Objective-C and Firebase: Powering Real-Time iOS Apps
In the ever-evolving landscape of mobile app development, creating real-time applications that seamlessly sync data across devices is a must. Objective-C, the language that has long been at the core of iOS app development, can be combined with Firebase to build feature-rich real-time iOS apps. In this comprehensive guide, we’ll delve into the world of Objective-C and Firebase, exploring the integration, key features, and best practices to build robust real-time iOS applications.
Table of Contents
1. Why Objective-C and Firebase?
Before we dive into the technical details, let’s understand why Objective-C and Firebase make a compelling choice for building real-time iOS apps.
1.1. Objective-C’s Legacy
Objective-C has a rich history in iOS app development, dating back to the early days of the iPhone. While Swift has gained popularity in recent years, Objective-C still plays a vital role in maintaining and extending legacy iOS apps. If you’re working on an existing Objective-C project or prefer its syntax, Firebase can seamlessly integrate with it.
1.2. Firebase’s Real-Time Database
Firebase, a comprehensive mobile and web application development platform, offers a real-time database as one of its core features. This real-time database allows developers to synchronize data across devices in real-time, making it an ideal choice for creating responsive and interactive iOS apps.
1.3. Firebase Authentication
Firebase provides robust authentication services, enabling you to secure your real-time iOS app with ease. Whether you need email/password authentication, social media login, or even custom authentication methods, Firebase has you covered.
Now that we’ve established why Objective-C and Firebase are a potent combination let’s explore the integration process and key concepts in building real-time iOS apps.
2. Integration of Firebase with Objective-C
Integrating Firebase into your Objective-C project is a straightforward process. Follow these steps to get started:
Step 1: Create a Firebase Project
If you don’t already have a Firebase project, go to the Firebase Console, create a new project, and follow the setup instructions.
Step 2: Install Firebase SDK
To integrate Firebase into your Objective-C project, you’ll need to add the Firebase SDK. The Firebase SDK is available through CocoaPods, a popular dependency manager for iOS projects. If you haven’t installed CocoaPods yet, run the following command:
shell $ gem install cocoapods
Next, create a Podfile in your project’s directory and add the Firebase dependencies you need. For real-time database and authentication, you can use the following:
ruby pod 'Firebase/Core' pod 'Firebase/Database' pod 'Firebase/Auth'
Then, run the following command to install the dependencies:
shell $ pod install
Step 3: Configure Firebase
In your Objective-C project, you’ll need to configure Firebase with your Firebase project’s credentials. Import the Firebase module and configure Firebase in your app delegate:
objective @import Firebase; // ... - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { [FIRApp configure]; // ... return YES; }
With Firebase integrated into your Objective-C project, you’re ready to start building real-time iOS apps.
3. Key Concepts in Firebase Real-Time Database
Firebase’s real-time database operates on the concept of a JSON tree, where data is stored in a hierarchical structure. Let’s explore some key concepts:
3.1. DatabaseReference
A DatabaseReference is a reference to a specific location in your Firebase real-time database. You can use it to read or write data to that location. Here’s how to create a DatabaseReference:
objective FIRDatabaseReference *ref = [[FIRDatabase database] reference];
3.2. Real-Time Updates
One of the standout features of Firebase is its real-time updates. When you read data from a DatabaseReference, any changes made to that data are automatically synchronized in real-time. This makes it perfect for chat applications, live sports scores, and collaborative tools.
3.3. Data Snapshot
A DataSnapshot contains the data at a specific location in the Firebase real-time database. You can use this snapshot to extract data:
objective [ref observeEventType:FIRDataEventTypeValue withBlock:^(FIRDataSnapshot *snapshot) { NSDictionary *post = snapshot.value; NSLog(@"Post: %@", post); }];
3.4. Authentication
Firebase provides robust authentication services. You can easily authenticate users using email/password, social media logins, or custom authentication methods. Here’s how you can authenticate a user with email and password:
objective [[FIRAuth auth] signInWithEmail:@"user@example.com" password:@"password" completion:^(FIRAuthDataResult * _Nullable authResult, NSError * _Nullable error) { if (error) { NSLog(@"Authentication failed: %@", error); } else { NSLog(@"Authentication successful!"); } }];
4. Building a Real-Time iOS App with Objective-C and Firebase
Now that we have a solid understanding of the integration and key concepts let’s build a simple real-time iOS app that demonstrates Firebase’s power.
