Ionic and Maps: Integrating Geolocation and Mapping Services
In today’s mobile-centric world, location-based services have become an integral part of countless applications. Whether it’s ride-sharing apps, food delivery services, or fitness trackers, integrating geolocation and mapping functionality enhances user experience and opens up new possibilities for businesses and developers.
Ionic, a popular open-source framework, enables developers to build cross-platform mobile applications with ease. In this blog post, we will explore how to leverage Ionic’s geolocation and mapping services to create location-aware apps. We’ll cover the basic setup, integrating geolocation, displaying maps, and adding custom markers to make your apps truly stand out. So, let’s get started!
1. Prerequisites
Before diving into the implementation, make sure you have the following prerequisites:
- Basic knowledge of Ionic and Angular.
- Ionic CLI and Node.js installed on your development machine.
- A code editor of your choice (e.g., Visual Studio Code).
2. Setting Up the Ionic Project
If you haven’t already set up an Ionic project, let’s start by creating a new one. Open your terminal or command prompt and run the following commands:
bash # Install Ionic CLI globally (skip if already installed) npm install -g @ionic/cli # Create a new Ionic project ionic start IonicMapsApp blank --type=angular cd IonicMapsApp
The above commands install the Ionic CLI and create a new Ionic project named “IonicMapsApp” based on the blank template with Angular.
3. Adding Geolocation Plugin
Ionic leverages Cordova plugins to access native device capabilities. To enable geolocation in your app, add the Cordova Geolocation plugin. Run the following command in your project directory:
bash ionic cordova plugin add cordova-plugin-geolocation npm install @ionic-native/geolocation
This adds the Geolocation plugin to your project and installs the Ionic Native wrapper for the plugin, allowing you to use it in your Angular code seamlessly.
4. Implementing Geolocation Functionality
Now that we have the Geolocation plugin integrated into our Ionic project, let’s implement the geolocation functionality. We’ll create a service to encapsulate geolocation-related functions and then use it in our components.
Step 1: Create Geolocation Service
In your project, create a new service using the following command:
bash ionic generate service services/geolocation
This command generates a new service named “GeolocationService” in the “services” folder. Open the generated file (geolocation.service.ts) and update it as follows:
typescript import { Injectable } from '@angular/core'; import { Geolocation, GeolocationOptions, Geoposition } from '@ionic-native/geolocation/ngx'; @Injectable({ providedIn: 'root', }) export class GeolocationService { constructor(private geolocation: Geolocation) {} getCurrentPosition(options?: GeolocationOptions): Promise<Geoposition> { return this.geolocation.getCurrentPosition(options); } }
The service imports the necessary dependencies and provides a getCurrentPosition function that returns a promise, resolving with the current position of the device.
Step 2: Implement Geolocation in a Component
Next, let’s use the GeolocationService we just created in a component to fetch the user’s current location. For demonstration purposes, we’ll display the coordinates in an alert.
Create a new component using the following command:
bash ionic generate component components/location-display
This command generates a new component named “LocationDisplay” in the “components” folder. Open the generated file (location-display.component.ts) and update it as follows:
typescript import { Component, OnInit } from '@angular/core'; import { GeolocationService } from '../../services/geolocation.service'; @Component({ selector: 'app-location-display', templateUrl: './location-display.component.html', styleUrls: ['./location-display.component.scss'], }) export class LocationDisplayComponent implements OnInit { latitude: number; longitude: number; constructor(private geolocationService: GeolocationService) {} ngOnInit() { this.getCurrentLocation(); } getCurrentLocation() { this.geolocationService.getCurrentPosition().then((position) => { this.latitude = position.coords.latitude; this.longitude = position.coords.longitude; alert(`Latitude: ${this.latitude}, Longitude: ${this.longitude}`); }).catch((error) => { console.error('Error getting location', error); }); } }
In this component, we import and use the GeolocationService to obtain the current position. The getCurrentLocation function fetches the coordinates and displays them in an alert. Don’t forget to add this component to your desired page to see the results.
Step 3: Displaying the Location
Now that we have the geolocation functionality implemented, let’s display the user’s location on a map. To achieve this, we’ll use the powerful Google Maps JavaScript API.
5. Adding Google Maps to Your Ionic App
To include Google Maps in your Ionic app, you’ll need to register for an API key from the Google Developer Console. If you don’t have one yet, follow these steps:
- Go to the Google Developer Console (https://console.developers.google.com/).
- Create a new project (if you don’t have one) and enable the Maps JavaScript API.
- Create an API key for your project.
Once you have the API key, add it to your Ionic project by opening the ‘index.html’ file (located in the ‘src’ folder) and adding the following script tag inside the head element:
html <script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places"></script>
Replace YOUR_API_KEY with your actual Google Maps API key.
