Objective-C Design Patterns: Building Maintainable iOS Code
Objective-C is a powerful and versatile programming language that has been a staple in iOS app development for many years. When it comes to building iOS applications, one of the key challenges developers face is writing maintainable and scalable code. This is where design patterns come into play. In this blog, we’ll delve into the world of Objective-C design patterns and how they can help you create iOS applications that are easier to maintain, understand, and extend.
Table of Contents
1. Why Design Patterns Matter in iOS Development
Before we dive into the specific design patterns in Objective-C, it’s important to understand why design patterns are crucial in iOS development.
1.1. Maintainability
As iOS apps grow in complexity, it becomes increasingly difficult to keep the codebase organized and understandable. Design patterns provide a structured way of organizing code, making it easier to maintain and modify. When you need to make changes or add new features to your app, having a well-defined structure in place reduces the risk of introducing bugs and makes the process more efficient.
1.2. Reusability
Design patterns encourage the reuse of code. Instead of reinventing the wheel every time you encounter a common problem, you can use established patterns that have been tried and tested. This not only saves time but also ensures that you’re using proven solutions to common challenges.
1.3. Collaboration
In a team-based development environment, design patterns act as a common language. When team members are familiar with design patterns, it’s easier to communicate ideas, share code, and work together seamlessly. This leads to a more efficient development process and higher-quality code.
Now that we understand the importance of design patterns in iOS development, let’s explore some of the most commonly used design patterns in Objective-C.
2. Common Objective-C Design Patterns
2.1. Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. This is particularly useful for managing shared resources, such as a network manager or a database connection.
Here’s an example of implementing the Singleton pattern in Objective-C:
objective // MySingleton.h @interface MySingleton : NSObject + (instancetype)sharedInstance; @end // MySingleton.m @implementation MySingleton + (instancetype)sharedInstance { static MySingleton *sharedInstance = nil; static dispatch_once_t onceToken; dispatch_once(&onceToken, ^{ sharedInstance = [[self alloc] init]; }); return sharedInstance; } @end
In this example, the sharedInstance method ensures that there’s only one instance of MySingleton created throughout the app’s lifecycle.
2.2. Factory Method Pattern
The Factory Method pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created. It’s commonly used for creating objects with complex initialization logic.
Here’s an example of the Factory Method pattern in Objective-C:
objective // Product.h @interface Product : NSObject - (void)display; @end // ConcreteProduct.h @interface ConcreteProduct : Product @end // ConcreteProduct.m @implementation ConcreteProduct - (void)display { NSLog(@"ConcreteProduct"); } @end // Creator.h @interface Creator : NSObject - (Product *)factoryMethod; @end // ConcreteCreator.h @interface ConcreteCreator : Creator @end // ConcreteCreator.m @implementation ConcreteCreator - (Product *)factoryMethod { return [[ConcreteProduct alloc] init]; } @end
In this example, Creator defines the factoryMethod, which is overridden in ConcreteCreator to create specific instances of Product.
2.3. Observer Pattern
The Observer pattern defines a one-to-many relationship between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is widely used in iOS for implementing event handling and notifications.
Here’s an example of the Observer pattern in Objective-C:
objective // Observer.h @protocol Observer <NSObject> - (void)update:(NSString *)message; @end // Subject.h @interface Subject : NSObject - (void)addObserver:(id<Observer>)observer; - (void)removeObserver:(id<Observer>)observer; - (void)notifyObservers:(NSString *)message; @end // Subject.m @implementation Subject { NSMutableArray<id<Observer>> *_observers; } - (instancetype)init { self = [super init]; if (self) { _observers = [NSMutableArray array]; } return self; } - (void)addObserver:(id<Observer>)observer { [_observers addObject:observer]; } - (void)removeObserver:(id<Observer>)observer { [_observers removeObject:observer]; } - (void)notifyObservers:(NSString *)message { for (id<Observer> observer in _observers) { [observer update:message]; } } @end
In this example, Subject maintains a list of observers and notifies them when an event occurs.
