Objective-C Core Data: Persistent Storage in iOS Apps
In the world of iOS app development, efficient data management and storage are paramount. Enter Core Data, a powerful framework that simplifies the task of managing the model layer of your app and provides seamless data persistence. While Swift has gained popularity in recent years, Objective-C remains a viable language for iOS development. In this blog, we’ll delve into Objective-C Core Data, exploring its features, benefits, and providing you with practical code samples to get you started on the path to efficient data storage in iOS apps.
Table of Contents
1. Why Use Core Data in iOS Apps?
Before we dive into Objective-C Core Data, let’s take a moment to understand why it’s a valuable tool for iOS developers.
1.1. Data Persistence
In iOS, data persistence is crucial to store user preferences, app settings, and any data that should survive app restarts. Core Data offers a robust solution for this, allowing you to save and retrieve data easily.
1.2. Efficient Data Management
Managing complex data structures can be challenging. Core Data simplifies this by providing a high-level object-oriented API for data management, making it easier to work with data models.
1.3. Undo and Redo Support
Core Data comes with built-in support for undo and redo operations. This is incredibly useful when dealing with user actions that require data changes, ensuring a smooth user experience.
1.4. Relationship Management
In many apps, data is interconnected through relationships. Core Data simplifies the management of these relationships, allowing you to define them in your data model.
1.5. Data Validation
Data integrity is crucial, and Core Data helps you enforce rules and validate data before it’s saved, reducing the chances of data corruption.
Now that we’ve established the benefits of Core Data, let’s get hands-on with Objective-C code.
2. Setting Up a Core Data Project in Objective-C
To get started with Objective-C Core Data, follow these steps to set up a new project:
Step 1: Create a New Xcode Project
Open Xcode and select “Create a new Xcode project.” Choose the “Single View App” template and name your project.
Step 2: Enable Core Data
In the project settings, select your target, and under the “Capabilities” tab, enable “Use Core Data.” This action will generate the necessary files and configurations.
Step 3: Create a Data Model
In the Xcode project navigator, locate the .xcdatamodeld file and double-click to open it. Here, you can define your data model. Click the “+” button to add entities, attributes, and relationships.
With the project set up, let’s dive into some code samples.
3. Working with Core Data in Objective-C
3.1. Creating a Managed Object Subclass
When you define your data model, Core Data generates managed object subclasses for you. However, it’s often more convenient to create custom subclasses that represent your data entities.
objective #import <CoreData/CoreData.h> @interface Person : NSManagedObject @property (nonatomic, strong) NSString *name; @property (nonatomic, strong) NSNumber *age; @end @implementation Person @dynamic name; @dynamic age; @end
In the code above, we’ve created a Person class that inherits from NSManagedObject, representing an entity in our data model. We’ve also defined two properties, name and age, which correspond to attributes in our data model.
3.2. Initializing Core Data Stack
To work with Core Data, you need to set up the Core Data stack. This involves initializing the NSManagedObjectContext, NSManagedObjectModel, NSPersistentStoreCoordinator, and the NSPersistentStore.
objective // Initialize the managed object context NSManagedObjectContext *managedObjectContext = [[NSManagedObjectContext alloc] initWithConcurrencyType:NSMainQueueConcurrencyType]; // Initialize the managed object model NSURL *modelURL = [[NSBundle mainBundle] URLForResource:@"YourDataModel" withExtension:@"momd"]; NSManagedObjectModel *managedObjectModel = [[NSManagedObjectModel alloc] initWithContentsOfURL:modelURL]; // Initialize the persistent store coordinator NSPersistentStoreCoordinator *persistentStoreCoordinator = [[NSPersistentStoreCoordinator alloc] initWithManagedObjectModel:managedObjectModel]; // Set up the persistent store NSURL *storeURL = [[self applicationDocumentsDirectory] URLByAppendingPathComponent:@"YourApp.sqlite"]; NSError *error = nil; if (![persistentStoreCoordinator addPersistentStoreWithType:NSSQLiteStoreType configuration:nil URL:storeURL options:nil error:&error]) { NSLog(@"Unresolved error %@, %@", error, [error userInfo]); abort(); } // Link the persistent store coordinator with the managed object context [managedObjectContext setPersistentStoreCoordinator:persistentStoreCoordinator];
In the code above, replace “YourDataModel” with the name of your data model, and “YourApp.sqlite” with your preferred SQLite database filename.
3.3. Saving Data
Now, let’s see how to save data to Core Data:
objective // Create a new person object Person *newPerson = [NSEntityDescription insertNewObjectForEntityForName:@"Person" inManagedObjectContext:managedObjectContext]; newPerson.name = @"John"; newPerson.age = @30; // Save the changes NSError *error = nil; if (![managedObjectContext save:&error]) { NSLog(@"Error saving context: %@", error); }
In this code snippet, we’ve created a new Person object, set its properties, and saved it to the Core Data store using the managed object context.
3.4. Fetching Data
Fetching data from Core Data is straightforward. Here’s how you can retrieve all Person objects:
objective NSFetchRequest *fetchRequest = [NSFetchRequest fetchRequestWithEntityName:@"Person"]; NSError *error = nil; NSArray *people = [managedObjectContext executeFetchRequest:fetchRequest error:&error]; if (error) { NSLog(@"Error fetching data: %@", error); } else { for (Person *person in people) { NSLog(@"Name: %@, Age: %@", person.name, person.age); } }
3.5. Updating and Deleting Data
Updating and deleting data is as simple as changing the properties of a managed object and saving the context. To delete an object:
objective [managedObjectContext deleteObject:personToDelete]; NSError *error = nil; if (![managedObjectContext save:&error]) { NSLog(@"Error deleting object: %@", error); }
3.6. Error Handling
Error handling is essential when working with Core Data. Always check for errors when performing operations like saving, fetching, updating, or deleting data.
Conclusion
Objective-C Core Data provides a powerful and efficient way to manage and persist data in iOS apps. It simplifies data management, enforces data integrity, and enhances the overall user experience by providing features like undo and redo support.
In this blog, we’ve covered the basics of setting up a Core Data project in Objective-C and working with managed object subclasses. We’ve also demonstrated how to initialize the Core Data stack, save, fetch, update, and delete data, along with error handling.
As you delve deeper into iOS development, mastering Core Data will become an invaluable skill. It opens up a world of possibilities for creating feature-rich, data-driven apps that provide a seamless and enjoyable user experience.
So, whether you’re a seasoned Objective-C developer or just starting your iOS journey, Core Data is a tool you should definitely add to your toolkit. Happy coding!
Table of Contents
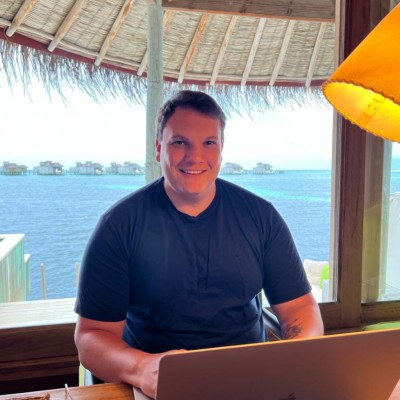
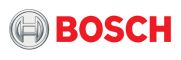