6. Displaying the Map
Now that we have Google Maps set up, let’s create a new component to display the map. Run the following command to generate the component:
bash ionic generate component components/map-display
This command generates a new component named “MapDisplay” in the “components” folder. Open the generated file (map-display.component.ts) and update it as follows:
typescript import { Component, ViewChild, AfterViewInit } from '@angular/core'; @Component({ selector: 'app-map-display', templateUrl: './map-display.component.html', styleUrls: ['./map-display.component.scss'], }) export class MapDisplayComponent implements AfterViewInit { @ViewChild('mapContainer') mapContainer: ElementRef; map: google.maps.Map; ngAfterViewInit() { this.loadMap(); } loadMap() { const mapOptions: google.maps.MapOptions = { center: { lat: -34.397, lng: 150.644 }, // Set your default map center zoom: 10, // Set the default zoom level }; this.map = new google.maps.Map(this.mapContainer.nativeElement, mapOptions); } }
In this component, we import the necessary dependencies and create the loadMap function to display the map with a default center and zoom level. The template (map-display.component.html) for this component is straightforward:
html <div #mapContainer class="map-container"></div>
We use the Angular ViewChild decorator to access the DOM element where we want to display the map.
7. Integrating Geolocation with Google Maps
With both the geolocation and map components ready, it’s time to bring them together. We’ll combine the current location fetched from the GeolocationService and display it on the map.
Step 1: Add the Map to Your Page
In your desired page, import and include the LocationDisplay and MapDisplay components. For example, open the ‘home.page.html’ file (located in the ‘src/app/pages/home’ folder) and update it as follows:
html <ion-header> <ion-toolbar> <ion-title> Ionic Maps Integration </ion-title> </ion-toolbar> </ion-header> <ion-content> <app-map-display></app-map-display> <app-location-display></app-location-display> </ion-content>
Here, we add both components to the ‘ion-content’ section of the page.
Step 2: Display User’s Location on the Map
Now, let’s modify the MapDisplay component to receive the user’s location from the GeolocationService and display it as a marker on the map.
Open the ‘map-display.component.ts’ file and update it as follows:
typescript import { Component, ViewChild, AfterViewInit } from '@angular/core'; import { GeolocationService } from '../../services/geolocation.service'; @Component({ selector: 'app-map-display', templateUrl: './map-display.component.html', styleUrls: ['./map-display.component.scss'], }) export class MapDisplayComponent implements AfterViewInit { @ViewChild('mapContainer') mapContainer: ElementRef; map: google.maps.Map; userMarker: google.maps.Marker; constructor(private geolocationService: GeolocationService) {} ngAfterViewInit() { this.loadMap(); this.displayUserLocation(); } loadMap() { const mapOptions: google.maps.MapOptions = { center: { lat: -34.397, lng: 150.644 }, // Set your default map center zoom: 10, // Set the default zoom level }; this.map = new google.maps.Map(this.mapContainer.nativeElement, mapOptions); } displayUserLocation() { this.geolocationService.getCurrentPosition().then((position) => { const userLatLng = new google.maps.LatLng( position.coords.latitude, position.coords.longitude ); if (this.userMarker) { this.userMarker.setPosition(userLatLng); } else { this.userMarker = new google.maps.Marker({ position: userLatLng, map: this.map, title: 'You are here!', }); } this.map.setCenter(userLatLng); }).catch((error) => { console.error('Error getting location', error); }); } }
In this updated component, we import the GeolocationService and modify the loadMap function to initialize the map. We also introduce a userMarker variable to hold the user’s marker.
The displayUserLocation function fetches the user’s current position and updates the marker’s position on the map. If the marker doesn’t exist yet, it creates a new one with a custom title.
With these changes, your Ionic app now displays the user’s location on a map!
Conclusion
In this blog post, we explored how to integrate geolocation and mapping services into an Ionic app. We set up the Geolocation plugin, implemented the geolocation functionality using the GeolocationService, and displayed the user’s location on a Google Map using the Maps JavaScript API.
Leveraging Ionic’s powerful framework along with geolocation and mapping services opens up endless possibilities for building location-aware mobile applications. You can now take this knowledge and create location-based features such as geofencing, route tracking, location-based recommendations, and much more.
Remember to keep your users’ privacy in mind and request location permissions responsibly. Additionally, consider optimizing the map display and geolocation functionality to ensure a smooth user experience.
Happy coding and happy mapping!
Table of Contents
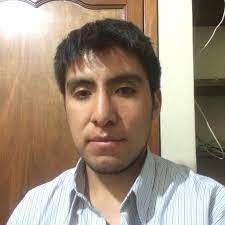
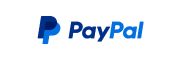