Ionic and Machine Learning: Adding Intelligence to Your Apps
In today’s digital era, mobile applications have become an integral part of our daily lives. Developers are continuously striving to create more intuitive and intelligent apps that can understand and cater to users’ needs better. This is where machine learning comes into play. By integrating machine learning capabilities into Ionic apps, developers can unlock a world of possibilities, from personalized user experiences to predictive analysis.
In this blog post, we will explore the fascinating synergy between Ionic and machine learning, how they complement each other, and how you can harness this combination to create smart, sophisticated applications that surpass traditional ones. We will dive into the fundamentals of machine learning, understand its application in mobile apps, and explore practical examples with code snippets.
1. Understanding Machine Learning
1.1. What is Machine Learning?
Machine learning is a subset of artificial intelligence that enables systems to learn from data without explicit programming. Instead of following predefined rules, machine learning algorithms can identify patterns and make data-driven decisions.
1.2. Machine Learning Types
- Supervised Learning: Algorithms are trained on labeled datasets, making predictions based on provided examples.
- Unsupervised Learning: Algorithms explore data without labeled outputs, finding patterns and relationships.
- Reinforcement Learning: Algorithms learn through trial and error, receiving feedback for their actions in a specific environment.
2. The Role of Machine Learning in Mobile Apps
Mobile applications can greatly benefit from integrating machine learning capabilities. Here are some ways machine learning can enhance Ionic apps:
2.1. Personalization
By analyzing user behavior and preferences, machine learning algorithms can offer personalized content and recommendations. Whether it’s suggesting relevant products or tailoring content, personalization enhances user engagement and satisfaction.
2.2. Image and Speech Recognition
Machine learning enables apps to recognize images and understand speech. This can be utilized for a wide range of applications, from facial recognition for security purposes to enabling voice-controlled interfaces.
2.3. Sentiment Analysis
By applying natural language processing (NLP) techniques, mobile apps can gauge users’ sentiments from their comments, reviews, or social media posts. This analysis can provide valuable insights into customer feedback and satisfaction levels.
2.4. Predictive Analytics
Predictive models built with machine learning can anticipate user preferences and behaviors, aiding in demand forecasting and targeted marketing strategies.
3. Integrating Machine Learning in Ionic Apps
Ionic offers a versatile platform to build cross-platform mobile applications using web technologies like HTML, CSS, and JavaScript. Combining this with machine learning capabilities empowers developers to create intelligent apps that deliver unique experiences to users.
To demonstrate this integration, we’ll walk through a step-by-step example of building an Ionic app with sentiment analysis using a pre-trained NLP model.
3.1. Prerequisites
Before we begin, make sure you have the following installed:
- Node.js and npm (Node Package Manager)
- Ionic CLI
Step 1: Set Up a New Ionic Project
First, let’s create a new Ionic project. Open your terminal and run the following commands:
bash npm install -g @ionic/cli ionic start SentimentAnalysis blank cd SentimentAnalysis
Step 2: Install Dependencies
Next, we’ll install the required dependencies for using machine learning in our Ionic app. We’ll use TensorFlow.js, a popular library for machine learning in JavaScript:
bash npm install @tensorflow/tfjs @tensorflow-models/nlp
Step 3: Create Sentiment Analysis Service
Now, let’s create a new file named sentiment.service.ts in the src/app/services directory. This service will handle the sentiment analysis using the pre-trained NLP model.
typescript // src/app/services/sentiment.service.ts import { Injectable } from '@angular/core'; import * as tf from '@tensorflow/tfjs'; import * as nlp from '@tensorflow-models/nlp'; @Injectable({ providedIn: 'root' }) export class SentimentService { private model: nlp.NLClassifier; async loadModel() { this.model = await nlp.load(); } async analyzeSentiment(text: string): Promise<string> { const result = await this.model.classify(text); return result.label; } }
In this service, we import the necessary libraries, load the NLP model, and create a method analyzeSentiment() to analyze the sentiment of a given text.
Step 4: Implement the Sentiment Analysis in the App
Next, we’ll modify the src/app/home/home.page.ts file to use the SentimentService and display the sentiment analysis results.
typescript // src/app/home/home.page.ts import { Component } from '@angular/core'; import { SentimentService } from '../services/sentiment.service'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage { inputText: string; sentimentResult: string; constructor(private sentimentService: SentimentService) {} async analyzeSentiment() { if (this.inputText) { this.sentimentResult = await this.sentimentService.analyzeSentiment(this.inputText); } else { this.sentimentResult = 'Input text is empty'; } } }
In this component, we’ve imported the SentimentService and added a method analyzeSentiment() to trigger the sentiment analysis. The result will be displayed in the sentimentResult variable.
Step 5: Design the User Interface
Now, let’s update the src/app/home/home.page.html file to include the input field and button for sentiment analysis.
html <!-- src/app/home/home.page.html --> <ion-header> <ion-toolbar> <ion-title> Sentiment Analysis App </ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-card> <ion-card-content> <ion-item> <ion-textarea [(ngModel)]="inputText" placeholder="Enter your text here..." rows="4" ></ion-textarea> </ion-item> <ion-button expand="full" (click)="analyzeSentiment()"> Analyze Sentiment </ion-button> <div *ngIf="sentimentResult"> <h2>Result: {{ sentimentResult }}</h2> </div> </ion-card-content> </ion-card> </ion-content>
In this UI, we have an input textarea to enter the text for sentiment analysis, a button to trigger the analysis, and a section to display the result.
Step 6: Run the Ionic App
Finally, let’s run our Ionic app on a development server to see the sentiment analysis in action.
bash ionic serve
Conclusion
In this blog post, we explored the exciting realm of Ionic and machine learning integration. We learned how machine learning can add intelligence to mobile apps, enabling features like personalization, image and speech recognition, sentiment analysis, and predictive analytics.
By following the step-by-step guide and code samples, you can now implement machine learning capabilities into your Ionic apps. This empowers you to build smarter, more intuitive applications that provide a superior user experience, setting your apps apart from the competition.
Table of Contents
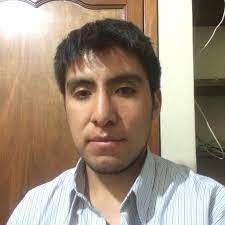
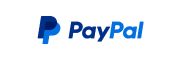