Objective-C Testing: Unit Testing and Test-Driven Development in iOS
In the dynamic world of iOS app development, ensuring the reliability and stability of your codebase is paramount. Objective-C, a venerable language with a rich history in iOS development, still plays a vital role in building robust applications. One key aspect of ensuring code quality is testing, and in this blog post, we’ll explore how to perform unit testing and embrace Test-Driven Development (TDD) in iOS using Objective-C.
1. Why Test Your Objective-C Code?
Before diving into the specifics of unit testing and Test-Driven Development in Objective-C, let’s address the fundamental question: why should you bother testing your code?
1.1. Bug Prevention and Detection
Testing allows you to identify and address bugs early in the development process, reducing the likelihood of costly and time-consuming bug fixes down the road.
1.2. Code Quality
Well-tested code is typically cleaner and more maintainable. It forces you to think about modularizing your code, which ultimately leads to better software architecture.
1.3. Refactoring Confidence
When you have a comprehensive suite of tests, you can confidently refactor your codebase without worrying about introducing new bugs.
1.4. Collaboration
Testing facilitates collaboration among team members. Tests serve as documentation and provide a clear specification of how code should behave.
Now that we’ve established the importance of testing, let’s delve into the two essential concepts in Objective-C testing: unit testing and Test-Driven Development.
2. Unit Testing in Objective-C
Unit testing involves breaking your code into small, testable units, typically individual methods or functions, and writing tests to verify their correctness. In Objective-C, you can use the XCTest framework to perform unit tests effectively.
2.1. Setting Up XCTest
To get started with XCTest in your Objective-C project, follow these steps:
- Open Xcode and create a new Objective-C project or use an existing one.
- In the project navigator, right-click on your project and select “New File.”
- Choose the “iOS” tab and select “Source.”
- From the template options, choose “Objective-C Test Case Class” and click “Next.”
- Give your test case class a name, e.g., MyAppTests, and ensure the “Also create a ‘MyAppTests.m’ file” option is checked. Click “Next” and then “Create.”
Now, you have a test case class and an associated implementation file where you can write your unit tests.
2.2. Writing Your First Unit Test
Let’s say you have a simple Objective-C class called Calculator with a method add:to: that adds two numbers. Here’s how you can write a unit test for it:
objective #import <XCTest/XCTest.h> #import "Calculator.h" @interface CalculatorTests : XCTestCase @end @implementation CalculatorTests - (void)testAddition { // Arrange Calculator *calculator = [[Calculator alloc] init]; // Act double result = [calculator add:5.0 to:7.0]; // Assert XCTAssertEqual(result, 12.0, @"Addition failed"); } @end
In this example, we import the XCTest framework, create a test case class CalculatorTests, and write a test method testAddition. Inside the test method, we:
- Arrange: Create an instance of the Calculator class.
- Act: Call the add:to: method with the values 5.0 and 7.0.
- Assert: Use XCTAssertEqual to verify that the result is equal to 12.0.
To run your tests, simply press the “Run” button (or use the shortcut Command + U) in Xcode. XCTest will execute your tests and report the results in the test navigator.
2.3. Best Practices for Unit Testing in Objective-C
Here are some best practices to keep in mind when writing unit tests in Objective-C:
1. Isolate Dependencies
Ensure that your unit tests only focus on the code you’re testing and don’t rely on external services, databases, or network calls. Use mocks and stubs when necessary to isolate dependencies.
2. Test Edge Cases
Don’t just test the happy path. Cover edge cases, boundary conditions, and error scenarios to ensure your code behaves correctly in all situations.
3. Use Descriptive Test Method Names
Give your test methods clear and descriptive names that convey what they’re testing. This makes it easier to understand the purpose of each test.
4. Keep Tests Fast
Unit tests should run quickly, so developers are encouraged to run them frequently. Slow tests can be a barrier to adopting TDD.
3. Test-Driven Development (TDD) in Objective-C
Test-Driven Development (TDD) is a software development approach that emphasizes writing tests before writing the actual code. The TDD cycle typically consists of three phases: red, green, and refactor.
3.1. The TDD Cycle
- Red: In this phase, you write a failing test case that describes the behavior you want to implement.
