Objective-C and Core Bluetooth: Building Bluetooth Low Energy Apps
Bluetooth Low Energy (BLE) technology has revolutionized the way we connect and communicate with various devices, opening up a world of possibilities for developers. If you’re an iOS developer looking to build BLE apps using Objective-C, you’re in the right place. In this comprehensive guide, we’ll explore the essentials of BLE communication with Objective-C and Apple’s Core Bluetooth framework.
Table of Contents
1. Understanding Bluetooth Low Energy
1.1. What is Bluetooth Low Energy (BLE)?
Bluetooth Low Energy, often referred to as BLE or Bluetooth Smart, is a wireless communication technology designed for low-power, short-range communication between devices. Unlike classic Bluetooth, which is more power-hungry and suitable for data-intensive tasks like audio streaming, BLE is optimized for applications where energy efficiency is critical.
BLE is widely used in various industries, including healthcare (for fitness trackers and medical devices), home automation (for smart bulbs and thermostats), and asset tracking (for locating lost items). To work with BLE on iOS using Objective-C, you’ll need to leverage the Core Bluetooth framework.
2. Getting Started with Core Bluetooth
2.1. Setting Up Your Xcode Project
Before diving into Objective-C and Core Bluetooth, you need to set up your Xcode project. Follow these steps:
- Launch Xcode and create a new project or open an existing one.
- Ensure your project has the necessary permissions to access Bluetooth hardware. In your project settings, under the “Signing & Capabilities” tab, enable the “Bluetooth” background mode.
2.2. Importing Core Bluetooth
Now that your project is ready, you can start using Core Bluetooth by importing it into your Objective-C files:
objective #import <CoreBluetooth/CoreBluetooth.h>
This line allows you to access all the BLE-related classes and functions provided by Core Bluetooth.
3. Scanning for BLE Devices
3.1. Initializing the Central Manager
The first step in BLE communication is to initialize a central manager. The central manager is responsible for scanning for and connecting to peripheral devices (the devices you want to communicate with).
objective CBCentralManager *centralManager; centralManager = [[CBCentralManager alloc] initWithDelegate:self queue:nil];
In this code snippet, we create a CBCentralManager instance and set its delegate to self. The delegate methods will be called when the central manager discovers BLE devices.
3.2. Scanning for Devices
Once the central manager is initialized, you can start scanning for nearby BLE devices:
objective [centralManager scanForPeripheralsWithServices:nil options:nil];
The scanForPeripheralsWithServices:options: method starts scanning for devices advertising the specified services. In this case, we’re scanning for all devices since we’ve passed nil for both the services and options.
3.3. Handling Discovered Peripherals
To handle discovered peripherals, implement the following delegate method:
objective - (void)centralManager:(CBCentralManager *)central didDiscoverPeripheral:(CBPeripheral *)peripheral advertisementData:(NSDictionary<NSString *,id> *)advertisementData RSSI:(NSNumber *)RSSI { // Handle discovered peripheral here }
This method is called when the central manager discovers a BLE peripheral device. You can extract information about the peripheral from the advertisementData dictionary and perform actions like connecting to it or displaying it in your app’s UI.
4. Connecting to a BLE Peripheral
4.1. Initiating a Connection
To establish a connection with a peripheral, call the connect method on the central manager:
objective [centralManager connectPeripheral:peripheral options:nil];
In this code, peripheral is the CBPeripheral object representing the device you want to connect to. Again, we pass nil for options, but you can specify connection options if needed.
4.2. Handling Connection Events
Implement the following delegate methods to handle connection events:
objective - (void)centralManager:(CBCentralManager *)central didConnectPeripheral:(CBPeripheral *)peripheral { // Connection successful, now you can discover services and characteristics } - (void)centralManager:(CBCentralManager *)central didFailToConnectPeripheral:(CBPeripheral *)peripheral error:(NSError *)error { // Connection failed, handle the error }
The didConnectPeripheral method is called when the connection is successfully established, while the didFailToConnectPeripheral:error: method is called if the connection attempt fails.
5. Discovering Services and Characteristics
BLE devices typically expose services and characteristics that define their capabilities and data exchange mechanisms. To work with these services and characteristics, you need to discover them after connecting to a peripheral.
5.1. Discovering Services
To discover the services offered by a peripheral, use the following method:
objective [peripheral discoverServices:nil];
This method initiates the discovery process, and the results are reported through the delegate method:
objective - (void)peripheral:(CBPeripheral *)peripheral didDiscoverServices:(NSError *)error { // Handle discovered services here }
In the didDiscoverServices delegate method, you can access the discovered services from the peripheral.services array.
