Objective-C Debugging: Tips and Techniques for Effective Troubleshooting
Debugging is an integral part of the software development process. Whether you are a seasoned developer or just starting your journey with Objective-C, understanding how to effectively troubleshoot your code is crucial. Objective-C, being a dynamic and powerful language, comes with its unique set of challenges when it comes to debugging. In this blog post, we will explore various tips and techniques to help you become a proficient Objective-C debugger.
1. Understanding the Importance of Debugging
Debugging is the process of identifying and fixing errors or bugs in your code. It plays a critical role in software development because, no matter how skilled a programmer you are, bugs are inevitable. Debugging not only helps you identify and resolve issues but also improves your understanding of the codebase.
2. Essential Debugging Tools
2.1 Xcode Debugger
Xcode, Apple’s integrated development environment, provides a powerful and user-friendly debugger. Here’s how you can use it to debug your Objective-C applications:
objective - (IBAction)debugButtonPressed:(id)sender { int x = 10; int y = 0; int result = x / y; // This will trigger an exception }
- Set breakpoints: Click on the line number where you want to pause execution. You can also set conditional breakpoints.
- Inspect variables: While debugging, you can hover over variables to see their current values.
- Step through code: Use the step over, step into, and step out buttons to navigate through your code step by step.
- Debug console: The debug console allows you to interact with your code in real-time and execute LLDB commands.
2.2 LLDB Command-Line Debugger
LLDB is the low-level debugger used by Xcode. If you prefer a command-line interface, LLDB is a great choice. Here’s a simple LLDB session:
shell $ lldb (lldb) target create "YourApp.app" (lldb) breakpoint set --file main.m --line 10 (lldb) run (lldb) po myObject
LLDB allows you to set breakpoints, inspect variables, and execute commands just like the Xcode debugger.
3. Debugging Techniques
3.1 Logging
Logging is a fundamental debugging technique. You can use NSLog to print messages to the console, providing insights into the program’s flow and variable values.
objective - (void)someMethod { NSLog(@"Entering someMethod"); // Your code here NSLog(@"Exiting someMethod"); }
3.2 Breakpoints
Breakpoints are invaluable for stopping execution at specific points in your code. You can set breakpoints in Xcode by clicking on the left margin of your source code editor. Use them to examine variable values, evaluate expressions, or step through code.
3.3 Exception Handling
Objective-C provides a robust exception-handling mechanism. Use @try, @catch, and @finally blocks to handle exceptions gracefully.
objective @try { // Code that may throw an exception } @catch (NSException *exception) { // Handle the exception } @finally { // Cleanup code }
3.4 Profiling
Profiling tools like Instruments in Xcode help you identify performance bottlenecks, memory leaks, and resource usage issues in your Objective-C code. Use them to optimize your application.
4. Common Debugging Scenarios
4.1 Memory Leaks
Memory leaks occur when you allocate memory but forget to deallocate it. Instruments’ Leaks tool can help you identify memory leaks in your Objective-C application.
4.2 Null Pointer Exceptions
Null pointer exceptions can crash your application. Proper null-checking and defensive programming can prevent these crashes.
objective if (myObject != nil) { // Safe to use myObject }
4.3 Infinite Loops
Infinite loops can hang your application. Use breakpoints and debugging techniques to identify the root cause of infinite loops and fix them.
4.4 Performance Issues
Performance problems can make your application sluggish. Profiling tools like Instruments can help you pinpoint performance bottlenecks and optimize your code.
5. Debugging Tips and Best Practices
- Keep a calm mindset: Debugging can be frustrating, but staying patient and focused is essential.
- Isolate the problem: Reproduce the issue in the simplest possible scenario to narrow down the root cause.
- Use version control: Keep your codebase in a version control system like Git to track changes and easily revert to a working state.
- Document your findings: Write down what you discover during debugging. It can be helpful for future reference.
- Pair programming: Sometimes, a fresh pair of eyes can spot issues you’ve missed.
Conclusion
Effective Objective-C debugging is a skill that every developer should master. With the right tools, techniques, and practices, you can efficiently identify and resolve issues in your code. Remember that debugging is not just about fixing problems; it’s also an opportunity to learn and grow as a programmer. So, embrace the debugging process, and you’ll become a more proficient Objective-C developer in no time. Happy debugging!
Table of Contents
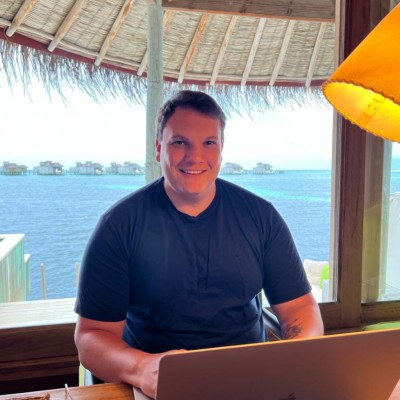
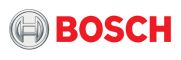