Objective-C Animation and Graphics: Adding Visual Appeal to iOS Apps
In the fast-paced world of mobile app development, creating visually appealing and engaging user interfaces is crucial. Objective-C, the language that has powered iOS app development for years, offers a robust set of tools for achieving this goal. In this blog, we’ll explore how Objective-C can be used to add animation and graphics to your iOS apps, elevating the user experience to new heights.
Table of Contents
1. Why Animation and Graphics Matter
Before we dive into the technical aspects of adding animation and graphics to your iOS apps, let’s take a moment to understand why they are so essential.
- Enhanced User Engagement: Visually pleasing animations and graphics capture users’ attention and keep them engaged with your app. They create a memorable user experience, making users more likely to return.
- Improved User Interface: Animation can make your app’s user interface more intuitive and user-friendly. It can guide users through complex processes and provide feedback on their actions.
- Brand Identity: Well-crafted animations and graphics can help establish and reinforce your app’s brand identity. They make your app stand out from the competition and leave a lasting impression.
Now that we know why animation and graphics are crucial, let’s delve into how Objective-C can be used to implement them effectively.
2. Core Animation Framework
Objective-C provides the Core Animation framework, a powerful toolset for creating smooth animations and graphics. Core Animation is based on the CALayer class, which represents the visual content of your app. Here are some key concepts and techniques:
2.1. Layer-Based Animation
Layer-based animation is at the core of Core Animation. You can animate properties of CALayer objects, such as position, size, opacity, and more. Let’s look at an example of animating the position of a view using Objective-C code:
objective // Create a new CALayer CALayer *myLayer = [CALayer layer]; myLayer.frame = CGRectMake(0, 0, 100, 100); myLayer.backgroundColor = [UIColor blueColor].CGColor; // Add the layer to a view's layer [self.view.layer addSublayer:myLayer]; // Create a basic animation for the position property CABasicAnimation *positionAnimation = [CABasicAnimation animationWithKeyPath:@"position"]; positionAnimation.fromValue = [NSValue valueWithCGPoint:CGPointMake(0, 0)]; positionAnimation.toValue = [NSValue valueWithCGPoint:CGPointMake(200, 200)]; positionAnimation.duration = 1.0; // Animation duration in seconds // Add the animation to the layer [myLayer addAnimation:positionAnimation forKey:@"position"];
In this code sample, we create a CALayer, set its initial position and background color, and then animate its position to a new location.
2.2. View Animation
Objective-C also provides UIView animations, which simplify the process of animating UIViews. UIView animations allow you to animate changes to view properties with concise and readable code. Here’s an example of a simple fade-in animation:
objective [UIView animateWithDuration:0.5 animations:^{ myView.alpha = 1.0; // Set the view's alpha (opacity) to 1.0 for a fade-in effect }];
UIView animations are great for common animations like fades, slides, and scaling effects.
3. Graphics with Core Graphics
In addition to animations, Objective-C allows you to create custom graphics using Core Graphics (Quartz 2D). Core Graphics is a powerful drawing framework that enables you to generate images, charts, and custom UI elements. Let’s explore how to draw a simple shape using Core Graphics:
3.1. Drawing a Rectangle
objective - (void)drawRect:(CGRect)rect { CGContextRef context = UIGraphicsGetCurrentContext(); CGContextSetFillColorWithColor(context, [UIColor blueColor].CGColor); CGContextFillRect(context, CGRectMake(50, 50, 100, 100)); }
In this example, we override the drawRect: method of a custom UIView subclass to draw a blue rectangle. Core Graphics provides various drawing functions to create complex shapes, gradients, and paths.
4. Incorporating Animation and Graphics in Your App
Now that we’ve covered the basics of animation and graphics in Objective-C, let’s discuss how to effectively incorporate them into your iOS app:
4.1. Plan Your Animations
Before you start coding, plan your animations and graphics carefully. Consider the user experience and the message you want to convey. Sketch your animations on paper or using design tools to visualize the desired outcome.
4.2. Keep Performance in Mind
Smooth animations and graphics are essential, but they should not compromise app performance. Test your animations on various devices and optimize them as needed. Use tools like Instruments to identify and address performance bottlenecks.
4.3. Use Animation Libraries
Objective-C has a vibrant community, and several animation libraries are available to simplify complex animations. Libraries like “pop” and “Facebook Shimmer” provide pre-built animations that you can easily integrate into your app.
4.4. Utilize UIKit Dynamics
UIKit Dynamics is a framework that simulates real-world physics in your UI. It’s excellent for creating realistic animations, such as bouncing buttons or interactive elements. Take advantage of UIKit Dynamics to add depth and realism to your app.
5. Expert Tips for Stunning Visuals
To take your iOS app’s visual appeal to the next level, consider these expert tips:
5.1. Layer Compositing
Combine multiple CALayers to create intricate animations and effects. You can apply masks, shadows, and blending modes to achieve stunning visuals.
5.2. Keyframe Animations
Keyframe animations allow you to define multiple points in an animation sequence. They are perfect for creating complex animations with precise control over timing and easing.
5.3. Interactive Graphics
Implement touch interactions within your custom graphics. Allow users to manipulate and engage with your graphics for a more immersive experience.
5.4. 3D Graphics
If your app requires 3D graphics, consider using SceneKit or OpenGL for advanced rendering and animations.
5.5. Consistency
Maintain a consistent design language throughout your app. Use animations and graphics that align with your app’s branding and style guide.
Conclusion
Incorporating animation and graphics into your iOS app can significantly enhance its visual appeal and user engagement. Objective-C, with its Core Animation framework and Core Graphics capabilities, provides the tools you need to bring your app to life. By carefully planning your animations, optimizing for performance, and following expert tips, you can create stunning visuals that leave a lasting impression on your users. So, go ahead and add that extra layer of magic to your iOS app with animation and graphics powered by Objective-C. Your users will thank you for it. Happy coding!
As you embark on your journey to create visually captivating iOS apps, remember that the key to success lies in creativity, experimentation, and attention to detail. With Objective-C’s animation and graphics capabilities at your disposal, you have the tools you need to make your app stand out in the crowded App Store. Happy coding!
Table of Contents
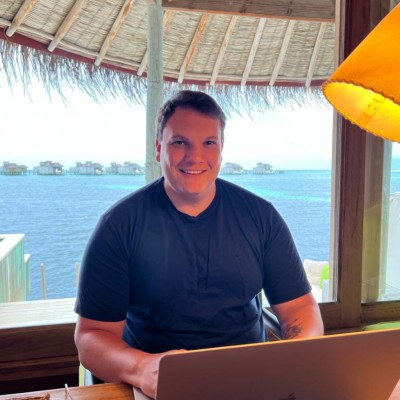
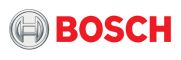