Objective-C Blocks: Simplifying Asynchronous Programming in iOS
Asynchronous programming is a fundamental aspect of iOS app development, allowing apps to remain responsive and perform tasks in the background. While it’s a powerful concept, writing asynchronous code can be complex and error-prone. Objective-C Blocks come to the rescue, simplifying asynchronous programming in iOS. In this blog post, we’ll explore what Objective-C Blocks are, how they work, and how they make asynchronous programming more efficient and readable.
1. Understanding Asynchronous Programming
Before diving into Objective-C Blocks, let’s briefly understand what asynchronous programming is and why it’s crucial for iOS app development.
1.1. What is Asynchronous Programming?
In iOS development, tasks like network requests, file I/O, and animations can be time-consuming. If we execute these tasks synchronously on the main thread, it will freeze the user interface, resulting in a poor user experience. Asynchronous programming allows us to perform these tasks in the background without blocking the main thread, ensuring the app remains responsive.
1.2. Why Asynchronous Programming?
Asynchronous programming offers several advantages:
- Responsiveness: Keeps the app’s user interface responsive by running time-consuming tasks in the background.
- Parallelism: Executes multiple tasks concurrently, making the best use of multi-core processors.
- Improved User Experience: Prevents UI freezes, providing a smoother user experience.
- Efficiency: Optimizes resource usage, as background tasks can wait for I/O operations without wasting CPU cycles.
Now that we’ve established the importance of asynchronous programming, let’s explore how Objective-C Blocks simplify the implementation of asynchronous code.
2. Introduction to Objective-C Blocks
Objective-C Blocks, introduced in iOS 4.0, are a powerful feature for working with functions and blocks of code as first-class objects. They encapsulate a segment of code and allow you to pass it around like a variable. Blocks enable the creation of self-contained, reusable pieces of code, making asynchronous programming more manageable.
2.1. Anatomy of an Objective-C Block
A typical Objective-C Block has the following structure:
objective returnType (^blockName)(parameterType) = ^(parameterType parameters) { // Code to be executed asynchronously };
- returnType: The type of value the block returns.
- blockName: The name of the block variable.
- parameterType: The type of input parameters (if any) the block takes.
- parameters: The actual input parameters.
Let’s break down the components of an Objective-C Block with a practical example.
2.2. Practical Example: A Simple Block
objective // Define a block that takes two integers and returns their sum int (^addBlock)(int, int) = ^(int a, int b) { return a + b; }; // Call the block int result = addBlock(3, 4); NSLog(@"Result: %d", result); // Output: Result: 7
In this example, we define a block named addBlock that takes two integers and returns their sum. We can then call this block like a regular function, passing it the required parameters.
2.3. Using Blocks for Asynchronous Programming
Objective-C Blocks are particularly useful when working with asynchronous tasks. They allow you to define a piece of code that will be executed at a later time, making it easy to manage callbacks, delegates, and completion handlers. Here’s how you can use blocks for asynchronous programming:
1. Defining an Asynchronous Block:
objective void (^downloadTask)(void) = ^{ // Code to download data asynchronously };
2. Executing the Asynchronous Block:
objective dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{ downloadTask(); // Execute the asynchronous block });
3. Handling Completion:
objective // Assume this method is called when the download is complete - (void)downloadComplete { // Perform actions after download completion } objective // In your asynchronous block, call the completion handler downloadTask = ^{ // Code to download data asynchronously // Once done, call the completion handler [self downloadComplete]; };
By using Objective-C Blocks, you can encapsulate asynchronous code, making it more modular and easier to understand. Additionally, you can pass blocks as arguments to functions or methods, allowing for flexible and customizable behavior.
3. Leveraging Blocks in iOS Development
Now that we’ve covered the basics of Objective-C Blocks, let’s explore some common scenarios where blocks simplify asynchronous programming in iOS development.
