Objective-C and HealthKit: Integrating Health and Fitness in iOS
In the era of smart devices and a growing emphasis on health and fitness, iOS app developers have a unique opportunity to create applications that empower users to monitor and improve their well-being. Apple’s HealthKit framework, coupled with Objective-C, provides a robust platform for integrating health and fitness features into your iOS apps. In this comprehensive guide, we will explore the intricacies of Objective-C and HealthKit integration, providing you with the knowledge and code samples you need to build impactful health and fitness applications.
Table of Contents
1. Why Integrate HealthKit into Your iOS App?
Before we dive into the technical aspects, let’s understand why integrating HealthKit into your iOS app is a game-changer.
1.1. Seamless Data Access
HealthKit allows your app to seamlessly access health and fitness data collected by the user’s Apple devices. This includes data from the Apple Watch, iPhone, and other connected accessories. By integrating HealthKit, your app can provide a holistic view of the user’s health and fitness journey.
1.2. User Engagement
Health and fitness apps have seen tremendous growth in recent years. By offering features like step tracking, heart rate monitoring, and sleep analysis, you can engage users with a compelling and personalized experience.
1.3. Privacy and Security
Apple places a strong emphasis on user privacy. When you integrate HealthKit, you’re building on a platform that ensures user data is protected and permission-based. This fosters trust among your users.
1.4. Ecosystem Integration
By integrating HealthKit, your app becomes part of the broader Apple ecosystem. Users can see their health and fitness data in the Health app, and it can be shared with other health-related apps, creating a seamless experience for users.
Now that we understand the benefits, let’s dive into the technical details of integrating HealthKit with Objective-C.
2. Getting Started with HealthKit
2.1. Project Setup
To get started, open your Xcode project or create a new one. Ensure that your project is configured to use Objective-C as the primary language.
2.2. Importing HealthKit
You need to import the HealthKit framework into your project. To do this, add the following line to your Objective-C source file:
objective #import <HealthKit/HealthKit.h>
2.3. Requesting Authorization
Before accessing health and fitness data, your app must request permission from the user. You can use the HKHealthStore class to request authorization for specific data types. Here’s a code snippet that demonstrates how to request authorization for step count data:
objective HKHealthStore *healthStore = [[HKHealthStore alloc] init]; NSSet *readTypes = [NSSet setWithObject:[HKObjectType quantityTypeForIdentifier:HKQuantityTypeIdentifierStepCount]]; [healthStore requestAuthorizationToShareTypes:nil readTypes:readTypes completion:^(BOOL success, NSError *error) { if (success) { // User granted permission, you can now access health data } else { // Authorization request denied or failed } }];
This code creates an instance of HKHealthStore, specifies the data types your app wants to read (in this case, step count), and presents a request for user authorization.
3. Reading Health and Fitness Data
Now that your app has obtained authorization, you can start reading health and fitness data from HealthKit. Let’s explore how to retrieve step count data as an example.
3.1. Querying Step Count Data
HealthKit uses queries to retrieve data. To query step count data, you can use an HKSampleQuery. Here’s a code snippet to fetch the user’s daily step count:
objective HKSampleType *stepCountType = [HKSampleType quantityTypeForIdentifier:HKQuantityTypeIdentifierStepCount]; NSCalendar *calendar = [NSCalendar currentCalendar]; NSDateComponents *components = [calendar components:NSCalendarUnitYear | NSCalendarUnitMonth | NSCalendarUnitDay fromDate:[NSDate date]]; NSDate *startDate = [calendar dateFromComponents:components]; NSDate *endDate = [calendar dateByAddingUnit:NSCalendarUnitDay value:1 toDate:startDate options:0]; NSPredicate *predicate = [HKQuery predicateForSamplesWithStartDate:startDate endDate:endDate options:HKQueryOptionStrictStartDate]; HKSampleQuery *query = [[HKSampleQuery alloc] initWithSampleType:stepCountType predicate:predicate limit:HKObjectQueryNoLimit sortDescriptors:nil resultsHandler:^(HKSampleQuery *query, NSArray *results, NSError *error) { if (!error && results) { // Process step count data here } else { // Handle error } }]; [healthStore executeQuery:query];
In this code, we create an HKSampleType for step count data and define a time range for the query (in this case, the current day). The HKSampleQuery is executed using the HKHealthStore, and the results are processed in the resultsHandler block.
3.2. Handling Data
Once you have retrieved step count data, you can use it in your app as needed. For example, you could display the user’s daily step count on a dashboard or store it for further analysis.