- Green: In this phase, you write the minimum code necessary to make the failing test pass.
- Refactor: After the test passes, you can refactor your code with confidence, knowing that you have tests in place to catch any regressions.
Let’s walk through an example of TDD in Objective-C to create a simple string manipulation class.
Step 1: Red – Write a Failing Test
objective - (void)testReverseString { // Arrange NSString *input = @"Hello, World!"; // Act NSString *result = [StringManipulator reverseString:input]; // Assert XCTAssertEqualObjects(result, @"!dlroW ,olleH", @"String reversal failed"); }
In this red phase, we write a test for a StringManipulator class that should reverse a string. Since the StringManipulator class doesn’t exist yet, this test will fail.
Step 2: Green – Write the Minimum Code
objective @implementation StringManipulator + (NSString *)reverseString:(NSString *)input { // Minimum code to make the test pass NSMutableString *reversed = [NSMutableString stringWithCapacity:[input length]]; [input enumerateSubstringsInRange:NSMakeRange(0, [input length]) options:(NSStringEnumerationReverse | NSStringEnumerationByComposedCharacterSequences) usingBlock:^(NSString *substring, NSRange substringRange, NSRange enclosingRange, BOOL *stop) { [reversed appendString:substring]; }]; return [reversed copy]; } @end
In the green phase, we implement the StringManipulator class with the minimum code required to make the test pass.
Step 3: Refactor
In the refactor phase, you can clean up and optimize your code while ensuring that your tests continue to pass.
3.2. Benefits of TDD
- Test-Driven Development offers several benefits when working with Objective-C or any other programming language:
- Improved Code Quality: TDD encourages you to write code that’s easy to test, leading to cleaner and more maintainable code.
- Reduced Debugging Time: Since you catch bugs early in the development process, you spend less time debugging.
- Confident Refactoring: With a suite of tests, you can refactor your code without fear of breaking existing functionality.
- Better Collaboration: Tests serve as documentation, making it easier for team members to understand your code and collaborate effectively.
4. Asynchronous Testing in Objective-C
In iOS development, you often work with asynchronous operations such as network requests or background tasks. Testing asynchronous code can be challenging, but XCTest provides tools to handle it effectively.
4.1. Testing Asynchronous Code with Expectations
XCTest includes XCTestExpectation, a class that allows you to test asynchronous code by setting up expectations and waiting for them to fulfill. Here’s an example of testing an asynchronous network request:
objective - (void)testAsynchronousNetworkRequest { XCTestExpectation *expectation = [self expectationWithDescription:@"Network Request"]; // Assume you have a network manager class with a method `fetchDataWithCompletion:` [NetworkManager fetchDataWithCompletion:^(NSData *data, NSError *error) { XCTAssertNil(error, @"Network request failed"); XCTAssertNotNil(data, @"No data received"); [expectation fulfill]; }]; [self waitForExpectationsWithTimeout:5.0 handler:^(NSError *error) { if (error) { XCTFail(@"Expectation timed out with error: %@", error); } }]; }
In this example, we create an expectation and fulfill it when the network request completes. We use waitForExpectationsWithTimeout to wait for the expectation to be fulfilled, with a timeout of 5 seconds.
4.2. Asynchronous Testing Best Practices
When testing asynchronous code in Objective-C, consider the following best practices:
- Set a reasonable timeout for waitForExpectationsWithTimeout to avoid hanging tests.
- Ensure proper error handling and assertion checks for asynchronous operations.
- Use expectations to synchronize and control the flow of your asynchronous tests.
5. Mocking and Dependency Injection
In real-world iOS applications, your code often depends on external services, databases, or APIs. When writing unit tests, you want to isolate your code from these external dependencies. Mocking and dependency injection are valuable techniques to achieve this isolation.
5.1. Mocking with OCMock
OCMock is a popular Objective-C library for creating mock objects. It allows you to replace real objects with mock objects that simulate the behavior of external dependencies.
To use OCMock in your Objective-C unit tests, follow these steps:
- Add the OCMock framework to your Xcode project.
