Working with Arrays and Collections in Objective-C
Objective-C, a dynamic and object-oriented programming language, has been a cornerstone of macOS and iOS app development for years. When building iOS or macOS applications, you often need to manage collections of data efficiently. This is where arrays and collections come into play. In this comprehensive guide, we’ll explore how to work with arrays and collections in Objective-C, providing you with a solid foundation for managing and manipulating data in your applications.
1. Introduction to Arrays and Collections
1.1 Understanding the Basics
Arrays and collections are fundamental data structures that allow you to store and manipulate a collection of objects. They provide a way to group related data together, making it easier to manage and work with large sets of information. Objective-C offers several classes for handling arrays and collections, each with its own unique features and use cases.
1.2 Advantages of Using Arrays and Collections
Using arrays and collections in your Objective-C code offers numerous advantages:
- Efficient Data Storage: Arrays and collections provide efficient storage for collections of objects, allowing you to access and manipulate data quickly.
- Flexibility: You can store a variety of data types in arrays and collections, including custom objects, primitive types, and other collections.
- Convenience: Objective-C provides a rich set of methods and syntax for working with arrays and collections, making it easier to perform common operations.
Now, let’s dive into the specific classes provided by Objective-C for working with arrays and collections.
2. NSArray and NSMutableArray
2.1 Creating NSArray and NSMutableArray
To create an NSArray, you can use the following syntax:
objective NSArray *immutableArray = @[@"Apple", @"Banana", @"Cherry"];
To create an NSMutableArray, which allows you to modify its contents, use:
objective NSMutableArray *mutableArray = [NSMutableArray arrayWithObjects:@"One", @"Two", @"Three", nil];
2.2 Adding and Removing Objects
To add objects to an NSMutableArray, you can use the addObject: method:
objective [mutableArray addObject:@"Four"];
To remove an object, you can use the removeObject: method:
objective [mutableArray removeObject:@"Two"];
2.3 Accessing Elements
You can access elements in an NSArray or NSMutableArray using the subscript notation:
objective NSString *fruit = mutableArray[0];
2.4 Enumerating Arrays
Objective-C provides various ways to enumerate arrays. One common approach is using fast enumeration:
objective for (NSString *item in mutableArray) { NSLog(@"%@", item); }
3. NSDictionary and NSMutableDictionary
3.1 Creating NSDictionary and NSMutableDictionary
To create an NSDictionary, use the following syntax:
objective NSDictionary *dictionary = @{@"key1": @"value1", @"key2": @"value2"};
To create an NSMutableDictionary:
objective NSMutableDictionary *mutableDictionary = [NSMutableDictionary dictionaryWithObjectsAndKeys: @"value1", @"key1", @"value2", @"key2", nil];
3.2 Adding and Removing Key-Value Pairs
You can add key-value pairs to an NSMutableDictionary using the setObject:forKey: method:
objective [mutableDictionary setObject:@"newvalue" forKey:@"newkey"];
To remove a key-value pair:
objective [mutableDictionary removeObjectForKey:@"key1"];
3.3 Accessing Values
You can access values in an NSDictionary using the key:
objective NSString *value = dictionary[@"key1"];
3.4 Enumerating Dictionaries
To iterate through the keys and values of a dictionary, you can use fast enumeration:
objective for (NSString *key in dictionary) { NSString *value = dictionary[key]; NSLog(@"%@: %@", key, value); }
4. NSSet and NSMutableSet
4.1 Creating NSSet and NSMutableSet
To create an NSSet:
objective NSSet *set = [NSSet setWithObjects:@"A", @"B", @"C", nil];
To create an NSMutableSet:
objective NSMutableSet *mutableSet = [NSMutableSet setWithObjects:@"X", @"Y", @"Z", nil];
4.2 Adding and Removing Objects
To add objects to an NSMutableSet, use the addObject: method:
objective [mutableSet addObject:@"W"];
To remove an object:
objective [mutableSet removeObject:@"X"];
4.3 Testing for Membership
You can check if an object is a member of a set:
objective if ([set containsObject:@"A"]) { NSLog(@"A is in the set"); }
4.4 Enumerating Sets
To enumerate the elements of a set, you can use fast enumeration:
objective for (NSString *item in set) { NSLog(@"%@", item); }
5. Working with Custom Objects
5.1 Creating Custom Classes
To work with custom objects, you’ll first need to create custom classes that conform to the NSObject protocol. Here’s an example:
objective @interface Person : NSObject @property (nonatomic, strong) NSString *name; @property (nonatomic, assign) NSInteger age; @end @implementation Person @end
5.2 Storing Custom Objects in Collections
You can store custom objects in arrays, dictionaries, and sets just like any other objects:
objective Person *person1 = [[Person alloc] init]; person1.name = @"Alice"; person1.age = 30; Person *person2 = [[Person alloc] init]; person2.name = @"Bob"; person2.age = 25; NSArray *peopleArray = @[person1, person2];
5.3 Searching and Sorting Custom Objects
You can search for custom objects in an array using NSPredicate and sort them using NSSortDescriptor. For example, to find all people older than 28:
objective NSPredicate *predicate = [NSPredicate predicateWithFormat:@"age > 28"]; NSArray *filteredArray = [peopleArray filteredArrayUsingPredicate:predicate];
To sort the array by name:
objective NSSortDescriptor *nameSortDescriptor = [NSSortDescriptor sortDescriptorWithKey:@"name" ascending:YES]; NSArray *sortedArray = [peopleArray sortedArrayUsingDescriptors:@[nameSortDescriptor]];
6. Best Practices
6.1 Memory Management
When working with collections in Objective-C, remember to manage memory properly. Ensure that you release objects when you’re done with them, especially when dealing with mutable collections.
6.2 Enumerating with Blocks
In modern Objective-C, you can use block-based enumeration for more flexible and concise code:
objective [peopleArray enumerateObjectsUsingBlock:^(Person *person, NSUInteger index, BOOL *stop) { NSLog(@"Person: %@", person.name); }];
6.3 Performance Considerations
Consider the performance implications of the data structures you choose. For example, if you frequently need to search for elements, using an NSSet might be more efficient than an NSArray.
Conclusion
Working with arrays and collections in Objective-C is essential for building robust iOS and macOS applications. With the knowledge gained from this guide, you’ll be well-equipped to manage and manipulate data efficiently in your Objective-C projects. Arrays, dictionaries, and sets, along with the ability to work with custom objects, provide you with powerful tools to handle a wide range of data scenarios. As you continue your journey in Objective-C development, mastering these collection classes will be invaluable. Happy coding!
Table of Contents
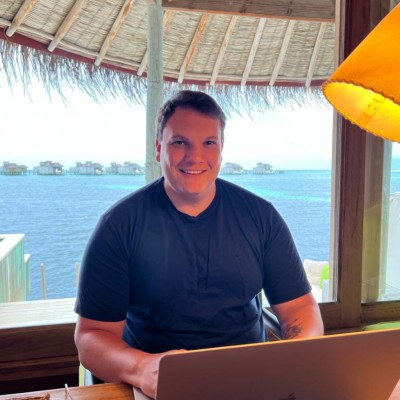
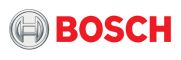