Ionic and Health Monitoring: Creating Personalized Fitness Apps
In today’s fast-paced world, health and fitness have become paramount concerns for many individuals. Whether it’s tracking daily steps, monitoring heart rate, or managing calorie intake, technology has evolved to play a vital role in our fitness routines. And one of the key players in this technological revolution is Ionic.
Table of Contents
Ionic is a versatile and powerful framework for building cross-platform mobile applications using web technologies such as HTML, CSS, and JavaScript. With its flexibility and rich ecosystem, Ionic is uniquely positioned to drive innovation in health monitoring by allowing developers to create personalized fitness apps that cater to the unique needs of users. In this blog post, we will explore how Ionic can be leveraged to build personalized fitness apps that empower users to take charge of their health and well-being.
1. Why Personalized Fitness Apps Matter
Before diving into the technical aspects of building fitness apps with Ionic, it’s essential to understand why personalized fitness apps are so crucial in today’s fitness landscape. Personalization is the key to making fitness routines more effective and sustainable for individuals. Here are some reasons why personalized fitness apps matter:
1.1. Tailored Workouts
Personalized fitness apps can analyze user data, such as age, gender, fitness goals, and current fitness level, to create customized workout plans. This ensures that users get the most out of their exercise routines while minimizing the risk of injury.
1.2. Data-Driven Insights
These apps can collect and analyze data from wearable devices, providing users with valuable insights into their health and fitness progress. Users can track their steps, heart rate, sleep patterns, and more, helping them make informed decisions about their lifestyle.
1.3. Motivation and Accountability
By setting goals and sending reminders, personalized fitness apps keep users motivated and accountable. Gamification elements, such as badges and rewards, further encourage users to stick to their fitness goals.
1.4. Health Monitoring
For individuals with specific health conditions, personalized fitness apps can provide real-time health monitoring and alerts. This can be life-saving for those at risk of heart issues, diabetes, or other medical conditions.
Now that we understand the importance of personalized fitness apps let’s explore how to create them using the Ionic framework.
2. Getting Started with Ionic
Ionic is a popular choice among developers for building cross-platform mobile applications because it allows you to use web technologies you’re already familiar with. To get started with Ionic, you need to have Node.js and npm (Node Package Manager) installed on your system. Here’s how to set up a basic Ionic project:
2.1. Install Ionic CLI
Open your terminal or command prompt and run the following command to install the Ionic CLI globally:
bash npm install -g @ionic/cli
2.2. Create a New Ionic Project
Once the installation is complete, you can create a new Ionic project by running:
bash ionic start my-fitness-app blank
Replace my-fitness-app with your desired project name and choose a template (e.g., “blank” for a basic template).
2.3. Navigate to Your Project
Go to your project’s directory:
bash cd my-fitness-app
Now that you have your Ionic project set up, let’s dive into building personalized fitness features.
3. Building Personalized Fitness Features
3.1. User Registration and Profiles
The first step in creating a personalized fitness app is to allow users to register and create profiles. Ionic provides a straightforward way to build user registration and profile management features. Here’s a code snippet for a basic registration form:
html <ion-header> <ion-toolbar> <ion-title>Register</ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-item> <ion-label position="floating">Email</ion-label> <ion-input [(ngModel)]="email"></ion-input> </ion-item> <ion-item> <ion-label position="floating">Password</ion-label> <ion-input [(ngModel)]="password" type="password"></ion-input> </ion-item> <ion-button expand="full" (click)="register()">Register</ion-button> </ion-content>
In this code, we’re using Ionic components like ion-header, ion-content, and ion-item to create a simple registration form. The (ngModel) directive is used for two-way data binding, allowing us to capture user input in the email and password variables.
In your TypeScript file, you can implement the register method to handle user registration:
javascript import { Component } from '@angular/core'; import { NavController } from '@ionic/angular'; @Component({ selector: 'app-registration', templateUrl: 'registration.page.html', styleUrls: ['registration.page.scss'], }) export class RegistrationPage { email: string = ''; password: string = ''; constructor(private navCtrl: NavController) {} register() { // Implement user registration logic here // You can use Firebase, AWS Cognito, or your preferred authentication service // Once registered, you can navigate to the user's profile page this.navCtrl.navigateForward('/profile'); } }
In this TypeScript code, we’re importing necessary dependencies and implementing the register method. You can integrate your preferred authentication service, such as Firebase or AWS Cognito, to handle user registration securely. After successful registration, users are navigated to their profile page.
3.2. Personalized Workout Plans
To create personalized workout plans, you’ll need to collect user data, including their fitness goals, age, gender, and fitness level. You can use Ionic’s form components to gather this information and create tailored workout plans accordingly.
