Objective-C Multithreading: Building Responsive iOS Applications
In the world of iOS app development, responsiveness is key to providing a seamless user experience. Users expect apps to be quick and smooth, and this is where multithreading comes into play. Objective-C, the language that has powered iOS development for years, offers powerful tools for building responsive applications through multithreading.
Table of Contents
In this comprehensive guide, we’ll delve into the world of Objective-C multithreading, exploring the concepts, best practices, and code samples that will empower you to create iOS applications that respond swiftly to user interactions.
1. Why Multithreading Matters
Before we dive into the details of multithreading in Objective-C, it’s essential to understand why it matters. Multithreading allows an application to perform multiple tasks concurrently, enabling it to remain responsive while handling complex operations in the background. This is crucial for tasks such as fetching data from the internet, processing large datasets, or performing time-consuming calculations—all without freezing the app’s user interface.
1.1. The Problem of Blocking the Main Thread
In iOS development, the main thread is responsible for handling the user interface (UI). Any long-running task, if executed on the main thread, will block the UI, causing the app to appear unresponsive or even freeze. This is something you definitely want to avoid to ensure a positive user experience.
Multithreading allows you to move these time-consuming tasks to background threads, leaving the main thread free to handle user interactions. Let’s dive into the world of Objective-C multithreading and see how you can achieve this.
2. Threading Basics in Objective-C
2.1. Creating a New Thread
In Objective-C, you can create a new thread by using the NSThread class. Here’s a simple example of how to create and start a new thread:
objective NSThread *myThread = [[NSThread alloc] initWithTarget:self selector:@selector(myThreadEntryPoint:) object:nil]; [myThread start];
In this code, we create an NSThread object, specifying the target object (in this case, self) and the method (myThreadEntryPoint:) that should be executed on the new thread.
2.2. Thread Entry Point
The method specified as the thread entry point should have the following signature:
objective - (void)myThreadEntryPoint:(id)object;
This method will be executed on the new thread when you call start on the NSThread object.
2.3. Thread Synchronization
When working with multiple threads, it’s crucial to ensure thread safety. Thread safety prevents data races and ensures that data is accessed and modified safely by multiple threads. Objective-C provides several mechanisms for thread synchronization, including:
2.3.1. @synchronized
You can use the @synchronized directive to create a critical section in your code. This ensures that only one thread can access the synchronized block at a time. Here’s an example:
objective - (void)myThreadEntryPoint:(id)object { @synchronized(self) { // Perform thread-safe operations here } }
2.3.2. NSLock
The NSLock class provides a more explicit way to control access to resources in a multithreaded environment. You can create an NSLock object and use it to lock and unlock sections of your code where thread safety is required.
objective NSLock *myLock = [[NSLock alloc] init]; - (void)myThreadEntryPoint:(id)object { [myLock lock]; // Perform thread-safe operations here [myLock unlock]; }
These are just some of the basic threading concepts in Objective-C. As you become more comfortable with multithreading, you can explore more advanced topics like thread priorities, thread communication, and thread pooling.
3. Grand Central Dispatch (GCD)
While NSThread is a powerful tool for managing threads in Objective-C, Apple introduced a higher-level abstraction called Grand Central Dispatch (GCD) to simplify multithreading. GCD is built on top of the underlying threading system but provides a more intuitive and efficient way to manage tasks.
3.1. Dispatch Queues
At the core of GCD are dispatch queues. Dispatch queues are data structures that manage the execution of tasks in a concurrent or serial fashion. GCD provides two types of dispatch queues:
3.1.1. Serial Queues
Serial queues execute tasks one at a time in the order they are added to the queue. This ensures that tasks are executed in a predictable, sequential manner. You can create a serial queue like this:
objective dispatch_queue_t mySerialQueue = dispatch_queue_create("com.example.serialQueue", NULL);
3.1.2. Concurrent Queues
Concurrent queues allow multiple tasks to run concurrently, taking full advantage of multi-core processors. Tasks added to a concurrent queue can run in any order and overlap with each other. Creating a concurrent queue is just as easy:
objective dispatch_queue_t myConcurrentQueue = dispatch_queue_create("com.example.concurrentQueue", DISPATCH_QUEUE_CONCURRENT);
3.2. Dispatching Tasks
Once you have a dispatch queue, you can submit tasks to it for execution. GCD provides several ways to dispatch tasks, with the most common being dispatch_async. Here’s how you can use it:
objective dispatch_async(mySerialQueue, ^{ // Perform a task asynchronously on the serial queue });
In this example, the block of code will be executed asynchronously on the serial queue, allowing you to offload work from the main thread.
