Ionic and User Onboarding: Engaging Users from the Start
User onboarding is the critical process of introducing new users to your mobile app and helping them become familiar with its features and functionalities. It’s not just about showing users where to click or tap; it’s about creating an engaging and enjoyable experience that leaves a lasting impression. In this blog post, we will explore how to use Ionic, a popular framework for building cross-platform mobile apps, to create a compelling user onboarding experience that keeps users engaged from the moment they first launch your app.
Table of Contents
1. Why User Onboarding Matters
Before diving into the technical aspects of implementing user onboarding in Ionic, let’s understand why it’s crucial for the success of your mobile app.
1.1. First Impressions Matter
The first interaction a user has with your app sets the tone for their entire experience. If your onboarding process is confusing, overwhelming, or uninspiring, users may abandon your app before even giving it a chance.
1.2. Reduces Churn Rate
User onboarding can significantly reduce your app’s churn rate. By guiding users through the app’s features and functionalities, you increase the likelihood that they will stick around and continue to use your app.
1.3. Increases User Engagement
Engaging onboarding experiences can pique users’ interest and encourage them to explore your app further. When users feel confident and comfortable with your app, they are more likely to become active and engaged users.
1.4. Enhances User Satisfaction
A well-designed onboarding process can make users feel valued and understood. It shows that you care about their experience and want to make it as smooth as possible.
Now that we’ve established the importance of user onboarding, let’s dive into how to implement it effectively using Ionic.
2. Getting Started with Ionic
Ionic is a popular open-source framework for building cross-platform mobile apps using web technologies like HTML, CSS, and JavaScript. It provides a set of UI components, a flexible grid system, and tools for building beautiful and interactive mobile applications.
If you haven’t already, you’ll need to set up Ionic on your development environment. You can do this by following the official Ionic installation guide for your platform.
Once you have Ionic installed, you can start creating your mobile app and integrating user onboarding.
3. Designing an Engaging Onboarding Experience
Effective user onboarding is a delicate balance between providing information and keeping users engaged. Here are some design principles to keep in mind:
3.1. Keep It Short and Sweet
Your onboarding process should be concise and to the point. Avoid overwhelming users with too much information. Focus on the essential features and benefits of your app.
3.2. Use Visuals
Visual elements such as images, icons, and animations can help convey information more effectively and make the onboarding process more engaging.
3.3. Interactive Tutorials
Consider adding interactive tutorials that allow users to try out key features while they’re learning. This hands-on approach can be very effective.
3.4. Personalization
Tailor the onboarding experience to the user’s preferences and needs. Ask for their input and adjust the onboarding flow accordingly.
Now, let’s explore how to implement these principles using Ionic.
4. Implementing User Onboarding in Ionic
Ionic provides a robust set of tools and components for building user interfaces, making it an excellent choice for creating engaging onboarding experiences. Here’s a step-by-step guide on how to implement user onboarding in Ionic:
4.1. Create the Onboarding Page
In Ionic, you can create a dedicated onboarding page using Ionic’s routing system. This page will serve as the starting point for your onboarding experience.
typescript // Create a new Ionic page for onboarding ionic generate page onboarding
4.2. Design the Onboarding UI
Design a visually appealing onboarding UI using Ionic’s UI components. You can add slides, images, and text to introduce users to your app’s features.
html <!-- onboarding.page.html --> <ion-header> <ion-toolbar> <ion-title> Welcome to MyApp </ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-slides pager="true"> <ion-slide> <h2>Discover</h2> <p>Explore exciting features and functionalities.</p> <ion-img src="assets/img/discover.png"></ion-img> </ion-slide> <!-- Add more slides as needed --> </ion-slides> </ion-content>
4.3. Add Navigation Buttons
Include navigation buttons or gestures to allow users to move forward and backward through the onboarding slides.
html <!-- onboarding.page.html --> <ion-footer> <ion-button (click)="nextSlide()" expand="full" fill="solid">Next</ion-button> </ion-footer>
4.4. Implement Logic
In your onboarding page’s TypeScript file, implement the logic for handling slide navigation and completing the onboarding process.
typescript // onboarding.page.ts import { Component } from '@angular/core'; import { Router } from '@angular/router'; @Component({ selector: 'app-onboarding', templateUrl: './onboarding.page.html', styleUrls: ['./onboarding.page.scss'], }) export class OnboardingPage { constructor(private router: Router) {} nextSlide() { // Implement logic to navigate to the next slide } finishOnboarding() { // Implement logic to complete the onboarding process and navigate to the main app } }
4.5. Customize to User Preferences
To personalize the onboarding experience, you can add optional questions or settings screens that users can skip or complete based on their preferences.
html <!-- settings.page.html --> <ion-content> <ion-list> <ion-item> <ion-label>Choose your interests</ion-label> <ion-select [(ngModel)]="selectedInterests" multiple="true"> <ion-select-option value="sports">Sports</ion-select-option> <ion-select-option value="music">Music</ion-select-option> <!-- Add more options as needed --> </ion-select> </ion-item> </ion-list> </ion-content> typescript Copy code // settings.page.ts import { Component } from '@angular/core'; @Component({ selector: 'app-settings', templateUrl: './settings.page.html', styleUrls: ['./settings.page.scss'], }) export class SettingsPage { selectedInterests: string[] = []; constructor() {} }
By providing users with the option to customize their experience, you make them feel more connected to your app.
4.6. Track User Progress
To keep users engaged, you can add progress indicators to show how far they’ve progressed in the onboarding process.
html <!-- onboarding.page.html --> <ion-footer> <ion-progress-bar [value]="slideIndex / totalSlides"></ion-progress-bar> <ion-button (click)="nextSlide()" expand="full" fill="solid">Next</ion-button> </ion-footer> typescript Copy code // onboarding.page.ts import { Component } from '@angular/core'; import { Router } from '@angular/router'; @Component({ selector: 'app-onboarding', templateUrl: './onboarding.page.html', styleUrls: ['./onboarding.page.scss'], }) export class OnboardingPage { slideIndex: number = 0; totalSlides: number = 3; // Update with the actual number of onboarding slides constructor(private router: Router) {} nextSlide() { if (this.slideIndex < this.totalSlides - 1) { this.slideIndex++; } else { this.finishOnboarding(); } } finishOnboarding() { // Implement logic to complete the onboarding process and navigate to the main app } }
Conclusion
User onboarding is a critical part of creating a successful mobile app. It’s your opportunity to make a great first impression, reduce churn, increase engagement, and enhance user satisfaction. With Ionic, you have the tools and flexibility to design and implement engaging onboarding experiences that captivate users from the moment they launch your app.
Remember to keep the onboarding process short and visually appealing, offer personalization options, and track user progress. By following these principles and best practices, you can create a compelling onboarding experience that sets your app apart and keeps users coming back for more. Start building your engaging onboarding experience with Ionic today!
Table of Contents
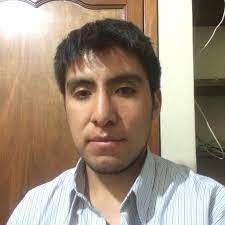
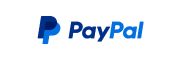