Objective-C Security: Protecting Data in iOS Applications
In an era dominated by smartphones, the need to secure user data within iOS applications has never been more critical. Whether you’re building a financial app, a messaging platform, or a game, protecting sensitive user information is paramount. Objective-C, a language that’s been at the core of iOS development for years, provides robust tools and techniques to enhance the security of your iOS applications.
Table of Contents
This blog post will delve into Objective-C security, covering encryption, data protection, and best practices to safeguard user data effectively. By the end, you’ll have a comprehensive understanding of how to fortify your iOS applications against various security threats.
1. Understanding the Importance of Data Security
Before we dive into the nitty-gritty of Objective-C security, let’s take a moment to appreciate why it’s so crucial. Data breaches and privacy violations can lead to severe consequences, including financial losses, legal troubles, and reputational damage. As an iOS developer, you’re entrusted with the responsibility of ensuring that user data remains confidential and tamper-proof.
2. Encryption: The First Line of Defense
Encryption is a cornerstone of data security. It involves converting plaintext data into a scrambled format that can only be deciphered with the appropriate decryption key. Objective-C provides several libraries and tools to implement encryption in your iOS applications.
2.1. Using CommonCryptor for Symmetric Encryption
CommonCryptor is a powerful library that allows you to perform symmetric encryption in Objective-C. Symmetric encryption uses the same key for both encryption and decryption, making it efficient for securing data at rest.
Here’s a simple example of how to use CommonCryptor for encryption:
objective #import <CommonCrypto/CommonCryptor.h> - (NSData *)encryptData:(NSData *)data withKey:(NSData *)key { size_t bufferSize = [data length] + kCCBlockSizeAES128; void *buffer = malloc(bufferSize); size_t encryptedSize = 0; CCCryptorStatus cryptStatus = CCCrypt(kCCEncrypt, kCCAlgorithmAES, kCCOptionPKCS7Padding, [key bytes], kCCKeySizeAES256, NULL, [data bytes], [data length], buffer, bufferSize, &encryptedSize); if (cryptStatus == kCCSuccess) { return [NSData dataWithBytesNoCopy:buffer length:encryptedSize]; } free(buffer); return nil; }
In this code snippet, we’re using the Advanced Encryption Standard (AES) algorithm for encryption. Make sure to securely store and manage your encryption keys to prevent unauthorized access.
3. Leveraging Keychain Services for Secure Key Storage
The Keychain Services API is a secure storage mechanism for sensitive data like encryption keys, passwords, and tokens. It’s essential to use the Keychain to store encryption keys securely, as storing them in plaintext or insecurely can lead to vulnerabilities.
Here’s how you can store and retrieve an encryption key using Keychain Services:
objective #import <Security/Security.h> - (BOOL)storeEncryptionKey:(NSData *)key withIdentifier:(NSString *)identifier { NSDictionary *query = @{ (__bridge id)kSecClass: (__bridge id)kSecClassGenericPassword, (__bridge id)kSecAttrService: @"YourApp", (__bridge id)kSecAttrAccount: identifier, (__bridge id)kSecValueData: key }; OSStatus status = SecItemAdd((__bridge CFDictionaryRef)query, NULL); return status == errSecSuccess; } - (NSData *)retrieveEncryptionKeyWithIdentifier:(NSString *)identifier { NSDictionary *query = @{ (__bridge id)kSecClass: (__bridge id)kSecClassGenericPassword, (__bridge id)kSecAttrService: @"YourApp", (__bridge id)kSecAttrAccount: identifier, (__bridge id)kSecReturnData: (__bridge id)kCFBooleanTrue }; CFDataRef keyData = NULL; OSStatus status = SecItemCopyMatching((__bridge CFDictionaryRef)query, (CFTypeRef *)&keyData); if (status == errSecSuccess) { return (__bridge_transfer NSData *)keyData; } return nil; }
In this code, we’re storing and retrieving the encryption key using the Keychain Services API, ensuring that it remains protected from unauthorized access.
4. Data Protection API: Safeguarding User Data
iOS provides the Data Protection API, which is built into the system and automatically encrypts user data at rest. This encryption ensures that even if an attacker gains physical access to the device, they won’t be able to access sensitive data without the device passcode.
To enable Data Protection for sensitive data in your app, you need to specify the appropriate data protection class when saving the data. Here are some of the available data protection classes:
- NSFileProtectionNone: Data is accessible when the device is unlocked.
- NSFileProtectionComplete: Data is inaccessible until the device is unlocked.
- NSFileProtectionCompleteUnlessOpen: Data is accessible when the device is unlocked but will be protected when not in use.
- NSFileProtectionCompleteUntilFirstUserAuthentication: Data remains protected until the user logs in for the first time after a reboot.
You can specify the data protection class when creating and saving files:
objective NSDictionary *attributes = @{ NSFileProtectionKey: NSFileProtectionComplete }; BOOL success = [data writeToURL:fileURL options:NSDataWritingFileProtectionComplete error:&error];
By choosing the appropriate data protection class, you can ensure that user data is protected according to your app’s security requirements.
