Objective-C Categories: Extending Classes in iOS Development
In the realm of iOS app development, the Objective-C programming language has long been a cornerstone. One of its powerful features is the ability to extend existing classes using a mechanism known as “categories.” Categories allow developers to add new methods to existing classes without the need to subclass or modify the original source code. This blog post will delve into the world of Objective-C categories, explaining how they work and providing practical examples of how to use them to extend classes in iOS development.
1. Understanding Objective-C Categories
1.1 What Are Objective-C Categories?
Objective-C categories are a way to extend the functionality of a class without altering its original implementation. This powerful feature enables developers to add new methods to a class, making it more versatile and capable of performing additional tasks. Categories are particularly useful when working with classes from third-party libraries or system frameworks, as they allow you to enhance these classes without modifying their source code.
1.2 Key Benefits of Using Categories
Modularity: Categories promote a modular approach to code. You can organize related methods into separate categories, making your codebase more organized and maintainable.
- Reusability: You can reuse categories across multiple projects, enhancing the functionality of different classes without duplicating code.
- Third-party Libraries: When working with third-party libraries or system classes, categories offer a non-intrusive way to add missing functionality or fix issues.
- Runtime Flexibility: Categories are applied at runtime, which means you can extend classes even if you don’t have access to their source code during compilation.
2. Creating and Using Objective-C Categories
2.1 Creating a Category
To create a category, you need to implement a new Objective-C file with the .m extension and define your methods in it. Here’s a step-by-step guide on creating a category:
- Create a New Objective-C File: In Xcode, go to File > New > File… and select “Objective-C File.” Name it something like MyClass+CategoryName.m, where MyClass is the name of the class you want to extend, and CategoryName is the name of your category.
- Import the Header File: At the top of your category implementation file, import the header file of the class you’re extending:
objective #import "MyClass.h"
Define Your Methods: Implement your additional methods within the category file:
objective #import "MyClass+CategoryName.h" @implementation MyClass (CategoryName) - (void)newMethod { // Your implementation here } @end
2.2 Importing Categories
To use a category in your project, you only need to import the category’s header file where you want to use the additional methods. Here’s how you can import and use a category in your code:
objective #import "MyClass+CategoryName.h" // ... MyClass *object = [[MyClass alloc] init]; [object newMethod]; // Call the method from the category
2.3 Overriding Existing Methods
Categories can also be used to override existing methods in a class. However, caution is advised when doing this, as it can lead to unexpected behavior or conflicts with other categories. If you need to override a method, it’s often better to subclass the class instead.
3. Practical Examples of Objective-C Categories
Now that you understand the basics of categories, let’s explore some practical examples of how they can be used in iOS development.
Example 1: Adding Validation to UITextField
Suppose you want to add validation functionality to UITextField to check if the input contains only numeric characters. Here’s how you can do it using a category:
objective #import <UIKit/UIKit.h> @interface UITextField (NumericValidation) - (BOOL)isValidNumber; @end objective #import "UITextField+NumericValidation.h" @implementation UITextField (NumericValidation) - (BOOL)isValidNumber { NSCharacterSet *numbersOnly = [NSCharacterSet decimalDigitCharacterSet]; NSCharacterSet *inputCharacterSet = [NSCharacterSet characterSetWithCharactersInString:self.text]; return [numbersOnly isSupersetOfSet:inputCharacterSet]; } @end
Now, any UITextField in your project can use the isValidNumber method to check if its text input contains only numeric characters.
objective UITextField *textField = [[UITextField alloc] initWithFrame:CGRectMake(0, 0, 200, 30)]; textField.text = @"12345"; BOOL isValid = [textField isValidNumber]; // Returns YES
Example 2: UIColor Convenience Methods
You can also use categories to create convenience methods for commonly used functionalities. For instance, you can extend UIColor to create colors with custom hex values easily:
objective #import <UIKit/UIKit.h> @interface UIColor (CustomColors) + (UIColor *)colorWithHex:(NSString *)hexString; @end objective #import "UIColor+CustomColors.h" @implementation UIColor (CustomColors) + (UIColor *)colorWithHex:(NSString *)hexString { NSScanner *scanner = [NSScanner scannerWithString:hexString]; unsigned int hexValue; [scanner scanHexInt:&hexValue]; CGFloat red = ((hexValue >> 16) & 0xFF) / 255.0; CGFloat green = ((hexValue >> 8) & 0xFF) / 255.0; CGFloat blue = (hexValue & 0xFF) / 255.0; return [UIColor colorWithRed:red green:green blue:blue alpha:1.0]; } @end
Now, you can create UIColor objects using hex values:
objective UIColor *customColor = [UIColor colorWithHex:@"#FF5733"];
4. Best Practices and Considerations
While Objective-C categories offer great flexibility and power, it’s essential to follow some best practices and consider potential pitfalls:
4.1 Prefix Your Categories
To avoid naming conflicts with other libraries or future updates, always prefix your category names. For example, instead of naming your category “Utils,” use something like “MyApp_Utils.”
4.2 Use Categories Sparingly
Reserve the use of categories for cases where subclassing or delegation is not a suitable solution. Overusing categories can make your codebase less predictable and harder to maintain.
4.3 Be Cautious with Method Overrides
As mentioned earlier, overriding methods in categories should be approached with caution to prevent unexpected behavior or conflicts.
4.4 Document Your Categories
Provide clear documentation for your categories, explaining their purpose and usage. This helps other developers understand how to use your extensions effectively.
4.5 Avoid Adding Instance Variables
Categories cannot add instance variables to a class, so be mindful not to attempt this. If you need to store additional data, consider using associated objects or subclassing.
Conclusion
Objective-C categories are a valuable tool in iOS development, allowing you to extend the functionality of existing classes without modifying their source code. This modularity and flexibility can make your codebase more organized, maintainable, and efficient. By following best practices and considering potential pitfalls, you can harness the power of categories to enhance your iOS apps and streamline your development process.
In this blog post, we’ve covered the basics of Objective-C categories, demonstrated how to create and use them, and provided practical examples of their application in iOS development. Armed with this knowledge, you can start using categories to improve and extend classes in your own iOS projects.
Table of Contents
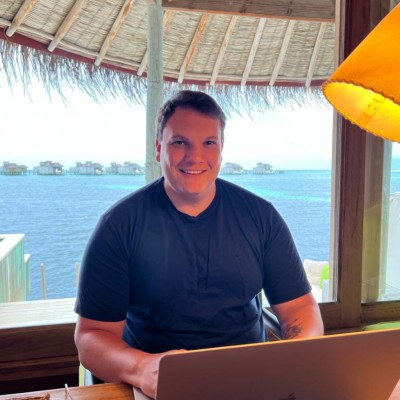
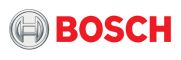