobile gaming has become an integral part of our daily lives, providing entertainment, relaxation, and challenges at our fingertips. As the demand for engaging mobile games continues to grow, developers are constantly seeking versatile and efficient platforms to create immersive gaming experiences. One such platform that may surprise you is Ionic.
Table of Contents
In this blog, we will delve into the world of Ionic and explore how it can be used to craft captivating mobile games. We’ll cover everything from the basics of setting up your development environment to more advanced techniques for building feature-rich games. So, fasten your seatbelts, and let’s embark on this exciting journey of Ionic-powered gaming!
1. What is Ionic?
Before we dive into game development, let’s start with the fundamentals. Ionic is a popular open-source framework for building cross-platform mobile applications. It’s based on web technologies like HTML, CSS, and JavaScript, making it an excellent choice for web developers looking to create mobile apps without diving into native development for multiple platforms.
Ionic provides a rich set of UI components, a robust development environment, and a vibrant community. While it’s commonly used for creating mobile apps, it’s also a powerful tool for game development when used creatively.
2. Setting Up Your Ionic Development Environment
2.1. Installing Node.js and npm
To begin your Ionic-powered game development journey, you’ll need Node.js and npm (Node Package Manager) installed on your machine. These tools are essential for managing dependencies and running various development tasks.
You can download Node.js from the official website (https://nodejs.org/) and npm will be included with it. Once installed, open your terminal or command prompt and run the following commands to verify their installation:
bash node -v npm -v
You should see version numbers displayed, confirming that Node.js and npm are correctly installed.
2.2. Installing Ionic CLI
With Node.js and npm in place, it’s time to install the Ionic CLI (Command Line Interface), which will be your primary tool for managing Ionic projects. Open your terminal or command prompt and run the following command:
bash npm install -g @ionic/cli
This command installs the Ionic CLI globally on your system, allowing you to create and manage Ionic projects from anywhere on your machine.
3. Creating Your First Ionic Project
Now that you have the necessary tools installed, let’s create your first Ionic project. Navigate to the directory where you’d like to create your project and run the following command:
bash ionic start MyGame blank
Replace MyGame with the desired name of your game project. This command initializes a new Ionic project using the “blank” template.
4. Running Your Ionic Project
Once your project is created, navigate into its directory using the cd command:
bash cd MyGame
Now, you can run your Ionic project in a web browser by using the following command:
bash ionic serve
This command starts a development server and opens your project in a web browser. You can make changes to your game and see them in real-time as you save your code.
5. Building a Simple Game with Ionic
Now that your development environment is set up, let’s create a simple game using Ionic. We’ll build a classic “Guess the Number” game where the player tries to guess a randomly generated number.
5.1. Project Structure
Before diving into the code, let’s take a look at the project structure. In your Ionic project directory, you’ll find various files and folders. Here’s a brief overview of some of the essential ones:
- src/: This folder contains your game’s source code, including HTML, CSS, and JavaScript files.
- src/index.html: The main HTML file of your game.
- src/app/: This folder contains the Angular components that make up your app.
- src/app/app.module.ts: The main module file where you can import and configure components.
- src/app/app.component.ts: The root component of your app.
5.2. Creating the Game
Let’s start by editing the src/app/app.component.html file to create the game interface. Replace the existing content with the following code:
html <ion-header> <ion-toolbar> <ion-title> Guess the Number </ion-title> </ion-toolbar> </ion-header> <ion-content class="ion-padding"> <h2>Welcome to Guess the Number!</h2> <p>Try to guess the number between 1 and 100.</p> <ion-input type="number" placeholder="Enter your guess"></ion-input> <ion-button expand="full" (click)="checkGuess()">Submit Guess</ion-button> <p>{{ message }}</p> </ion-content>
In this code, we’ve created a simple user interface with a title, instructions, an input field for the player’s guess, a submit button, and a message area to display feedback.
