Optimizing Performance in Ionic Apps: Best Practices and Techniques
Creating high-performing Ionic apps is crucial for delivering a seamless user experience. Poor app performance can lead to user frustration and abandonment. Fortunately, there are several best practices and techniques you can implement to optimize the performance of your Ionic apps. In this blog, we will explore various strategies to improve speed, efficiency, and overall performance.
Use Lazy Loading
Lazy loading is a powerful technique that loads modules and components on demand, rather than all at once when the app initializes. By implementing lazy loading, you can significantly reduce the initial load time of your app. This is especially beneficial for large apps with numerous pages and complex components.
To enable lazy loading in Ionic, simply use the IonicModule.forChild() method when defining your routes. This ensures that each page or component is loaded only when it is required, resulting in a faster initial load time.
typescript const routes: Routes = [ { path: 'lazy', loadChildren: () => import('./lazy/lazy.module').then(m => m.LazyPageModule) } ];
Optimize Images
Images often contribute to the bulk of an app’s size and can significantly impact performance. It’s crucial to optimize images to reduce their file size without compromising quality. Consider the following techniques:
1. Compress images: Use tools like imagemin or online services to compress your images without sacrificing quality.
2. Use lazy loading for images: Load images only when they are in the user’s viewport or about to be displayed. Ionic provides the lazy-img directive, which helps implement lazy loading for images effortlessly.
html <ion-img [src]="'path/to/image.jpg'" [lazy]="true"></ion-img>
Minify and Bundle Code
Minifying and bundling your code can significantly improve app performance by reducing the file size and the number of requests made by the app. Ionic leverages the power of Angular’s build system, which includes minification and bundling by default.
To build your Ionic app with optimized code, use the following command:
bash ionic build --prod
This will generate a minified and bundled version of your app, ready for production deployment.
Reduce HTTP Requests
Excessive HTTP requests can impact app performance, especially in scenarios where the app needs to fetch data from various sources. Minimizing the number of requests is crucial for improving performance.
- Combine API requests: If your app requires multiple API calls, consider combining them into a single request using techniques like batch processing or server-side aggregation.
- Cache API responses: Implement client-side caching to store API responses. This reduces the need for subsequent requests and improves app performance by retrieving data locally.
Optimize Data Binding
Efficient data binding is essential for improving the performance of Ionic apps. Excessive data binding can lead to unnecessary updates and re-rendering, impacting app speed. Consider the following practices:
- Use one-time binding: If your data doesn’t change frequently, consider using one-time binding ([property]=”::value”) instead of two-way binding ([(property)]=”value”) to prevent unnecessary updates.
- Debounce input events: When capturing user input, debounce the event to reduce the number of updates and improve performance.
typescript <ion-input (ionChange)="onInputChange($event)" debounce="500"></ion-input>
Optimize CSS
CSS optimization plays a significant role in improving the rendering performance of your Ionic app. Consider these techniques:
- Minify CSS: Remove unnecessary spaces, comments, and reduce the size of your CSS files. Use tools like cssnano or online services to minify your CSS.
- Use efficient CSS selectors: Avoid using complex selectors, as they can impact rendering performance. Opt for simpler selectors whenever possible.
Test and Profile Performance
Regularly testing and profiling your Ionic app’s performance is essential to identify bottlenecks and areas for improvement. Ionic provides tools like the Chrome DevTools and the Ionic DevApp for testing and profiling your app’s performance.
Use Chrome DevTools to analyze network requests, JavaScript execution, memory usage, and more. Ionic DevApp allows you to test your app on multiple devices simultaneously, providing valuable insights into performance across various platforms.
Conclusion
Optimizing performance in Ionic apps is crucial for delivering a fast and responsive user experience. By following these best practices and implementing the recommended techniques, you can significantly enhance the speed, efficiency, and overall performance of your Ionic apps. Remember to test and profile your app regularly to identify any performance bottlenecks and continuously improve your app’s performance. With these strategies in place, you’ll be able to create highly performant Ionic apps that keep your users engaged and satisfied.
Remember, optimizing performance in Ionic apps is a continuous process. Stay updated with the latest advancements and keep experimenting with new techniques to ensure your app performs at its best.
Table of Contents
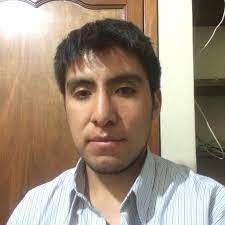
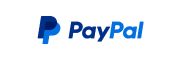