4.1. Scenario: Real-Time Chat App
We’ll create a basic real-time chat application where users can send and receive messages in real-time.
Step 1: Set Up the User Interface
First, create a user interface with a table view to display messages and a text field to input new messages.
objective @interface ChatViewController () <UITableViewDelegate, UITableViewDataSource> @property (weak, nonatomic) IBOutlet UITableView *tableView; @property (weak, nonatomic) IBOutlet UITextField *messageTextField; @property (strong, nonatomic) NSMutableArray *messages; @end
Step 2: Initialize Firebase
In your viewDidLoad method, initialize Firebase and set up a reference to the chat room in your database.
objective FIRDatabaseReference *chatRef = [[[FIRDatabase database] reference] child:@"chat_room"];
Step 3: Send and Receive Messages
To send a message, you can add an event handler to a button press:
objective - (IBAction)sendMessage:(id)sender { NSString *messageText = self.messageTextField.text; FIRDatabaseReference *newMessageRef = [chatRef childByAutoId]; NSDictionary *message = @{@"text": messageText, @"sender": @"user123"}; [newMessageRef setValue:message]; self.messageTextField.text = @""; }
And to receive and display messages in real-time, add an observer to the chat reference:
objective [chatRef observeEventType:FIRDataEventTypeChildAdded withBlock:^(FIRDataSnapshot *snapshot) { NSDictionary *message = snapshot.value; [self.messages addObject:message]; [self.tableView reloadData]; }];
Step 4: Authentication
To keep the chat secure, you can implement user authentication using Firebase Authentication. Users must sign in to use the chat.
5. Best Practices for Real-Time iOS Apps
Building real-time iOS apps with Objective-C and Firebase is exciting, but it comes with its set of challenges. Here are some best practices to keep in mind:
5.1. Data Structure
Design your database structure carefully. Organize data in a way that minimizes read and write operations and ensures efficient real-time updates.
5.2. Security Rules
Use Firebase Security Rules to control who can access your data and how they can access it. Define rules that match your app’s specific requirements.
5.3. Offline Support
Firebase provides offline support out of the box. Make sure to handle offline scenarios gracefully, and implement conflict resolution strategies if necessary.
5.4. Scalability
Plan for scalability from the start. Firebase can handle a large number of concurrent connections, but optimizing your database structure is crucial for long-term success.
Conclusion
Objective-C may be considered a legacy language, but when combined with Firebase, it can still create cutting-edge real-time iOS applications. Firebase’s real-time database, authentication, and synchronization capabilities make it a compelling choice for developers looking to build responsive and interactive iOS apps.
In this guide, we covered the integration of Firebase with Objective-C, explored key concepts in Firebase’s real-time database, and built a real-time chat app as an example. We also discussed best practices to ensure the scalability and security of your real-time iOS apps.
So, whether you’re working on a legacy iOS project or simply prefer Objective-C’s syntax, you now have the knowledge and tools to create powerful real-time iOS apps that can compete in today’s dynamic app market. Happy coding!
Now, go ahead and explore the world of real-time iOS app development with Objective-C and Firebase, and unlock the potential to create engaging, interactive experiences for your users.
Table of Contents
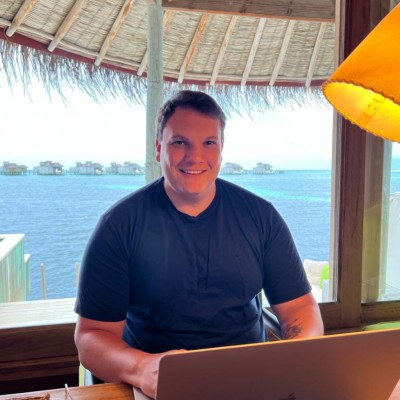
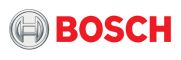