Objective-C Exception Handling: Ensuring Robust iOS Apps
In the dynamic and ever-evolving world of mobile app development, creating robust and reliable iOS applications is of paramount importance. One critical aspect of achieving this is effective exception handling. Objective-C, the programming language at the core of iOS app development, offers powerful mechanisms for managing exceptions and errors.
Exception handling is the practice of gracefully managing unexpected errors and exceptions that can occur during the execution of your iOS app. Properly handling exceptions can prevent crashes and improve the overall user experience. In this comprehensive guide, we will delve into Objective-C exception handling, exploring best practices, code samples, and error management strategies to ensure your iOS apps are robust and resilient.
1. Understanding Exceptions in Objective-C
Before diving into the details of exception handling, let’s gain a clear understanding of what exceptions are in Objective-C and when they occur.
1.1. What Are Exceptions?
In Objective-C, exceptions are a mechanism for handling runtime errors or exceptional conditions that may occur during program execution. These exceptions represent situations where the normal flow of code execution is disrupted due to unexpected circumstances, such as division by zero, accessing an invalid memory location, or trying to send a message to a nil object.
1.2. When Do Exceptions Occur?
Exceptions in Objective-C typically occur in situations where the program cannot recover gracefully. Common examples include:
- Divide by Zero: Attempting to divide a number by zero.
- Nil Messaging: Sending a message to an object that is nil (i.e., not initialized).
- Array Bounds Exceeded: Trying to access an array element beyond its bounds.
- Memory Allocation Failures: Failing to allocate memory using malloc or related functions.
- File Not Found: Attempting to access a file that does not exist.
It’s essential to handle these exceptions to prevent application crashes and provide users with meaningful error messages.
2. The Try-Catch Exception Handling Mechanism
Objective-C provides a powerful exception handling mechanism through the @try, @catch, and @finally blocks. These blocks allow you to handle exceptions gracefully and take appropriate actions when an exception occurs.
2.1. Using @try, @catch, and @finally
Here’s a basic structure of how to use @try, @catch, and @finally blocks:
objective @try { // Code that may throw an exception } @catch (NSException *exception) { // Handle the exception } @finally { // Code that always executes, whether an exception occurred or not }
Let’s break down the components:
- The @try block contains the code that you suspect may throw an exception. If an exception occurs within this block, the program will jump to the corresponding @catch block.
- The @catch block specifies how to handle the exception. It takes an NSException object as a parameter, which provides information about the exception, such as its name and reason. You can customize the error-handling logic within this block.
- The @finally block contains code that always executes, regardless of whether an exception occurred or not. It is often used for cleanup tasks, like releasing resources.
3. Example: Handling Division by Zero
Let’s look at an example of using @try, @catch, and @finally to handle a division by zero exception:
objective @try { int result = 10 / 0; // Attempting to divide by zero } @catch (NSException *exception) { NSLog(@"Exception: %@", [exception description]); // Handle the exception, e.g., display an error message } @finally { // Cleanup code, if needed }
In this example, when the code attempts to divide by zero, it triggers an exception. The program jumps to the @catch block, where you can log the exception and implement custom error-handling logic.
4. Best Practices for Exception Handling in Objective-C
To ensure robust iOS apps, it’s crucial to follow best practices when handling exceptions in Objective-C. Here are some guidelines to keep in mind:
4.1. Be Specific in Catching Exceptions
Avoid catching all exceptions indiscriminately. Instead, catch specific exceptions that you expect and can handle. This practice helps you differentiate between different error scenarios and provides more meaningful error messages to users.
objective @try { // Code that may throw a specific exception } @catch (SpecificException *exception) { // Handle the specific exception } @catch (AnotherSpecificException *exception) { // Handle another specific exception }
4.2. Keep @try Blocks Short
Limit the amount of code within @try blocks to minimize the scope of exception handling. Long @try blocks can make it challenging to identify the source of exceptions and debug issues effectively.
4.3. Use @finally for Cleanup
Utilize the @finally block for releasing resources, closing files, or performing any necessary cleanup tasks. This ensures that your app remains in a consistent state, even if an exception occurs.
4.4. Log Exceptions
Always log exceptions to help with debugging and troubleshooting. Logging the exception details, including the name and reason, can be invaluable when diagnosing issues in production.
4.5. Provide User-Friendly Error Messages
When an exception occurs, translate it into a user-friendly error message. Inform the user about the problem and suggest possible actions they can take to resolve it gracefully.
5. Exception Handling in Real-World Scenarios
To illustrate exception handling in real-world scenarios, let’s explore a couple of common use cases: network requests and file operations.