5.3. Adding Game Logic
Now, let’s implement the game logic in src/app/app.component.ts. Replace the content of this file with the following TypeScript code:
typescript import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: 'app.component.html', styleUrls: ['app.component.scss'], }) export class AppComponent { randomNumber: number; playerGuess: number; message: string; constructor() { this.randomNumber = Math.floor(Math.random() * 100) + 1; } checkGuess() { if (this.playerGuess === this.randomNumber) { this.message = 'Congratulations! You guessed the correct number!'; } else if (this.playerGuess < this.randomNumber) { this.message = 'Try a higher number.'; } else { this.message = 'Try a lower number.'; } } }
This code defines the game logic. When the player submits a guess, the checkGuess function compares it to the randomly generated number and provides feedback in the message area.
5.4. Styling the Game
To make our game look more appealing, we can add some CSS styles to src/app/app.component.scss. Here’s some sample styling:
scss ion-content { --background: #f4f4f4; } ion-button { margin-top: 10px; }
These styles set the background color and adjust the button’s spacing.
5.5. Testing the Game
Now that you’ve created the game, save your changes and run the development server using ionic serve. Open your web browser and navigate to http://localhost:8100 to play the game. You can enter guesses, and the game will provide feedback until you guess the correct number.
6. Advanced Techniques for Ionic Game Development
Creating a simple game is a great starting point, but Ionic can be used for more complex and feature-rich mobile games. Here are some advanced techniques and considerations for Ionic game development:
6.1. Using Canvas for Advanced Graphics
To create games with intricate graphics, you can incorporate the HTML5 <canvas> element into your Ionic app. This allows you to draw and manipulate graphics directly on the screen, opening up possibilities for 2D games, animations, and more.
Here’s an example of how to use the <canvas> element in an Ionic app:
html <ion-content class="ion-padding"> <canvas id="gameCanvas" width="400" height="400"></canvas> </ion-content>
You can then use JavaScript to interact with the canvas and create custom game graphics.
6.2. Integrating Game Engines
For more ambitious projects, consider integrating a game engine like Phaser or Three.js into your Ionic app. These engines provide powerful tools for creating 2D and 3D games, respectively. By using them in conjunction with Ionic, you can harness the best of both worlds: Ionic’s cross-platform capabilities and the game engine’s advanced features.
6.3. Handling Game State
As games become more complex, managing game state becomes crucial. Ionic’s component-based architecture can help you organize and manage different game states efficiently. For example, you can create separate Angular components for the game menu, gameplay, and game over screens, allowing for clean transitions between states.
6.4. Performance Optimization
Mobile game performance is a critical factor. Ionic provides tools for optimizing performance, such as lazy loading modules and Ahead-of-Time (AOT) compilation. Additionally, consider profiling and optimizing your game’s code to ensure smooth gameplay on a wide range of devices.
6.5. Monetization Strategies
If you plan to monetize your game, explore options like in-app purchases, ads, or premium versions. Ionic’s flexibility allows you to integrate various monetization strategies seamlessly.
Conclusion
Ionic is not just limited to creating mobile apps; it can also be a fantastic platform for developing mobile games. Whether you’re building a simple guessing game or a complex 2D/3D adventure, Ionic provides the tools and flexibility to bring your game ideas to life. With the right techniques and a dash of creativity, you can craft immersive and engaging mobile game experiences that captivate players worldwide.
So, start exploring Ionic’s game development potential, experiment with different game genres, and watch your gaming ideas come to life on the screens of millions of players.
Remember, the key to creating memorable mobile game experiences is not just the technology you use but also the passion and dedication you put into your game development journey. Happy gaming!
Table of Contents
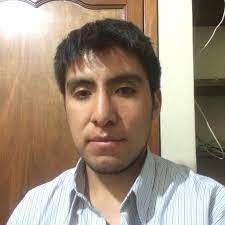
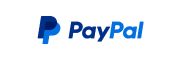