2.4. MVC (Model-View-Controller) Pattern
MVC is a fundamental design pattern in iOS development. It separates an application into three interconnected components: Model, View, and Controller. This separation of concerns makes it easier to manage the user interface, application logic, and data separately.
Here’s a high-level overview of the MVC pattern in iOS:
- Model: Represents the data and business logic of the application.
- View: Displays the user interface and receives user input.
- Controller: Acts as an intermediary between the Model and View, handling user input and updating the Model and View accordingly.
3. Benefits of Using Design Patterns in Objective-C
Now that we’ve explored some common design patterns in Objective-C, let’s discuss the benefits of incorporating these patterns into your iOS projects.
3.1. Improved Code Structure
Design patterns provide a clear and organized structure for your code. This makes it easier to understand, maintain, and debug your iOS applications. When you follow established patterns, you create a consistent and predictable codebase that is easier for you and your team to work with.
3.2. Enhanced Reusability
By using design patterns, you can encapsulate common functionality and behavior in reusable components. This not only saves you time but also promotes code reusability across different parts of your application. You can build on proven patterns instead of reinventing the wheel for each feature.
3.3. Scalability
Design patterns help your codebase scale gracefully as your iOS application grows. As new requirements and features are added, you can extend and modify your existing patterns or introduce new ones without disrupting the entire application. This flexibility ensures that your codebase remains adaptable and maintainable over time.
3.4. Collaboration and Teamwork
When your development team is familiar with design patterns, collaboration becomes more efficient. Team members can easily understand each other’s code and share common patterns and practices. This leads to smoother development processes and higher-quality code.
4. Choosing the Right Design Pattern
While design patterns offer many benefits, it’s important to choose the right pattern for your specific use case. Not every pattern will fit every situation, and forcing a pattern where it doesn’t belong can lead to unnecessary complexity.
Here are some factors to consider when selecting a design pattern for your iOS project:
4.1. Problem Domain
Start by understanding the problem you’re trying to solve. Some patterns are well-suited for specific problems, while others are more general-purpose. Choose a pattern that aligns with the requirements and challenges of your project.
4.2. Flexibility
Consider how flexible your chosen pattern is. Will it allow you to accommodate future changes and new features? A good design pattern should be adaptable and not overly rigid.
4.3. Familiarity
Take into account your team’s familiarity with the chosen pattern. If your team has experience with a particular pattern, it may be more efficient to stick with what they know. On the other hand, if the pattern is new to your team, be prepared to invest time in learning and implementation.
4.4. Performance
Evaluate the performance implications of your chosen pattern. Some patterns may introduce overhead or complexity that could impact the app’s performance. Ensure that the pattern aligns with your performance requirements.
Conclusion
Design patterns are a valuable tool in the arsenal of any Objective-C developer looking to build maintainable and scalable iOS applications. By following established patterns like Singleton, Factory Method, Observer, and MVC, you can create code that is easier to maintain, understand, and extend.
As you embark on your iOS development journey, remember that design patterns are not one-size-fits-all solutions. It’s important to choose the right pattern for each situation and be flexible in your approach. With the right design patterns in your toolkit, you’ll be well-equipped to tackle the challenges of iOS development and build high-quality, maintainable code.
So, start exploring these design patterns in Objective-C, apply them to your iOS projects, and watch your codebase become more organized and resilient. Happy coding!
Table of Contents
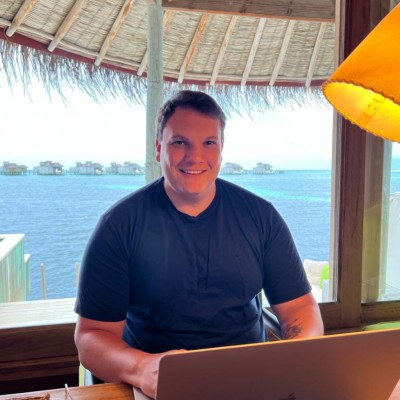
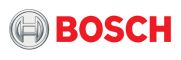