5.1. Handling Network Request Failures
In iOS app development, making network requests is a common task. These requests can fail due to various reasons, such as a loss of internet connectivity or an invalid server response. To handle such scenarios gracefully, you can use exception handling.
objective @try { // Make a network request NSData *responseData = [self fetchDataFromServer]; // Process the response [self processResponse:responseData]; } @catch (NetworkException *exception) { // Handle network-related exceptions [self displayErrorMessage:@"Network error. Please check your internet connection."]; } @catch (InvalidResponseException *exception) { // Handle invalid server response [self displayErrorMessage:@"Invalid server response. Please try again later."]; } @finally { // Cleanup code, if needed }
In this example, we use @try and @catch blocks to handle network-related exceptions and provide appropriate error messages to the user.
5.2. Managing File Operations
Working with files is another common task in iOS app development. File operations can fail if the file is missing, permissions are inadequate, or the storage is full. Exception handling can help us address these issues.
objective @try { // Attempt to read a file NSString *fileContents = [self readFile:@"example.txt"]; // Process the file contents [self processFileContents:fileContents]; } @catch (FileNotFoundException *exception) { // Handle file not found exception [self displayErrorMessage:@"File not found. Please check the file path."]; } @catch (PermissionDeniedException *exception) { // Handle permission denied exception [self displayErrorMessage:@"Permission denied. You do not have access to this file."]; } @catch (StorageFullException *exception) { // Handle storage full exception [self displayErrorMessage:@"Storage is full. Please free up space and try again."]; } @finally { // Cleanup code, if needed }
In this scenario, we use @try and @catch blocks to manage different file-related exceptions and provide user-friendly error messages.
6. Custom Exception Classes
Objective-C allows you to create custom exception classes that inherit from NSException. Creating custom exception classes is beneficial when you want to handle specific types of errors in a more organized and readable manner.
6.1. Creating a Custom Exception Class
Here’s how you can create a custom exception class:
objective @interface CustomException : NSException @end @implementation CustomException @end You can then throw and catch instances of this custom exception class within your code. objective Copy code @try { // Code that may throw a custom exception } @catch (CustomException *exception) { // Handle the custom exception } @finally { // Cleanup code, if needed }
Custom exception classes make your code more expressive and help distinguish between different error scenarios.
7. Handling Memory Management Exceptions
Memory management is crucial in iOS app development, and exceptions related to memory allocation and deallocation can be particularly challenging to handle. Objective-C provides ways to address these issues gracefully.
7.1. Detecting Memory-Related Issues
To detect memory-related issues, you can use tools like the Static Analyzer and Instruments. These tools help identify potential memory leaks, over-releases, and other memory-related problems in your code.
7.2. Handling Memory Errors
If you encounter memory-related exceptions at runtime, it’s essential to handle them appropriately. For example, if you receive an NSMallocException due to failed memory allocation, you can handle it like this:
objective @try { // Attempt to allocate memory char *buffer = malloc(SIZE_MAX); // Attempting to allocate a very large buffer if (!buffer) { @throw [NSMallocException exceptionWithName:@"MemoryAllocationFailure" reason:@"Failed to allocate memory" userInfo:nil]; } // Proceed with memory allocation } @catch (NSException *exception) { // Handle memory allocation failure [self displayErrorMessage:@"Memory allocation failed. Please try again later."]; } @finally { // Cleanup code, if needed }
In this example, we explicitly throw an NSMallocException when memory allocation fails, allowing us to catch and handle it within the @catch block.
8. Dealing with Unhandled Exceptions
While we aim to handle exceptions gracefully, there may be cases where an exception goes unhandled. In such situations, it’s crucial to set up crash reporting and monitoring mechanisms to capture and analyze these exceptions in a production environment.
Tools like Crashlytics and Sentry can help you monitor and track unhandled exceptions, allowing you to identify and prioritize issues for future app updates.
Conclusion
Exception handling is a critical aspect of ensuring robust iOS apps in Objective-C. By effectively using @try, @catch, and @finally blocks, following best practices, and creating custom exception classes, you can manage unexpected errors and provide a smoother user experience. Remember to log exceptions, offer user-friendly error messages, and handle memory-related issues appropriately. Additionally, setting up crash reporting tools for unhandled exceptions is essential for continuous app improvement. With the right exception handling strategies in place, you can create iOS apps that are resilient and reliable even in the face of unexpected errors.
Table of Contents
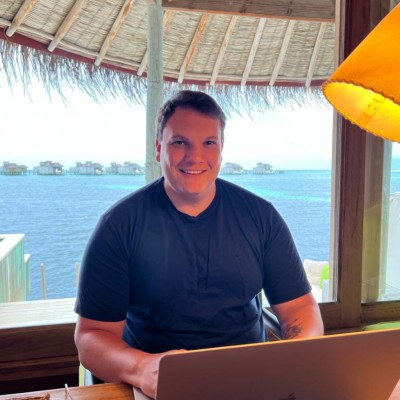
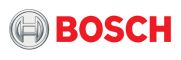