5.2. Discovering Characteristics
Once you have discovered the services, you can proceed to discover the characteristics within those services:
objective for (CBService *service in peripheral.services) { [peripheral discoverCharacteristics:nil forService:service]; }
This code iterates through the discovered services and initiates the discovery of characteristics for each service. The results are reported through the delegate method:
objective - (void)peripheral:(CBPeripheral *)peripheral didDiscoverCharacteristicsForService:(CBService *)service error:(NSError *)error { // Handle discovered characteristics here }
In the didDiscoverCharacteristicsForService delegate method, you can access the discovered characteristics from the service.characteristics array.
6. Reading and Writing Characteristics
6.1. Reading a Characteristic
To read the value of a characteristic, use the following method:
objective [peripheral readValueForCharacteristic:characteristic];
You need to specify the characteristic you want to read. The result is obtained through the delegate method:
objective - (void)peripheral:(CBPeripheral *)peripheral didUpdateValueForCharacteristic:(CBCharacteristic *)characteristic error:(NSError *)error { // Handle the updated characteristic value here }
In the didUpdateValueForCharacteristic delegate method, you can access the updated value of the characteristic from characteristic.value.
6.2. Writing to a Characteristic
To write a value to a characteristic, use the following method:
objective [peripheral writeValue:data forCharacteristic:characteristic type:CBCharacteristicWriteWithResponse];
In this code, data is an NSData object containing the data you want to write, and characteristic is the characteristic you want to write to. The type parameter specifies whether the write should be with or without a response.
The result of the write operation is reported through the delegate method:
objective - (void)peripheral:(CBPeripheral *)peripheral didWriteValueForCharacteristic:(CBCharacteristic *)characteristic error:(NSError *)error { // Handle the result of the write operation here }
7. Handling Notifications
BLE devices often use notifications to inform the central device (your iOS device) about changes in a characteristic’s value. To receive notifications for a characteristic, follow these steps:
Enable notifications for the characteristic:
objective [peripheral setNotifyValue:YES forCharacteristic:characteristic];
Implement the following delegate method to handle notifications:
objective - (void)peripheral:(CBPeripheral *)peripheral didUpdateNotificationStateForCharacteristic:(CBCharacteristic *)characteristic error:(NSError *)error { // Handle notification state changes here }
Receive updated values through the didUpdateValueForCharacteristic delegate method, as discussed earlier.
8. Disconnecting from a Peripheral
When you’re done communicating with a peripheral, you should disconnect from it to conserve power and resources. To disconnect, simply call the cancelPeripheralConnection method:
objective [centralManager cancelPeripheralConnection:peripheral];
This method will terminate the connection and trigger the centralManager:didDisconnectPeripheral:error: delegate method:
objective - (void)centralManager:(CBCentralManager *)central didDisconnectPeripheral:(CBPeripheral *)peripheral error:(NSError *)error { // Handle disconnection here }
9. Error Handling and Best Practices
Building BLE apps with Objective-C and Core Bluetooth is a powerful capability, but it comes with its challenges. Here are some best practices and tips for error handling:
- Check for BLE Availability: Before initializing the central manager, check if BLE is available on the device by using the CBCentralManagerState property.
objective if (centralManager.state == CBCentralManagerStatePoweredOn) { // BLE is available, proceed with initialization } else { // Handle the case where BLE is not available }
- Handle Background Execution: If your app needs to work with BLE in the background, ensure you have the necessary background modes enabled in your project settings and handle background tasks appropriately.
- Implement Reconnection Logic: BLE connections can be fragile, so consider implementing reconnection logic in case of disconnections.
- Threading and Concurrency: Pay attention to threading and concurrency when interacting with Core Bluetooth. Most Core Bluetooth methods should be called on the main thread to ensure proper synchronization.
- Bluetooth Pairing: Understand the concept of pairing and security in BLE. Some devices may require pairing before communication.
Conclusion
Objective-C remains a valuable tool for iOS development, and when combined with Core Bluetooth, it opens up exciting possibilities for creating Bluetooth Low Energy apps. With the fundamentals of scanning, connecting, discovering services and characteristics, and handling notifications, you can start building robust BLE applications that connect and interact with a wide range of devices.
Remember that BLE development can be complex, especially when dealing with multiple peripherals or advanced use cases. Continuously test your apps on various devices and scenarios to ensure a seamless user experience. As BLE technology continues to evolve, staying up-to-date with the latest advancements in the iOS ecosystem is crucial for building cutting-edge Bluetooth applications. Happy coding!
Table of Contents
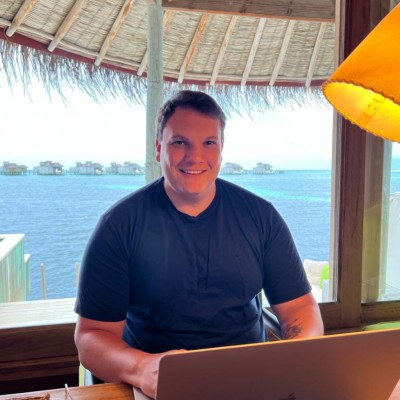
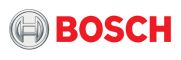