Objective-C Protocols: Enhancing Code Reusability in iOS
In the ever-evolving world of iOS app development, one thing remains constant: the need for code reusability. Code reusability is a fundamental principle that saves developers time, reduces errors, and improves maintainability. Objective-C, the programming language that has been the backbone of iOS development for years, offers a powerful feature called protocols that can significantly enhance code reusability.
In this blog, we will delve into the world of Objective-C protocols, understand their importance, and explore how they can be used to create modular and reusable code in iOS applications. We’ll cover the following topics:
1. What Are Objective-C Protocols?
In Objective-C, a protocol is a way to declare a set of methods that a class should implement. Think of a protocol as a blueprint or a contract that a class agrees to follow. It defines the methods that must be implemented but doesn’t provide the actual implementation.
objective @protocol MyProtocol - (void)doSomething; - (NSString *)getData; @end
In this example, we’ve defined a protocol called MyProtocol with two methods: doSomething and getData. Any class that adopts this protocol must implement these methods.
2. Why Are Protocols Important in iOS Development?
Protocols play a pivotal role in iOS development for several reasons:
2.1. Encapsulation and Abstraction
Protocols enable encapsulation and abstraction by defining a clear interface for classes. This separation of interface from implementation allows developers to focus on what a class should do (the interface) without concerning themselves with how it does it (the implementation).
2.2. Code Reusability
By adhering to protocols, you create reusable components that can be used in different parts of your app or even in entirely different apps. This reduces redundancy and saves development time.
2.3. Polymorphism
Protocols facilitate polymorphism, which allows objects of different classes to be treated as instances of a common interface. This makes your code more flexible and adaptable to changes.
3. Using Protocols to Define Interfaces
As mentioned earlier, protocols define a set of methods that a class should implement. Let’s see how we can define and use a protocol in Objective-C.
objective @protocol MyProtocol - (void)doSomething; - (NSString *)getData; @end
In this example, we’ve defined a protocol called MyProtocol with two methods: doSomething and getData. Now, let’s create a class that adopts this protocol and implements its methods.
objective @interface MyClass : NSObject <MyProtocol> @end @implementation MyClass - (void)doSomething { // Implementation of doSomething } - (NSString *)getData { // Implementation of getData } @end
Here, MyClass adopts the MyProtocol protocol by including it in its interface declaration. It must then provide implementations for the doSomething and getData methods as required by the protocol.
4. Implementing Protocols in Classes
When a class adopts a protocol, it must adhere to the contract defined by that protocol. In Objective-C, this means implementing all the methods declared in the protocol. Failure to do so will result in a compile-time error.
Now, let’s create another class that adopts the MyProtocol protocol but provides its own implementations for the methods.
objective @interface AnotherClass : NSObject <MyProtocol> @end @implementation AnotherClass - (void)doSomething { // Custom implementation of doSomething } - (NSString *)getData { // Custom implementation of getData } @end
In this example, AnotherClass adopts the MyProtocol protocol and provides its own unique implementations for doSomething and getData. This demonstrates how different classes can conform to the same protocol while providing their own specialized functionality.
5. Delegates: A Practical Use Case
One of the most common and powerful use cases for protocols in iOS development is the delegation pattern. Delegation allows one object to act on behalf of another, making it a fundamental part of many iOS frameworks, including UIKit.
5.1. What Is a Delegate?
A delegate is an object that conforms to a specific protocol and is assigned to another object as its delegate. The delegating object can then call methods on the delegate to notify it of events or request information.
For example, consider a table view in an iOS app. The table view can have a delegate object that conforms to the UITableViewDelegate protocol. This delegate is responsible for handling events such as row selection and cell customization.
objective @interface MyTableViewController : UIViewController <UITableViewDelegate> @property (nonatomic, strong) UITableView *tableView; @end
In this case, MyTableViewController adopts the UITableViewDelegate protocol to handle table view events.
6. Code Reusability in Action: A Real-World Example
To demonstrate the power of Objective-C protocols in enhancing code reusability, let’s consider a real-world example. Imagine you are developing an e-commerce app with various payment gateways, such as PayPal, Stripe, and Apple Pay. Each payment gateway requires different implementations for processing payments.
6.1. Protocol Definition
First, let’s define a protocol called PaymentGateway that outlines the methods needed for processing payments.
objective @protocol PaymentGateway - (void)processPayment:(float)amount; - (BOOL)isPaymentSuccessful; @end
The PaymentGateway protocol declares two methods: processPayment: and isPaymentSuccessful. Now, let’s create classes for each payment gateway, all of which will conform to this protocol.
6.2. Implementing Payment Gateways
1. PayPal
objective @interface PayPalGateway : NSObject <PaymentGateway> @end @implementation PayPalGateway - (void)processPayment:(float)amount { // Implement PayPal payment processing } - (BOOL)isPaymentSuccessful { // Check if PayPal payment was successful } @end
2. Stripe
objective @interface StripeGateway : NSObject <PaymentGateway> @end @implementation StripeGateway - (void)processPayment:(float)amount { // Implement Stripe payment processing } - (BOOL)isPaymentSuccessful { // Check if Stripe payment was successful } @end
3. Apple Pay
objective @interface ApplePayGateway : NSObject <PaymentGateway> @end @implementation ApplePayGateway - (void)processPayment:(float)amount { // Implement Apple Pay payment processing } - (BOOL)isPaymentSuccessful { // Check if Apple Pay payment was successful } @end
With this approach, we’ve created separate classes for each payment gateway, all of which adhere to the PaymentGateway protocol. This design promotes code reusability because you can easily switch between payment gateways by changing just a few lines of code.
Conclusion
Objective-C protocols are a powerful tool in an iOS developer’s arsenal. They enable code reusability, encapsulation, and abstraction, making your codebase cleaner and more maintainable. Protocols also play a crucial role in the delegation pattern, a cornerstone of iOS development.
By adopting protocols and designing your classes to conform to them, you can create modular, interchangeable components that enhance the flexibility and scalability of your iOS applications. Whether you’re building an e-commerce app with multiple payment gateways or a complex user interface with numerous custom views, Objective-C protocols can help you achieve cleaner, more reusable code.
In summary, embrace Objective-C protocols in your iOS development journey to take full advantage of their ability to enhance code reusability, promote good design practices, and make your apps more robust and adaptable.
Start implementing protocols in your iOS projects today, and watch your codebase become more modular, maintainable, and efficient. Happy coding!
Table of Contents
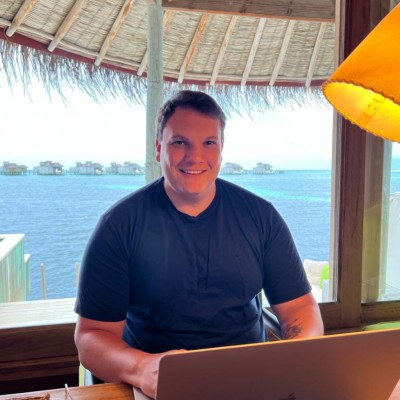
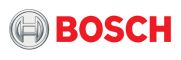