Here’s a simple example of a form for collecting user data:
html <ion-header> <ion-toolbar> <ion-title>Profile</ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-item> <ion-label>Age</ion-label> <ion-input [(ngModel)]="age" type="number"></ion-input> </ion-item> <ion-item> <ion-label>Gender</ion-label> <ion-select [(ngModel)]="gender"> <ion-select-option value="male">Male</ion-select-option> <ion-select-option value="female">Female</ion-select-option> <ion-select-option value="other">Other</ion-select-option> </ion-select> </ion-item> <ion-item> <ion-label>Fitness Goal</ion-label> <ion-select [(ngModel)]="fitnessGoal"> <ion-select-option value="weight_loss">Weight Loss</ion-select-option> <ion-select-option value="muscle_gain">Muscle Gain</ion-select-option> <ion-select-option value="maintain">Maintain</ion-select-option> </ion-select> </ion-item> <ion-button expand="full" (click)="createWorkoutPlan()">Create Workout Plan</ion-button> </ion-content>
In this code, we’re using ion-select for dropdown selections and capturing the user’s age, gender, and fitness goal in variables (age, gender, fitnessGoal).
In your TypeScript file, you can implement the createWorkoutPlan method to generate personalized workout plans based on the user’s input:
javascript import { Component } from '@angular/core'; @Component({ selector: 'app-profile', templateUrl: 'profile.page.html', styleUrls: ['profile.page.scss'], }) export class ProfilePage { age: number = 0; gender: string = ''; fitnessGoal: string = ''; constructor() {} createWorkoutPlan() { // Implement workout plan generation logic here // Use user input to customize the workout plan } }
You can use algorithms and fitness knowledge to generate workout plans tailored to the user’s goals and physical condition. These plans can then be presented to the user in a clear and user-friendly manner.
3.3. Data Visualization and Progress Tracking
One of the key features of personalized fitness apps is the ability to visualize data and track progress over time. Ionic provides various charting libraries, such as Chart.js, that can be integrated into your app to display data beautifully. Here’s an example of how to use Chart.js in an Ionic app:
javascript // Install Chart.js npm install chart.js // Import Chart.js in your component import { Chart } from 'chart.js'; // In your component class export class ProgressPage { // Initialize a Chart instance progressChart: any; constructor() {} ngAfterViewInit() { // Create a chart context const ctx = document.getElementById('progressChart'); // Define chart data and options const data = { labels: ['Jan', 'Feb', 'Mar', 'Apr', 'May'], datasets: [ { label: 'Weight (kg)', data: [70, 69, 68, 67, 66], borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1, }, ], }; const options = { scales: { y: { beginAtZero: true, }, }, }; // Create the chart this.progressChart = new Chart(ctx, { type: 'line', data: data, options: options, }); } }
In this code, we’re using Chart.js to create a line chart that visualizes weight progress over time. We initialize the chart in the ngAfterViewInit lifecycle hook and provide data and options to customize the chart’s appearance.
By integrating such charts into your personalized fitness app, users can easily track their progress, whether it’s weight loss, muscle gain, or any other fitness metric.
4. Benefits of Using Ionic for Health Monitoring Apps
Now that we’ve explored how to build personalized fitness features with Ionic, let’s discuss the benefits of using Ionic for health monitoring apps:
4.1. Cross-Platform Compatibility
Ionic allows you to write code once and deploy it on multiple platforms, including iOS, Android, and the web. This significantly reduces development time and effort.
4.2. Extensive Plugin Ecosystem
Ionic offers a wide range of plugins that enable integration with various wearable devices, health trackers, and sensors. You can easily connect to devices like Fitbit, Apple Watch, or any Bluetooth-enabled fitness device.
4.3. Rich UI Components
Ionic provides a library of pre-designed UI components that can be customized to create visually appealing and user-friendly interfaces for health monitoring apps.
4.4. Rapid Prototyping
With Ionic’s ease of use and a rich set of development tools, you can quickly prototype and iterate on your health monitoring app, ensuring that it meets user needs and expectations.
4.5. Community and Support
Ionic has a vibrant community and extensive documentation, making it easier to find solutions to common development challenges and receive support when needed.
Conclusion
In today’s health-conscious world, personalized fitness apps have the potential to make a significant impact on users’ well-being. Ionic, with its cross-platform capabilities, extensive plugin ecosystem, and user-friendly UI components, is an ideal framework for building such apps. By implementing features like user registration, personalized workout plans, data visualization, and progress tracking, you can create fitness apps that cater to individual needs and empower users to lead healthier lives.
Whether you’re a seasoned developer or just getting started, Ionic provides the tools and resources you need to create personalized fitness apps that can revolutionize health monitoring. So, harness the power of Ionic and embark on your journey to create innovative and life-changing health and fitness applications.
Start building your personalized fitness app with Ionic today and help users achieve their fitness goals while staying healthy and motivated.
Table of Contents
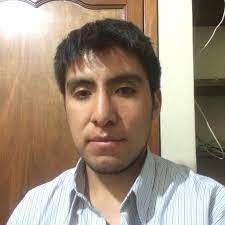
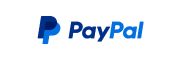