Ionic and GraphQL: Powerful Data Fetching for Mobile Apps
Mobile app development has come a long way, and the demand for feature-rich, dynamic, and responsive applications is constantly growing. To meet these expectations, developers need efficient data-fetching techniques that can seamlessly integrate with their frameworks and deliver a top-notch user experience. This is where Ionic, a popular mobile app development framework, and GraphQL, a powerful query language for APIs, join forces to create an unbeatable combination. In this blog post, we’ll explore how Ionic and GraphQL work together to provide powerful data fetching capabilities for mobile apps, enabling you to build high-performance and flexible applications.
1. Understanding Ionic and GraphQL:
Ionic is a popular open-source framework that allows developers to build cross-platform mobile applications using web technologies such as HTML, CSS, and JavaScript/TypeScript. It provides a wide range of UI components and tools, making it easier to create stunning and interactive mobile apps.
GraphQL, on the other hand, is a query language for APIs that enables clients to request only the data they need, allowing for more efficient data fetching and eliminating the issues of over-fetching and under-fetching data commonly faced with traditional REST APIs.
2. Advantages of Using GraphQL with Ionic:
When combined, Ionic and GraphQL offer several advantages that empower developers to build robust and performant mobile applications:
2.1 Reduced Over-fetching and Under-fetching:
With REST APIs, fetching data often involves retrieving fixed data structures, which may result in over-fetching (receiving more data than required) or under-fetching (not receiving all the required data). GraphQL solves this problem by allowing clients to specify the exact data they need, minimizing unnecessary data transfer and reducing latency.
2.2 Multiple Data Sources:
Mobile apps frequently require data from various sources like databases, third-party APIs, or real-time services. GraphQL’s ability to aggregate data from multiple sources in a single query simplifies data fetching and ensures a seamless user experience.
2.3 Real-time Data Updates:
GraphQL subscriptions enable real-time data updates by establishing a persistent connection between the client and the server. This capability is particularly valuable for building features like live chat, notifications, and collaborative applications.
3. Setting Up Ionic and GraphQL:
Before we can take advantage of the benefits offered by GraphQL in our Ionic app, we need to set up the development environment:
3.1 Creating an Ionic App:
If you haven’t already installed Ionic, start by installing it using npm (Node Package Manager):
bash npm install -g @ionic/cli
Next, create a new Ionic app:
bash ionic start my-ionic-app blank cd my-ionic-app
3.2 Integrating GraphQL in Ionic:
To incorporate GraphQL into our Ionic app, we need to choose a GraphQL server and set up a schema to define the data structure.
For the sake of this blog, let’s assume we already have a GraphQL server running with a schema that defines the data we want to access from our mobile app. We’ll proceed with writing queries to fetch data from the server.
4. Querying Data with GraphQL in Ionic:
With our Ionic app and GraphQL server set up, we can now start fetching data from the server using GraphQL queries.
4.1 Writing GraphQL Queries:
GraphQL queries define the data requirements for the client. We specify the fields we need in the query, and the server responds with the exact data structure we requested.
graphql // Sample GraphQL query to fetch a list of products query GetProducts { products { id name price description } }
4.2 Querying Data in Ionic Components:
In Ionic, we can use popular JavaScript libraries like Apollo Client or Relay to make GraphQL queries. Let’s see how to use Apollo Client to fetch data from our GraphQL server in an Ionic component:
typescript // Import the required dependencies import { ApolloClient, InMemoryCache, gql } from '@apollo/client'; // Create an instance of ApolloClient const client = new ApolloClient({ uri: 'https://example.com/graphql', // Replace with your GraphQL server URL cache: new InMemoryCache(), }); // Define the GraphQL query const GET_PRODUCTS = gql` query GetProducts { products { id name price description } } `; // Use the Apollo Client instance to query data client.query({ query: GET_PRODUCTS, }) .then(result => console.log(result.data.products)) .catch(error => console.error(error));
5. Handling Mutations with GraphQL in Ionic:
In addition to querying data, mobile apps often need to update or mutate data on the server, such as adding new items or modifying existing ones.
5.1 Updating Data on the Server:
GraphQL mutations are used to modify data on the server. We define mutations similar to queries but with specific input fields for data that needs to be modified.
graphql // Sample GraphQL mutation to add a new product mutation AddProduct($input: ProductInput!) { addProduct(input: $input) { id name price } }
5.2 Handling Mutations in Ionic:
Similar to querying data, we can use Apollo Client to handle mutations in our Ionic app:
typescript // Import the required dependencies import { ApolloClient, InMemoryCache, gql } from '@apollo/client'; // ... // Define the GraphQL mutation const ADD_PRODUCT = gql` mutation AddProduct($input: ProductInput!) { addProduct(input: $input) { id name price } } `; // Create a new product object const newProduct = { name: 'New Product', price: 19.99, }; // Use the Apollo Client instance to perform the mutation client.mutate({ mutation: ADD_PRODUCT, variables: { input: newProduct, }, }) .then(result => console.log(result.data.addProduct)) .catch(error => console.error(error));
6. Caching and Offline Support:
Mobile apps often face challenges with unreliable network connectivity. To tackle this, Ionic and GraphQL provide caching and offline support to enhance the user experience.
6.1 Implementing Caching with Ionic:
Apollo Client, by default, automatically caches query results. This means that if the same query is made again, Apollo Client will return the data from its cache instead of making a new request to the server.
6.2 Enabling Offline Support:
By integrating service workers and configuring the Apollo Client cache, you can enable offline support in your Ionic app. This allows users to continue using certain features of the app even without an internet connection.
7. Best Practices for Using Ionic and GraphQL Together:
To get the most out of Ionic and GraphQL in your mobile app development, consider the following best practices:
7.1 Avoiding Overly Complex Queries:
While GraphQL allows for powerful and flexible queries, be mindful of query complexity. Avoid excessively nested queries, as they may lead to performance issues on mobile devices.
7.2 Optimizing Queries for Mobile:
Optimize your GraphQL queries to minimize data transfer and reduce latency. Fetch only the necessary data, and avoid requesting large amounts of unnecessary information.
Conclusion
Ionic and GraphQL present a compelling combination for mobile app developers, offering powerful data fetching capabilities that improve performance, reduce unnecessary data transfer, and enhance the overall user experience. By following best practices and harnessing the potential of these technologies, you can build feature-rich and efficient mobile applications that cater to the needs of modern users.
With Ionic’s intuitive UI components and GraphQL’s flexible querying, your mobile apps are poised for success in today’s competitive market. Embrace the power of Ionic and GraphQL and elevate your mobile app development journey to new heights. Happy coding!
Table of Contents
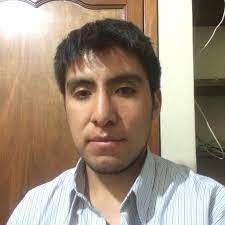
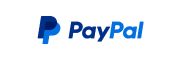