3.1. Networking Requests
Making network requests is a common asynchronous task in iOS apps. With Objective-C Blocks, you can define a network request block that encapsulates the request logic and callback handling. Here’s an example using NSURLSession:
objective - (void)fetchDataFromServer { NSURL *url = [NSURL URLWithString:@"https://api.example.com/data"]; void (^networkRequestBlock)(NSData *, NSError *) = ^(NSData *data, NSError *error) { if (error) { // Handle error NSLog(@"Network request failed with error: %@", error); } else { // Process data NSLog(@"Data received: %@", data); } }; NSURLSessionDataTask *dataTask = [[NSURLSession sharedSession] dataTaskWithURL:url completionHandler:networkRequestBlock]; [dataTask resume]; }
By using a block for the network request’s completion handler, you keep the code organized and make it clear how to handle the response or errors.
3.2. Animation
Animating UI elements is another scenario where blocks shine. You can use UIView animations with blocks to create smooth and interactive user interfaces.
objective [UIView animateWithDuration:0.5 animations:^{ // Animation code view.frame = CGRectMake(100, 100, 200, 200); } completion:^(BOOL finished) { // Completion code NSLog(@"Animation finished"); }];
The animation block defines the animation’s properties, such as duration and final state, while the completion block allows you to execute code after the animation finishes.
3.3. Grand Central Dispatch (GCD)
Grand Central Dispatch is a powerful framework for managing concurrent code execution. Blocks are a natural fit for GCD, making it easier to create and manage dispatch queues.
objective dispatch_queue_t customQueue = dispatch_queue_create("com.example.myqueue", NULL); dispatch_async(customQueue, ^{ // Perform work asynchronously on the custom queue });
Here, the dispatch_async function takes a block as its parameter, specifying the code to execute asynchronously on the custom queue.
3.4. View Controller Transitions
Transitioning between view controllers is a core part of iOS app navigation. Blocks simplify the process by allowing you to define the transition animations and completion handlers cleanly.
objective [self presentViewController:nextViewController animated:YES completion:^{ // Code to execute after the presentation animation finishes }];
The completion block specifies actions to take after the view controller presentation animation completes.
4. Best Practices for Using Objective-C Blocks
To effectively use Objective-C Blocks in your iOS development, consider the following best practices:
4.1. Keep Blocks Short and Focused
Blocks should encapsulate a specific piece of functionality. Avoid writing long, complex blocks that try to do too much. Short and focused blocks are easier to read, understand, and maintain.
4.2. Handle Retain Cycles
When capturing objects inside a block, be mindful of retain cycles that can lead to memory leaks. Use __weak or __block qualifiers to break strong reference cycles when necessary.
4.3. Use Block-Based Enumeration
Objective-C provides block-based enumeration methods for collections like arrays and dictionaries. These methods offer concise and efficient ways to iterate over elements.
objective NSArray *numbers = @[@1, @2, @3, @4, @5]; [numbers enumerateObjectsUsingBlock:^(NSNumber *number, NSUInteger idx, BOOL *stop) { // Block-based enumeration code NSLog(@"Number at index %lu: %@", idx, number); }];
4.4. Understand Capture Semantics
Blocks capture variables differently depending on their storage duration and type. Be aware of variable capture semantics and potential side effects.
4.5. Document Block Signatures
Provide clear documentation for the expected parameters and return types of your blocks. This helps other developers understand how to use your code effectively.
Conclusion
Objective-C Blocks are a valuable tool for simplifying asynchronous programming in iOS development. They enable you to encapsulate pieces of code, making your codebase more modular and readable. By leveraging blocks, you can efficiently handle asynchronous tasks, network requests, animations, and more. However, like any powerful feature, it’s essential to use blocks judiciously and follow best practices to ensure clean and maintainable code in your iOS apps.
In summary, embrace Objective-C Blocks in your iOS development journey, and you’ll find yourself writing more elegant and efficient asynchronous code while improving the overall user experience of your apps.
Table of Contents
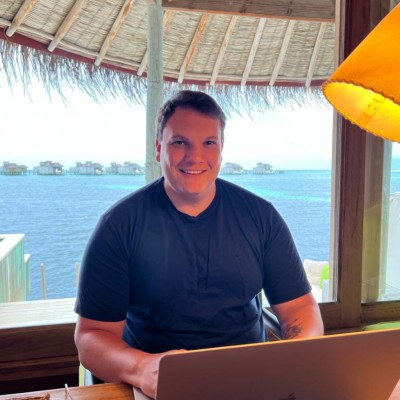
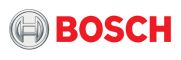