Ionic and Wearable Devices: Developing Apps for Smartwatches
In the era of rapidly evolving technology, wearable devices have become an integral part of our daily lives. Smartwatches, in particular, have gained immense popularity due to their versatility and ability to provide real-time information at a glance. As a developer, tapping into this growing market by creating apps for smartwatches can be a lucrative venture. In this blog, we’ll dive into the world of smartwatch app development using Ionic, a powerful framework. We’ll explore its features, provide code samples, and share tips to help you get started on your wearable development journey.
Table of Contents
1. Why Develop Apps for Smartwatches?
1.1. Growing Market Potential
Smartwatches have witnessed significant market growth in recent years, with users relying on them for fitness tracking, notifications, and even productivity apps. This surge in popularity presents a massive opportunity for developers to create unique and useful applications.
1.2. Enhanced User Experience
Smartwatches offer a unique user experience due to their compact form factor and quick-access nature. Developing apps for these devices allows you to provide users with an efficient and seamless way to interact with your software.
1.3. Diversification of Your Skillset
Venturing into smartwatch app development not only opens up new opportunities but also diversifies your skillset as a developer. Learning to design and code for wearable devices can be a valuable addition to your repertoire.
2. Getting Started with Ionic for Smartwatch App Development
Before we dive into the technical aspects, let’s set up our development environment and get acquainted with the tools and frameworks we’ll be using.
2.1. Prerequisites
To start developing apps for smartwatches with Ionic, you’ll need:
- Node.js: Ensure you have Node.js installed on your system, as Ionic is heavily reliant on it.
- Ionic CLI: Install the Ionic CLI globally using npm.
bash npm install -g @ionic/cli
2.2. Create a New Ionic App
Let’s create a new Ionic app using the Ionic CLI. Navigate to your desired directory and run the following command:
bash ionic start mySmartwatchApp blank
This command will set up a new Ionic project with the name “mySmartwatchApp” using the “blank” template. You can replace “blank” with other templates like “tabs” or “sidemenu” depending on your project requirements.
2.3. Platform Setup
Ionic allows you to develop apps for multiple platforms, including Android and iOS. To target smartwatches, we’ll need to add the appropriate platform:
bash ionic platform add android ionic platform add ios
2.4. Designing for Smartwatches
Designing for smartwatches requires a different approach than traditional mobile app design. Keep these key points in mind:
- Screen Size: Smartwatches have limited screen real estate, so focus on concise and essential information.
- Interactions: Utilize swipe gestures, taps, and voice commands for user interactions.
- Notifications: Smartwatches excel at delivering notifications, so make sure your app leverages this feature.
3. Building Your First Smartwatch App
Now that we have our development environment set up, let’s dive into building a simple smartwatch app using Ionic.
3.1. Create a New Page
In Ionic, each screen or view is represented as a page. To create a new page for our smartwatch app, run the following command:
bash ionic generate page myPage
This command will generate a new page named “myPage.”
3.2. Customize the Page
Navigate to the newly created page folder in your project directory. Here, you can customize the HTML, CSS, and TypeScript files to design your app’s interface and functionality.
For instance, you can create a basic interface with a title and a button:
myPage.html
html <ion-header> <ion-toolbar> <ion-title> My Smartwatch App </ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-button (click)="sendMessage()">Send Message</ion-button> </ion-content>
myPage.ts
typescript import { Component } from '@angular/core'; @Component({ selector: 'app-my-page', templateUrl: './my-page.page.html', styleUrls: ['./my-page.page.scss'], }) export class MyPagePage { constructor() {} sendMessage() { // Add code here to send a message or perform an action } }
3.3. Navigation
To navigate to your newly created page, you can use the Ionic NavController. Open your app’s root page (usually located in the src/app/app-routing.module.ts file) and configure the routing:
app-routing.module.ts
typescript import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; const routes: Routes = [ { path: '', redirectTo: 'my-page', pathMatch: 'full' }, { path: 'my-page', loadChildren: () => import('./my-page/my-page.module').then(m => m.MyPagePageModule) }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule {}
Now, when you navigate to the root URL of your app, it will redirect to your “myPage” page.
3.4. Testing Your App
To test your app on a smartwatch emulator or a physical device, you can use the Ionic CLI. For example, to run your app on an Android emulator, use the following command:
bash ionic cordova emulate android
And for iOS:
bash ionic cordova emulate ios
4. Enhancing Your Smartwatch App
Now that you have a basic smartwatch app up and running, let’s explore some ways to enhance it.
4.1. Use Sensors
Smartwatches often come equipped with various sensors like accelerometers, heart rate monitors, and GPS. You can leverage these sensors to create fitness or health-related apps.
For instance, you can access the accelerometer data to create a pedometer app that tracks the user’s steps or a workout app that monitors their movements.
4.2. Notifications
Utilize smartwatch notifications to keep users engaged with your app. Send timely reminders or updates to their wrist, ensuring they stay connected to your app’s services.
typescript // Sending a notification in Ionic import { LocalNotifications } from '@ionic-native/local-notifications/ngx'; constructor(private localNotifications: LocalNotifications) {} sendNotification() { this.localNotifications.schedule({ title: 'My App', text: 'Don't forget to drink water!', foreground: true, // Display even when the app is in the foreground }); }
4.3. Voice Commands
Leverage voice commands to create hands-free experiences. Smartwatches often come with built-in voice recognition, making it convenient for users to interact with your app.
typescript // Using voice recognition in Ionic import { SpeechRecognition } from '@ionic-native/speech-recognition/ngx'; constructor(private speechRecognition: SpeechRecognition) {} startListening() { this.speechRecognition.startListening() .subscribe( (matches: string[]) => { // Handle voice commands }, (error: any) => console.error(error) ); }
5. Challenges and Considerations
While developing apps for smartwatches can be rewarding, it comes with its own set of challenges and considerations:
5.1. Limited Resources
Smartwatches typically have limited processing power, memory, and battery life. Optimizing your app for these constraints is crucial.
5.2. Design Constraints
Designing for small screens requires careful consideration of user interface elements, fonts, and touch targets to ensure a seamless user experience.
5.3. Platform Variations
Different smartwatch platforms, such as Wear OS and watchOS, may have varying development requirements and capabilities. Be prepared to adapt your app accordingly.
6. Testing on Real Devices
To ensure your app works flawlessly on real smartwatches, consider testing on multiple devices with different screen sizes and operating systems.
Conclusion
Developing apps for smartwatches is a rewarding endeavor with a growing user base and a unique set of challenges. With Ionic, you can leverage your web development skills to create engaging and functional apps for these wearable devices. Whether you’re building fitness apps, productivity tools, or innovative utilities, the world of smartwatch app development holds immense potential. So, roll up your sleeves, dive into the code, and start crafting your next smartwatch masterpiece!
Table of Contents
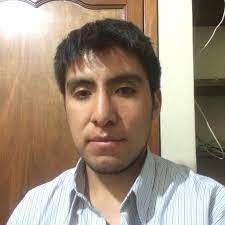
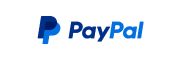