Objective-C Classes and Objects: Building Blocks of iOS Apps
Objective-C is a powerful programming language that forms the backbone of iOS app development. At the heart of Objective-C lies the concept of classes and objects, which serve as the fundamental building blocks for creating robust and scalable iOS applications. In this blog post, we will dive deep into the world of Objective-C classes and objects, understanding their structure, properties, methods, and their importance in the iOS app development process. By the end, you will have a solid foundation to build upon as you embark on your journey to create innovative and feature-rich iOS applications.
1. What are Classes and Objects?
In Object-Oriented Programming (OOP), a class is a blueprint or template for creating objects. An object, on the other hand, is an instance of a class. Think of a class as a blueprint for a house, and an object as an actual house built based on that blueprint. Each object created from a class has its own set of properties and can perform specific actions.
2. Structure of an Objective-C Class
An Objective-C class typically consists of two files: a header file (with a .h extension) and an implementation file (with a .m extension). The header file contains the class interface, which declares the public properties and methods, while the implementation file contains the actual code for those methods.
3. Declaring and Defining Classes
To declare a class, you need to define its name, superclass (if any), and the properties and methods it will have. Here’s an example of declaring a simple Objective-C class:
objective @interface MyClass : NSObject @property (nonatomic, strong) NSString *name; - (void)sayHello; @end
In this example, we declare a class named “MyClass” that inherits from the “NSObject” class. It has a single property named “name” of type NSString and a method named “sayHello” that takes no parameters and returns void.
4. Properties: State of an Object
Properties represent the state or characteristics of an object. They encapsulate data and provide an interface for accessing and modifying that data. Objective-C introduces the concept of properties to simplify the syntax for creating and managing instance variables.
4.1 Instance Variables
Instance variables hold the actual data of a property. They are declared within the class implementation and are typically prefixed with an underscore (_). You can access and modify these variables using property accessors.
4.2 Accessing Properties
Objective-C provides the dot notation for accessing and modifying properties of an object. For example:
objective MyClass *myObject = [[MyClass alloc] init]; myObject.name = @"John Doe"; NSString *objectName = myObject.name;
In this example, we create an instance of MyClass called myObject and assign a value to its name property using the dot notation. We can also retrieve the value of the name property in the same way.
4.3 Synthesized Accessors
In modern Objective-C, properties are typically synthesized automatically using the @synthesize directive. This means that the compiler generates the accessor methods for you, saving you from writing boilerplate code. However, you can still manually implement your own accessors if you need custom behavior.
5. Methods: Behavior of an Object
Methods define the behavior or actions that an object can perform. They encapsulate reusable blocks of code that can be invoked to manipulate the object’s state or perform specific tasks.
5.1 Declaring and Defining Methods
Similar to properties, methods are declared in the class interface and defined in the implementation file. Here’s an example of declaring and defining a method in Objective-C:
objective - (void)sayHello { NSLog(@"Hello!"); }
In this example, we declare a method named “sayHello” that takes no parameters and returns void. The implementation of the method is defined within the implementation file, and in this case, it simply logs “Hello!” to the console.
5.2 Method Parameters and Return Types
Methods can have parameters and return types, allowing them to accept inputs and provide outputs. Parameters are specified within the parentheses after the method name, and the return type is specified before the method declaration.
objective - (NSInteger)addNumber:(NSInteger)a toNumber:(NSInteger)b { return a + b; }
In this example, we declare a method named “addNumber:toNumber:” that takes two NSInteger parameters, ‘a’ and ‘b’, and returns an NSInteger. The method adds the two numbers together and returns the result.
5.3 Invoking Methods
To invoke a method on an object, you use the square bracket notation. Here’s an example of invoking the “sayHello” method on an instance of MyClass:
objective MyClass *myObject = [[MyClass alloc] init]; [myObject sayHello];
In this example, we create an instance of MyClass called myObject and invoke the “sayHello” method on it using the square bracket notation.
6. Code Samples: Putting It All Together
Let’s put everything we’ve learned into practice by creating a simple class, defining properties and methods, and instantiating objects:
6.1 Creating a Class
objective @interface Person : NSObject @property (nonatomic, strong) NSString *name; @property (nonatomic) NSInteger age; - (void)sayHello; @end
6.2 Defining Properties and Methods
objective @implementation Person - (void)sayHello { NSLog(@"Hello, my name is %@ and I'm %ld years old.", self.name, (long)self.age); } @end
6.3 Instantiating Objects
objective Person *person1 = [[Person alloc] init]; person1.name = @"John Doe"; person1.age = 30; Person *person2 = [[Person alloc] init]; person2.name = @"Jane Smith"; person2.age = 25;
6.4 Accessing Properties and Invoking Methods
objective NSLog(@"%@ is %ld years old.", person1.name, (long)person1.age); [person2 sayHello];
Output:
vbnet John Doe is 30 years old. Hello, my name is Jane Smith and I'm 25 years old.
Conclusion
In this blog post, we’ve explored the fundamental concepts of Objective-C classes and objects, which form the core of iOS app development. We’ve learned about their structure, properties, methods, and how to interact with them through code samples. Understanding classes and objects is essential for creating robust and scalable iOS applications. Armed with this knowledge, you are now ready to take the next steps on your journey to becoming a proficient iOS developer. Happy coding!
Table of Contents
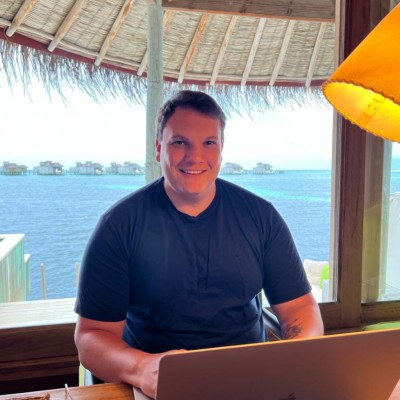
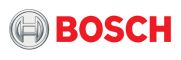