3.3. Main Queue
GCD also provides a special queue called the main queue, which is the main thread’s counterpart. You should use the main queue for any UI-related tasks to ensure they are executed on the main thread. Here’s how you can dispatch a task to the main queue:
objective dispatch_async(dispatch_get_main_queue(), ^{ // Perform a UI-related task on the main queue });
This ensures that the UI updates are done on the main thread, preventing any issues with UI responsiveness.
4. Practical Example: Image Download
Let’s put our knowledge of multithreading into practice with a practical example: downloading images asynchronously in an iOS app. This is a common use case where multithreading can significantly improve the user experience.
4.1. Without Multithreading
First, let’s see how image downloading would look without multithreading. Suppose you have a function to download an image from a remote server:
objective - (UIImage *)downloadImageFromURL:(NSURL *)url { NSData *imageData = [NSData dataWithContentsOfURL:url]; return [UIImage imageWithData:imageData]; }
If you call this function on the main thread while loading multiple images, the UI will become unresponsive until all the images are downloaded. Users will experience lag and may think the app has frozen.
4.2. Using GCD for Image Download
Now, let’s improve this by using GCD to download images asynchronously:
objective - (void)downloadImageFromURL:(NSURL *)url completion:(void (^)(UIImage *))completion { dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{ NSData *imageData = [NSData dataWithContentsOfURL:url]; UIImage *image = [UIImage imageWithData:imageData]; dispatch_async(dispatch_get_main_queue(), ^{ completion(image); }); }); }
In this updated code, we perform the image download on a background queue, preventing it from blocking the main thread. Once the download is complete, we dispatch the image back to the main queue and call the completion block, allowing you to update the UI with the downloaded image.
5. Best Practices for Objective-C Multithreading
While multithreading can significantly improve the responsiveness of your iOS applications, it can also introduce complexities and potential issues. Here are some best practices to keep in mind:
5.1. Use GCD for Asynchronous Tasks
Whenever possible, use Grand Central Dispatch (GCD) to manage concurrency and parallelism. GCD simplifies multithreading and is optimized for performance.
5.2. Avoid Direct Manipulation of UI on Background Threads
Never update the user interface (UI) directly on a background thread. Always dispatch UI-related tasks to the main queue to ensure a smooth and responsive user experience.
5.3. Be Mindful of Deadlocks
Deadlocks can occur when two or more threads are waiting for each other to release a resource. Be cautious when using locks and ensure proper synchronization to avoid deadlocks.
5.4. Consider Thread Safety
When sharing data across threads, use appropriate synchronization mechanisms like @synchronized or NSLock to prevent data races and ensure thread safety.
5.5. Profile and Optimize
Use profiling tools like Instruments to identify performance bottlenecks and optimize your multithreaded code. Monitoring and analyzing your app’s performance is essential for delivering a top-notch user experience.
Conclusion
Objective-C multithreading is a powerful tool that can take your iOS applications to the next level in terms of responsiveness and performance. By leveraging concepts like NSThread and Grand Central Dispatch (GCD), you can efficiently manage tasks in the background, ensuring that your app remains snappy and user-friendly.
As you continue to explore multithreading in Objective-C, remember to follow best practices, prioritize thread safety, and profile your code for optimization. With these skills in your toolkit, you’ll be well-equipped to build responsive iOS applications that delight users and keep them engaged.
Start implementing multithreading in your Objective-C projects today, and experience the difference it can make in your iOS app development journey. Happy coding!
Table of Contents
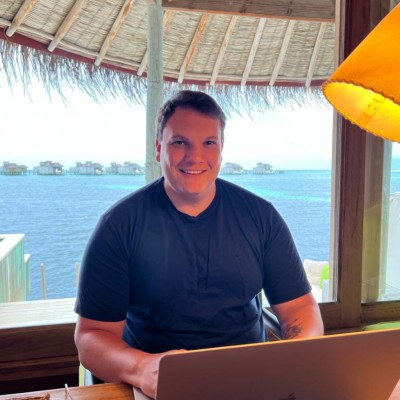
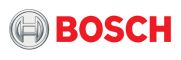