Objective-C and SiriKit: Enabling Voice Control in iOS Apps
Voice control is no longer a futuristic concept but a reality that has transformed the way we interact with technology. Apple’s Siri has played a pivotal role in bringing voice-controlled experiences to iOS devices. In this blog post, we will delve into how you can leverage SiriKit and Objective-C to empower your iOS apps with voice control capabilities.
Table of Contents
1. Understanding SiriKit
1.1. What is SiriKit?
SiriKit is a framework introduced by Apple that allows developers to integrate Siri voice commands into their apps. It enables users to interact with your app using natural language voice commands, providing a more intuitive and convenient user experience.
1.2. SiriKit Capabilities
SiriKit provides various domains or capabilities that your app can support. These domains encompass different types of actions and interactions. Some common SiriKit domains include:
1.2.1. Messaging
With the Messaging domain, users can send messages, search for messages, and manage conversations using Siri voice commands.
objective // Sample code for handling messaging with SiriKit - (void)handleSendMessage:(INSendMessageIntent *)intent completion:(void (^)(INSendMessageIntentResponse *response))completion { // Implement your logic to send a message here // ... completion([[INSendMessageIntentResponse alloc] initWithCode:INSendMessageIntentResponseCodeSuccess userActivity:nil]); }
1.2.2. Payments
The Payments domain enables users to send and request payments using Siri. You can integrate this capability into your finance or payment-related apps.
objective // Sample code for handling payments with SiriKit - (void)handleSendPayment:(INSendPaymentIntent *)intent completion:(void (^)(INSendPaymentIntentResponse *response))completion { // Implement your logic to send a payment here // ... completion([[INSendPaymentIntentResponse alloc] initWithCode:INSendPaymentIntentResponseCodeSuccess userActivity:nil]); }
1.2.3. Ride Booking
For ride-sharing apps, the Ride Booking domain allows users to book rides and manage their bookings with voice commands.
objective // Sample code for handling ride booking with SiriKit - (void)handleRequestRide:(INRequestRideIntent *)intent completion:(void (^)(INRequestRideIntentResponse *response))completion { // Implement your logic to book a ride here // ... completion([[INRequestRideIntentResponse alloc] initWithCode:INRequestRideIntentResponseCodeSuccess userActivity:nil]); }
1.2.4. Fitness
Fitness apps can utilize the Fitness domain to track workouts, start exercises, and retrieve health data through Siri.
objective // Sample code for handling fitness tracking with SiriKit - (void)handleStartWorkout:(INStartWorkoutIntent *)intent completion:(void (^)(INStartWorkoutIntentResponse *response))completion { // Implement your logic to start a workout here // ... completion([[INStartWorkoutIntentResponse alloc] initWithCode:INStartWorkoutIntentResponseCodeSuccess userActivity:nil]); }
These are just a few examples of SiriKit domains, and you can choose the one that best fits your app’s functionality.
2. Preparing Your App for Siri Integration
2.1. Set Up Your Xcode Project
To begin integrating SiriKit into your iOS app, follow these steps:
- Open your Xcode project: If you don’t have one yet, create a new project or open an existing one.
- Configure your app: In your project settings, navigate to the “Capabilities” tab and enable “Siri.”
- Add Siri intents: Create a new Siri intent definition file in your project. This file defines the intents your app can handle. You can do this by selecting “File” -> “New” -> “File…” -> “SiriKit Intent Definition File.”
- Define your app’s intents: In the intent definition file, define the specific intents your app supports. For example, if you’re building a messaging app, you would define intents for sending messages, searching for messages, and other relevant actions.
- Implement intent handlers: Create Objective-C classes that implement the intent handlers for the defined intents. These handlers contain the code to execute when SiriKit triggers an intent from your app. Make sure to conform to the relevant SiriKit protocols for each intent.
- Test your app: Use the Siri Simulator in Xcode to test your app’s Siri integration. This allows you to ensure that Siri can recognize and handle your app’s intents correctly.
3. Implementing SiriKit in Objective-C
Now that you’ve set up your Xcode project for Siri integration let’s dive into the implementation details using Objective-C.
3.1. Defining Custom Intents
To make your app Siri-compatible, you need to define custom intents. These intents specify the actions users can perform with your app through Siri. Create a .intentdefinition file in Xcode and define your custom intents.
xml <INIntentDefinitionType name="SendMessageIntent" abstract="false" extends="NSObject"> <content className="NSString" name="message" /> </INIntentDefinitionType>
In this example, we define a custom intent called SendMessageIntent that allows users to send messages through your app using Siri.
