Objective-C Localization: Supporting Multiple Languages in iOS
When it comes to creating a successful and user-friendly iOS app, providing support for multiple languages is crucial. Users around the world expect apps to be available in their native language, and neglecting localization can lead to missed opportunities and negative user experiences. In this blog post, we will explore how to implement Objective-C localization in iOS to make your app accessible to a global audience. We’ll cover the basics of localization, provide code samples, and discuss best practices to ensure a seamless user experience.
Table of Contents
1. Understanding Localization
Localization is the process of adapting your app to different languages and regions, ensuring that users from various backgrounds can understand and interact with it effectively. In iOS development, Objective-C offers robust support for localization through the use of .strings files, which contain key-value pairs for translating strings into different languages. These files are associated with specific languages and regions, allowing your app to switch between them based on the user’s device settings.
1.1. Creating a Localizable.strings File
To get started with Objective-C localization, you need to create a Localizable.strings file for each language you want to support. Here’s how you can create one:
- In Xcode, right-click on your project in the Project Navigator.
- Select “New File…”
- Choose “Strings File” under the Resource section and click “Next.”
- Name the file “Localizable.strings” and click “Create.”
Repeat this process for each language you intend to support. Each Localizable.strings file will contain translations for a specific language.
1.2. Adding Translations
Once you’ve created the Localizable.strings files, you can start adding translations. Open each file and add key-value pairs in the following format:
objective "key" = "translation";
For example, if you want to translate the word “Hello” into French, your Localizable.strings file for French might look like this:
objective "hello" = "Bonjour";
1.3. NSLocalizedString Macro
To retrieve localized strings in your Objective-C code, you can use the NSLocalizedString macro. This macro takes two arguments: the key and a comment. The comment is optional and can be used for context or clarification. Here’s an example of how to use NSLocalizedString in your code:
objective NSString *localizedString = NSLocalizedString(@"hello", @"Greeting");
In this example, the localizedString variable will contain the translated string based on the user’s language preference.
2. Setting the App’s Development Language
Before diving into code samples, it’s essential to set your app’s development language in Xcode. This is the language in which your app’s interface and elements are initially designed. Here’s how to do it:
- In Xcode, go to your project settings.
- Under the “Info” tab, find the “Localizations” section.
- Click the “+” button and select the languages you want to add.
Now, Xcode will generate language-specific .strings files for your interface elements, such as storyboard or XIB files. You can then customize these files for each language to ensure proper localization.
3. Code Samples: Implementing Objective-C Localization
Now that you have a basic understanding of localization in iOS, let’s dive into some code samples to demonstrate how to implement Objective-C localization effectively. We’ll cover different aspects, including localized strings, images, and date formatting.
3.1. Localized Strings
As mentioned earlier, you can use the NSLocalizedString macro to retrieve localized strings in your code. Here’s a practical example of how to use it in a UILabel:
objective UILabel *titleLabel = [[UILabel alloc] initWithFrame:CGRectZero]; titleLabel.text = NSLocalizedString(@"app_title", @"App Title");
In this example, we assume that you have a key-value pair in your Localizable.strings file for “app_title.” The NSLocalizedString macro will replace it with the appropriate translation based on the user’s language settings.
3.2. Localized Images
In addition to localized strings, you may also want to provide localized images, such as icons or graphics with text. To do this, create separate asset catalogs for each language you support.
- In Xcode, go to your project settings.
- Under the “Assets” tab, click the “+” button to create a new asset catalog.
- Name the catalog with the language code (e.g., “en” for English or “fr” for French).
- Add image assets to the catalog and provide language-specific versions.
Now, Xcode will automatically load the appropriate image based on the user’s device language.
3.3. Date Formatting
Date and time formats can vary significantly between languages and regions. To ensure that your app displays dates correctly, you can use the NSDateFormatter class with localization support. Here’s an example of how to format a date:
objective NSDate *date = [NSDate date]; NSDateFormatter *formatter = [[NSDateFormatter alloc] init]; formatter.dateStyle = NSDateFormatterMediumStyle; formatter.timeStyle = NSDateFormatterShortStyle; NSString *localizedDate = [formatter stringFromDate:date];
By setting the dateStyle and timeStyle properties, you can automatically format the date and time according to the user’s locale.
4. Testing Localization in the Simulator
To test your app’s localization in the iOS Simulator, follow these steps:
- Run your app in the Simulator.
- Go to the “Settings” app within the Simulator.
- Scroll down and select “General.”
- Tap “Language & Region.”
- Choose a different language and region.
Your app should now switch to the selected language, displaying localized strings, images, and date formats accordingly.
5. Best Practices for Objective-C Localization
To ensure a smooth localization process and deliver a great user experience, consider the following best practices:
5.1. Use Descriptive Key Names
When creating keys for your localized strings, use descriptive names that make it easy to understand their context. This will help translators and fellow developers understand where and how each string is used in your app.
5.2. Keep Text Short and Concise
Remember that translations may vary in length, so try to keep your original text short and concise to accommodate different languages without layout issues.
5.3. Avoid Concatenating Strings
Avoid concatenating strings in your code, as word order and sentence structure can differ in various languages. Instead, use placeholders and string formatting to construct dynamic sentences.
5.4. Collaborate with Translators
If possible, work closely with professional translators who are fluent in the target languages. They can provide accurate translations and ensure cultural nuances are considered.
5.5. Regularly Update Translations
As your app evolves, make sure to update and maintain your translations. New features, UI changes, or bug fixes may require adjustments in your localized files.
Conclusion
Effective localization is a key element of a successful iOS app, as it opens up your application to a global audience and enhances the user experience. With Objective-C’s support for localization, you can easily provide translations for strings, images, and date formats. By following best practices and testing thoroughly, you can ensure that your app delivers a seamless experience to users around the world, regardless of their language or region.
Incorporating localization from the beginning of your iOS app development process not only expands your user base but also demonstrates your commitment to creating inclusive and accessible software. So, start localizing today and make your app a global success!
Table of Contents
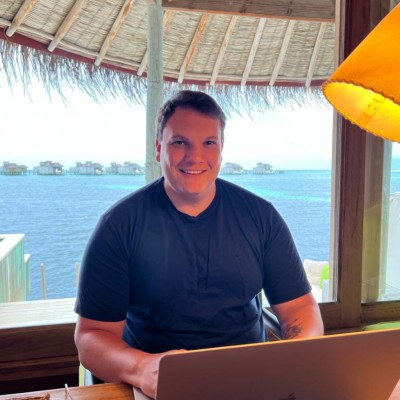
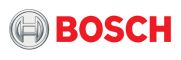