4. Writing Health Data
In addition to reading data, you can also write health and fitness data to HealthKit. This is useful if your app collects data from external sensors or user input.
4.1. Creating a Sample
To write data to HealthKit, you need to create an HKQuantitySample or HKCategorySample object. For example, if your app wants to record the user’s heart rate, you can create a HKQuantitySample like this:
objective HKQuantityType *heartRateType = [HKQuantityType quantityTypeForIdentifier:HKQuantityTypeIdentifierHeartRate]; HKQuantity *heartRateQuantity = [HKQuantity quantityWithUnit:[HKUnit unitFromString:@"count/min"] doubleValue:80.0]; HKQuantitySample *heartRateSample = [HKQuantitySample quantitySampleWithType:heartRateType quantity:heartRateQuantity startDate:[NSDate date] endDate:[NSDate dateWithTimeIntervalSinceNow:60]];
In this example, we create a HKQuantitySample for heart rate data with a value of 80 beats per minute.
4.2. Saving Data
Once you’ve created the sample, you can save it to HealthKit using the HKHealthStore:
objective [healthStore saveObject:heartRateSample withCompletion:^(BOOL success, NSError *error) { if (success) { // Data saved successfully } else { // Handle error } }];
This code snippet saves the heart rate sample to HealthKit, and you can handle the success or error response accordingly.
5. Observing HealthKit Data Changes
HealthKit also supports data change observations, allowing your app to receive updates when new health and fitness data becomes available. This is useful for keeping your app’s data in sync with the user’s health data.
5.1. Setting Up an Observer
To observe data changes, you can use an HKObserverQuery. Here’s how to set up an observer for step count data:
objective HKSampleType *stepCountType = [HKSampleType quantityTypeForIdentifier:HKQuantityTypeIdentifierStepCount]; HKObserverQuery *query = [[HKObserverQuery alloc] initWithSampleType:stepCountType predicate:nil updateHandler:^(HKObserverQuery *query, HKObserverQueryCompletionHandler completionHandler, NSError *error) { if (!error) { // Handle data change here } else { // Handle error } }]; [healthStore executeQuery:query];
This code creates an observer query for step count data. When new step count data is available, the updateHandler block is called, allowing your app to respond to changes.
5.2. Handling Updates
In the updateHandler block, you can update your app’s user interface or perform any necessary data synchronization. This ensures that your app remains up to date with the user’s health and fitness data.
6. Best Practices and Considerations
Before you conclude your HealthKit integration, consider the following best practices and considerations:
6.1. User Education
Educate users about why your app needs access to their health data and how it will be used. Transparent communication builds trust and encourages users to grant permission.
6.2. Data Privacy
Respect user privacy by only requesting access to the specific data types your app needs. Avoid requesting unnecessary permissions.
6.3. Error Handling
Implement robust error handling to gracefully handle authorization denials, query failures, and other potential issues.
6.4. Testing
Thoroughly test your app’s HealthKit integration on different iOS devices to ensure a seamless user experience.
6.5. Documentation
Document your HealthKit integration thoroughly for future reference and collaboration with other developers.
Conclusion
Integrating HealthKit into your Objective-C iOS app empowers you to create health and fitness experiences that can transform users’ lives. Whether you want to build a step tracking app, a heart rate monitor, or a comprehensive health dashboard, HealthKit provides the tools you need to access and manage health data with ease.
In this guide, we’ve covered the essential steps to get started with HealthKit integration in Objective-C. From requesting authorization to reading and writing health data, you now have the knowledge and code samples to kickstart your health and fitness app development journey. So go ahead, leverage the power of Objective-C and HealthKit, and create apps that help users lead healthier lives. Your app could be the key to unlocking a world of well-being for countless iOS users!
Table of Contents
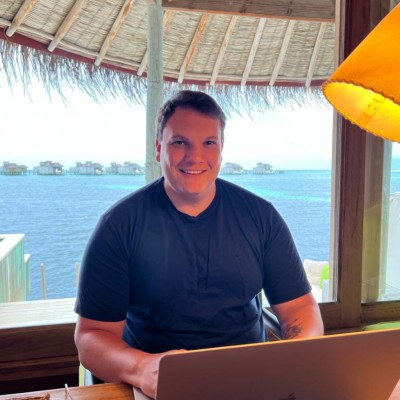
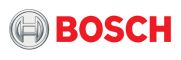