3.2. Implementing Intent Handlers
Next, create Objective-C classes to implement the intent handlers for your custom intents. For our SendMessageIntent, we’ll create a class called SendMessageIntentHandler.
objective #import <Intents/Intents.h> @interface SendMessageIntentHandler : NSObject <INSendMessageIntentHandling> - (void)handleSendMessage:(INSendMessageIntent *)intent completion:(void (^)(INSendMessageIntentResponse *response))completion; @end
In the SendMessageIntentHandler class, we conform to the INSendMessageIntentHandling protocol and implement the handleSendMessage:completion: method. This method contains the logic to send a message via your app.
3.3. Handling Intent Requests
In your intent handler class, you’ll implement the handleSendMessage:completion: method to handle Siri’s request. Here’s an example of how you might implement this method:
objective - (void)handleSendMessage:(INSendMessageIntent *)intent completion:(void (^)(INSendMessageIntentResponse *response))completion { NSString *message = intent.message; // Use the 'message' parameter to send a message via your app [self sendMessage:message]; // Provide a response to Siri INSendMessageIntentResponse *response = [[INSendMessageIntentResponse alloc] initWithCode:INSendMessageIntentResponseCodeSuccess userActivity:nil]; completion(response); }
In this code, we extract the message from the INSendMessageIntent and use it to send a message through your app. After performing the action, we create a response object and call the completion block to notify Siri of the result.
3.4. Don’t Forget Permissions
To use SiriKit in your app, you’ll need to request the necessary permissions from the user. Add the required usage descriptions to your app’s Info.plist file for the specific SiriKit domain you’re using. For example, if your app uses the Messaging domain, include the NSSiriUsageDescription key with an appropriate description in your Info.plist.
xml <key>NSUserActivityTypes</key> <dict> <key>INSendMessageIntent</key> <string>Send a message</string> </dict>
3.5. Testing Siri Integration
Before deploying your app, it’s crucial to thoroughly test your Siri integration. Use the Siri Simulator in Xcode to simulate voice commands and ensure your app responds correctly. Verify that Siri recognizes your custom intents and executes the associated actions.
4. User Experience and Design Considerations
Integrating voice control into your app with SiriKit can greatly enhance the user experience. However, to create a seamless and intuitive voice-controlled interface, you should consider the following design principles:
4.1. Natural Language Understanding
Siri excels at understanding natural language. Ensure that your app’s voice commands are conversational and easy to understand. Test different phrasings to make sure Siri can interpret user requests accurately.
4.2. Feedback and Confirmation
Provide audio or visual feedback when Siri executes a voice command. Users should receive confirmation that their request was understood and completed successfully. This feedback enhances user confidence in the voice control feature.
4.3. Error Handling
Consider different scenarios where voice commands might fail, such as network issues or incomplete information. Implement error handling to gracefully handle these situations and provide helpful guidance to users.
4.4. Privacy and Security
Voice commands can involve sensitive information. Ensure that your app’s voice control feature adheres to privacy and security best practices. Prompt users for consent when accessing personal data and make privacy settings easily accessible.
Conclusion
By integrating SiriKit and Objective-C into your iOS app, you can offer users a more engaging and efficient way to interact with your application using voice commands. SiriKit provides a powerful framework that enables seamless voice control, opening up new possibilities for user experiences.
To get started, define custom intents, implement intent handlers, and thoroughly test your app’s Siri integration. Remember to focus on user experience and design considerations to create a voice-controlled interface that enhances usability and convenience.
As voice technology continues to advance, embracing SiriKit is a strategic move that can set your iOS app apart in terms of functionality and user satisfaction. So, go ahead and explore the world of voice-controlled apps with SiriKit and Objective-C. Your users will thank you for it!
Table of Contents
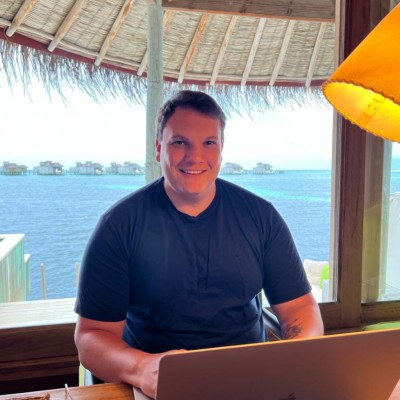
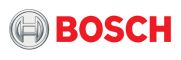