- Import the necessary headers in your test file:
objective #import <XCTest/XCTest.h> #import <OCMock/OCMock.h>
- Create a mock object for the class or protocol you want to replace.
- Define the behavior of the mock object using OCMock expectations.
Here’s a simple example of mocking a network request using OCMock:
objective - (void)testMockingNetworkRequest { id mockNetworkManager = OCMClassMock([NetworkManager class]); // Define the expected behavior [[[mockNetworkManager expect] andDo:^(NSInvocation *invocation) { // Simulate a successful network request void (^completionBlock)(NSData *data, NSError *error); [invocation getArgument:&completionBlock atIndex:2]; completionBlock([@"Mocked data" dataUsingEncoding:NSUTF8StringEncoding], nil); }] fetchDataWithCompletion:OCMOCK_ANY]; // Test your code that depends on NetworkManager // Verify that the method was called OCMVerify([mockNetworkManager fetchDataWithCompletion:OCMOCK_ANY]); // Clean up [mockNetworkManager stopMocking]; }
In this example, we create a mock object for the NetworkManager class and define the behavior for the fetchDataWithCompletion: method. This allows us to simulate a successful network request without actually making a network call.
5.2. Dependency Injection
Another approach to isolating dependencies is through dependency injection. Instead of directly creating or referencing external dependencies in your code, you inject them as dependencies. This makes it easier to replace real dependencies with mock objects during testing.
Consider the following example where we inject a network manager dependency into a view controller:
objective @interface MyViewController : UIViewController - (instancetype)initWithNetworkManager:(NetworkManager *)networkManager; @end @implementation MyViewController - (instancetype)initWithNetworkManager:(NetworkManager *)networkManager { self = [super init]; if (self) { _networkManager = networkManager; } return self; } // Rest of your view controller implementation @end
During testing, you can inject a mock network manager into the view controller, allowing you to control its behavior and test how the view controller interacts with it.
6. Continuous Integration and Testing
As your iOS project grows, it becomes crucial to automate your testing process. Continuous Integration (CI) tools like Jenkins, Travis CI, or GitHub Actions can help you achieve this. CI pipelines can automatically run your unit tests whenever changes are pushed to your code repository, ensuring that your code remains stable.
Here’s a high-level overview of setting up continuous integration for Objective-C projects:
- Choose a CI Service: Select a CI service that suits your project’s needs. GitHub Actions is a popular choice for GitHub-hosted repositories.
- Configure Your CI Workflow: Create a configuration file (e.g., .github/workflows/ci.yml for GitHub Actions) that defines your CI workflow. Specify the steps for building, testing, and deploying your project.
- Integrate XCTest: Ensure that your CI workflow includes the XCTest framework and runs your unit tests as part of the build process.
- Trigger on Push: Set up triggers so that your CI pipeline runs automatically whenever changes are pushed to your repository.
- Monitor and Analyze: Monitor your CI pipeline’s results and analyze test reports to identify and address issues promptly.
Conclusion
Testing is an essential part of iOS app development, even when working with Objective-C. By embracing unit testing and Test-Driven Development, you can ensure the reliability and maintainability of your codebase. Remember to write clear and descriptive test cases, cover edge cases, and consider asynchronous code, mocking, and dependency injection techniques to build robust and bug-free iOS applications.
Incorporating testing into your development workflow, along with continuous integration, will help you deliver high-quality iOS apps that provide a seamless user experience.
Start testing your Objective-C code today, and enjoy the benefits of more robust, maintainable, and bug-free iOS applications.
Table of Contents
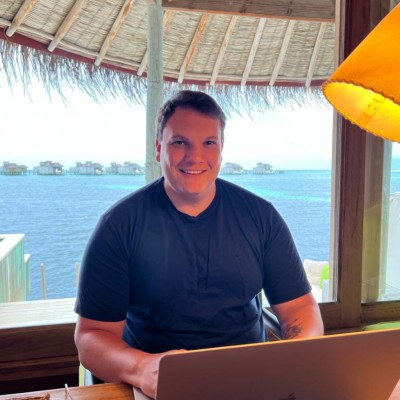
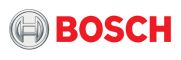