Objective-C and Core Location: Building Location-Aware iOS Apps
In today’s interconnected world, location-aware apps have become an integral part of our daily lives. Whether it’s finding the nearest coffee shop, tracking your morning run, or geotagging photos, location data plays a crucial role. Objective-C, the programming language for iOS development, along with Core Location framework, provides the tools needed to build robust location-aware applications. In this comprehensive guide, we will explore the fundamentals of Objective-C and Core Location, and how to harness their power to create compelling iOS apps.
Table of Contents
1. Introduction to Core Location
1.1. Understanding Core Location
Core Location is a powerful framework provided by Apple for iOS and macOS development. It allows you to determine the geographic location of a device and provides information about that location, such as latitude, longitude, altitude, and more. Core Location is not just limited to GPS; it can also use Wi-Fi and cellular networks to provide location data.
1.2. Importing Core Location Framework
To start using Core Location in your Objective-C project, you need to import the Core Location framework. You can do this by adding the following line to your source file:
objective #import <CoreLocation/CoreLocation.h>
1.3. Requesting User Authorization
Before you can access the user’s location, you must request permission. This is crucial to respect the user’s privacy. To request permission, add the following code to your app:
objective CLLocationManager *locationManager = [[CLLocationManager alloc] init]; [locationManager requestWhenInUseAuthorization];
2. Getting Location Updates
2.1. Single Location Updates
To obtain a single location update, you can use the following code:
objective CLLocationManager *locationManager = [[CLLocationManager alloc] init]; locationManager.delegate = self; // Set your delegate to receive updates [locationManager startUpdatingLocation];
Implement the CLLocationManagerDelegate methods to receive location updates:
objective - (void)locationManager:(CLLocationManager *)manager didUpdateLocations:(NSArray<CLLocation *> *)locations { CLLocation *location = [locations lastObject]; NSLog(@"Latitude: %f, Longitude: %f", location.coordinate.latitude, location.coordinate.longitude); }
2.2. Continuous Location Updates
For continuous updates, you can start location updates and set the desired accuracy:
objective [locationManager startUpdatingLocation]; locationManager.desiredAccuracy = kCLLocationAccuracyNearestTenMeters;
2.3. Handling Location Manager Events
Core Location provides various events that you can handle to manage location updates. These events include authorization changes, location updates, and errors. Be sure to implement the necessary delegate methods to handle these events gracefully.
objective - (void)locationManager:(CLLocationManager *)manager didChangeAuthorizationStatus:(CLAuthorizationStatus)status { // Handle authorization changes } - (void)locationManager:(CLLocationManager *)manager didFailWithError:(NSError *)error { // Handle location manager errors }
3. Geofencing and Region Monitoring
3.1. What is Geofencing?
Geofencing is a location-based service that allows you to define virtual boundaries or regions on a map and monitor when a device enters or exits these regions. This is incredibly useful for creating location-aware apps that trigger actions based on a user’s proximity to a specific location.
3.2. Monitoring Regions
To monitor a region, you need to create an instance of CLCircularRegion and start monitoring it:
objective CLLocationCoordinate2D centerCoordinate = CLLocationCoordinate2DMake(latitude, longitude); CLCircularRegion *region = [[CLCircularRegion alloc] initWithCenter:centerCoordinate radius:radius identifier:@"MyGeofence"]; [locationManager startMonitoringForRegion:region];
3.3. Responding to Region Events
When a device enters or exits a monitored region, the delegate method locationManager:didEnterRegion: and locationManager:didExitRegion: will be called. You can implement these methods to trigger actions in your app, such as sending notifications or updating the user interface.
objective - (void)locationManager:(CLLocationManager *)manager didEnterRegion:(CLRegion *)region { // Handle region entry } - (void)locationManager:(CLLocationManager *)manager didExitRegion:(CLRegion *)region { // Handle region exit }
4. Location Data and Services
4.1. Retrieving Location Data
Core Location provides various ways to retrieve location data. You can get the device’s current location, track significant location changes, and even fetch location updates in the background.
objective // Get the device's current location CLLocation *currentLocation = locationManager.location; // Track significant location changes [locationManager startMonitoringSignificantLocationChanges];
4.2. Reverse Geocoding
Reverse geocoding is the process of converting a latitude and longitude coordinate into a human-readable address. Core Location makes this task straightforward:
objective CLLocation *location = [[CLLocation alloc] initWithLatitude:latitude longitude:longitude]; [geocoder reverseGeocodeLocation:location completionHandler:^(NSArray<CLPlacemark *> * _Nullable placemarks, NSError * _Nullable error) { if (error == nil && [placemarks count] > 0) { CLPlacemark *placemark = [placemarks firstObject]; NSString *address = [NSString stringWithFormat:@"%@, %@", placemark.locality, placemark.country]; NSLog(@"Address: %@", address); } }];
4.3. Location-Based Services
Location data can enhance the functionality of your app in various ways. You can integrate maps, provide location-specific recommendations, or create location-based social features. Make sure to explore the possibilities that location data offers for your app’s unique requirements.
5. Practical Examples
5.1. Building a Location-Based Weather App
Let’s put our knowledge of Objective-C and Core Location into practice by creating a simple location-based weather app. This app will fetch the user’s current location and display the weather information for that location using a weather API.
objective // Fetching the current location CLLocation *currentLocation = locationManager.location; // Use the location to fetch weather data from a weather API
5.2. Creating a Geofencing Reminder App
Another practical example is building a geofencing reminder app. This app allows users to set reminders associated with specific locations. When the user enters or exits a predefined region, the app sends a notification to remind them of the task.
objective // Create geofence regions and monitor them CLCircularRegion *reminderRegion = [[CLCircularRegion alloc] initWithCenter:centerCoordinate radius:radius identifier:@"ReminderRegion"]; [locationManager startMonitoringForRegion:reminderRegion]; // Handle region events to trigger reminders
6. Best Practices and Optimization
6.1. Battery Efficiency
Continuous location updates can drain the device’s battery quickly. To mitigate this, use the lowest acceptable accuracy level for your app’s functionality and minimize the frequency of location updates.
6.2. Error Handling
Always handle errors gracefully when working with Core Location. Common errors include lack of authorization, device’s location services being disabled, or loss of GPS signal.
6.3. User Experience Considerations
Respect the user’s privacy by requesting location access only when necessary and providing clear explanations of why you need their location data. Additionally, consider offering an option to disable location services within your app.
Conclusion
Objective-C, paired with Core Location, provides a robust foundation for building location-aware iOS apps. Whether you’re developing a navigation app, a geofencing reminder, or a location-based game, the knowledge and skills you’ve gained from this guide will be invaluable. Remember to always prioritize user privacy and efficient use of device resources when working with location data. With the right approach and creativity, you can create compelling and user-friendly location-aware applications that cater to the needs of your target audience.
Table of Contents
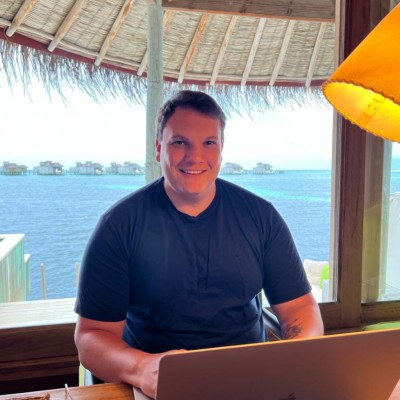
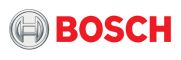