5. Implementing Secure Communication
In addition to protecting data at rest, it’s crucial to secure data in transit. When your app communicates with remote servers or services, you must use secure protocols like HTTPS and implement proper certificate validation to prevent man-in-the-middle attacks.
5.1. Using NSURLSession for Secure Network Requests
NSURLSession is a powerful API for making network requests in iOS apps. To ensure secure communication, you should configure NSURLSession to use HTTPS and validate server certificates.
Here’s how you can create an NSURLSession with secure settings:
objective NSURLSessionConfiguration *config = [NSURLSessionConfiguration defaultSessionConfiguration]; config.TLSMinimumSupportedProtocol = kTLSProtocol12; // Use TLS 1.2 or higher config.TLSMaximumSupportedProtocol = kTLSProtocol12; NSURLSession *session = [NSURLSession sessionWithConfiguration:config delegate:self delegateQueue:nil];
By setting the TLSMinimumSupportedProtocol and TLSMaximumSupportedProtocol, you enforce the use of TLS 1.2 or higher, which is considered secure.
5.2. Implementing Certificate Pinning
Certificate pinning is a security mechanism that validates server certificates to ensure that your app communicates with trusted servers. To implement certificate pinning in Objective-C, you need to implement the NSURLSessionDelegate methods:
objective - (void)URLSession:(NSURLSession *)session didReceiveChallenge:(NSURLAuthenticationChallenge *)challenge completionHandler:(void (^)(NSURLSessionAuthChallengeDisposition disposition, NSURLCredential *credential))completionHandler { if ([challenge.protectionSpace.authenticationMethod isEqualToString:NSURLAuthenticationMethodServerTrust]) { SecTrustRef serverTrust = challenge.protectionSpace.serverTrust; SecCertificateRef certificate = SecTrustGetCertificateAtIndex(serverTrust, 0); NSData *serverCertificateData = CFBridgingRelease(SecCertificateCopyData(certificate)); // Compare serverCertificateData with your pinned certificate if ([serverCertificateData isEqualToData:pinnedCertificateData]) { NSURLCredential *credential = [NSURLCredential credentialForTrust:serverTrust]; completionHandler(NSURLSessionAuthChallengeUseCredential, credential); } else { completionHandler(NSURLSessionAuthChallengeCancelAuthenticationChallenge, nil); } } else { completionHandler(NSURLSessionAuthChallengePerformDefaultHandling, nil); } }
In this code, we’re comparing the server’s certificate with a pinned certificate to ensure that the connection is secure. If the certificates match, the connection is allowed; otherwise, it’s canceled.
6. Best Practices for Objective-C Security
In addition to the specific techniques and tools we’ve discussed, here are some best practices to keep in mind when enhancing the security of your Objective-C iOS applications:
6.1. Regularly Update Dependencies
Keep your Objective-C libraries and dependencies up to date. Developers often release updates to patch security vulnerabilities. Regularly reviewing and updating your dependencies can help you stay protected against known security issues.
6.2. Conduct Security Audits
Consider conducting security audits and code reviews to identify potential vulnerabilities in your code. This can be done internally or by hiring a third-party security firm to assess your app’s security posture.
6.3. Implement User Authentication
Implement robust user authentication mechanisms, such as biometric authentication (e.g., Touch ID or Face ID) or strong password policies, to ensure that only authorized users can access the app and its data.
6.4. Store Sensitive Data Securely
Always store sensitive data, such as user credentials or encryption keys, securely. Avoid hardcoding sensitive information in your code, and make use of secure storage options like the Keychain.
6.5. Educate Your Team
Ensure that your development team is aware of security best practices and is well-trained in identifying and addressing security vulnerabilities. Security is a team effort, and everyone should play their part.
Conclusion
Securing user data in iOS applications is a non-negotiable responsibility for developers. Objective-C, with its robust encryption libraries, Data Protection API, and secure communication capabilities, equips you with the tools needed to protect sensitive information effectively. By following best practices and staying informed about the latest security threats, you can build iOS applications that users can trust with their data.
Remember, security is an ongoing process. Stay vigilant, keep your code up to date, and prioritize user data protection from day one of your iOS development journey.
Table of Contents
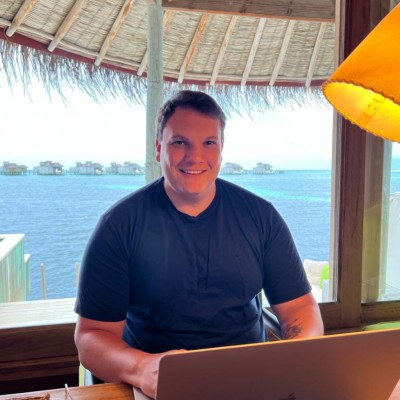
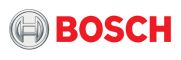