Objective-C and Push Notifications: Engaging Users in iOS
Push notifications have become a cornerstone of mobile app engagement strategies, and for good reason. They are a powerful tool for re-engaging users, delivering timely updates, and driving user retention. If you’re an iOS app developer, mastering push notifications is essential to keep your users informed and engaged. In this blog post, we’ll delve into the world of Objective-C and push notifications, exploring how you can effectively implement them in your iOS app.
Table of Contents
1. Why Push Notifications Matter
Before we dive into the technical details of implementing push notifications in Objective-C, let’s understand why they are crucial for your iOS app’s success.
1.1. User Engagement
Push notifications are one of the most direct ways to engage with your app’s users. They can notify users about new features, discounts, or other relevant information, bringing them back into your app.
1.2. Timely Updates
Keeping your users informed about important updates, such as news articles, sports scores, or product releases, can be accomplished effortlessly with push notifications. This real-time communication keeps your app relevant and users informed.
1.3. Retention Boost
Apps that effectively use push notifications tend to have better user retention rates. By reminding users of your app’s value, you can reduce churn and keep users coming back.
2. Getting Started with Push Notifications in Objective-C
Now that we’ve established the importance of push notifications, let’s get started with implementing them in Objective-C.
2.1. Configuring Push Notifications
To enable push notifications in your iOS app, you need to configure the necessary settings in your Xcode project and on the Apple Developer Portal.
Code Sample – Enabling Push Notifications:
objective // Request permission for push notifications UNUserNotificationCenter *center = [UNUserNotificationCenter currentNotificationCenter]; [center requestAuthorizationWithOptions:(UNAuthorizationOptionAlert | UNAuthorizationOptionSound | UNAuthorizationOptionBadge) completionHandler:^(BOOL granted, NSError * _Nullable error) { if (granted) { NSLog(@"Permission granted for push notifications"); } else { NSLog(@"Permission denied for push notifications"); } }];
2.2. Registering for Remote Notifications
Once permissions are granted, you can register your app for remote notifications.
Code Sample – Registering for Remote Notifications:
objective [[UIApplication sharedApplication] registerForRemoteNotifications];
2.3. Handling Push Notifications
To handle incoming push notifications, you need to implement the didReceiveRemoteNotification method in your app’s delegate.
Code Sample – Handling Push Notifications:
objective - (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo { // Handle the received notification }
2.4. Customizing Notifications
You can customize the appearance and behavior of your push notifications by creating custom notification content extensions.
Code Sample – Custom Notification Content Extension:
objective @interface NotificationViewController : UIViewController <UNNotificationContentExtension> @end @implementation NotificationViewController - (void)didReceiveNotification:(UNNotification *)notification { // Customize the notification content here } @end
2.5. Sending Push Notifications from Server
To send push notifications to your app, you’ll need a server-side component. You can use Apple’s Push Notification Service (APNs) to send notifications to registered devices.
2.6. Handling Notification Actions
You can add interactive elements to your push notifications using notification actions. For example, allowing users to like or comment directly from the notification.
Code Sample – Adding Notification Actions:
objective UNNotificationAction *likeAction = [UNNotificationAction actionWithIdentifier:@"LIKE_ACTION" title:@"Like" options:UNNotificationActionOptionForeground]; UNNotificationAction *commentAction = [UNNotificationAction actionWithIdentifier:@"COMMENT_ACTION" title:@"Comment" options:UNNotificationActionOptionForeground]; UNNotificationCategory *category = [UNNotificationCategory categoryWithIdentifier:@"MY_CATEGORY" actions:@[likeAction, commentAction] intentIdentifiers:@[] options:UNNotificationCategoryOptionCustomDismissAction]; NSSet *categories = [NSSet setWithObject:category]; [center setNotificationCategories:categories];
3. Advanced Push Notification Features
Now that you’ve got the basics down, let’s explore some advanced features and best practices for implementing push notifications in Objective-C.
3.1. Silent Push Notifications
Silent push notifications are notifications that don’t display a message to the user but instead trigger background tasks. They are useful for updating content in the background, like fetching new emails or refreshing data.
Code Sample – Sending Silent Push Notifications:
objective { "aps" : { "content-available" : 1 }, "custom_key" : "custom_value" }
3.2. Personalization and Segmentation
To make your push notifications more effective, you can personalize them based on user preferences and behavior. Additionally, segmenting your user base allows you to send targeted notifications.
3.3. A/B Testing
Experiment with different notification styles and content to determine what resonates best with your audience. A/B testing can help you optimize your push notification strategy.
3.4. Opt-Out Mechanism
Respect user preferences and provide an easy way for users to opt out of receiving push notifications. Failing to do so can lead to negative user experiences and complaints.
3.5. Analytics and Tracking
Implement analytics to track the performance of your push notifications. Measure open rates, click-through rates, and conversion rates to fine-tune your strategy.
4. Avoiding Common Pitfalls
While push notifications can greatly benefit your app, there are some common pitfalls to avoid:
4.1. Overuse of Notifications
Sending too many notifications can annoy users and lead to them disabling notifications for your app. Be judicious in your use of push notifications.
4.2. Poor Timing
Consider your users’ time zones and daily routines when sending notifications. Avoid sending them at inconvenient times, such as late at night.
4.3. Irrelevant Content
Ensure that the content of your notifications is relevant and valuable to the user. Irrelevant notifications can lead to frustration and opt-outs.
4.4. Lack of Testing
Always thoroughly test your push notifications on various iOS devices and configurations to ensure they work as expected.
Conclusion
Push notifications are a vital tool for engaging users in your iOS app, and mastering their implementation in Objective-C can significantly enhance your app’s success. By following best practices, customizing notifications, and staying mindful of user preferences, you can create a push notification strategy that keeps users informed and engaged, ultimately leading to higher retention rates and a more successful app.
Remember that while push notifications are a powerful engagement tool, they should be used responsibly and with consideration for the user experience. When done right, push notifications can be a win-win for both developers and users, keeping your app relevant and your audience engaged.
Table of Contents
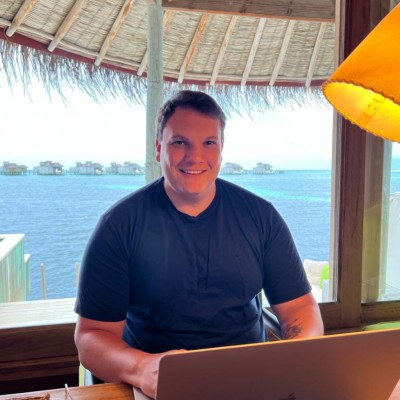
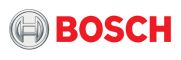