Ruby for Web Scraping: Extracting Data from Websites
In today’s data-driven world, the ability to extract information from websites efficiently has become increasingly valuable. Web scraping allows us to collect data from various sources, opening up opportunities for insights, analysis, and automation. Among the myriad of programming languages available, Ruby stands out as a robust and flexible choice for web scraping tasks. In this blog, we’ll explore the ins and outs of web scraping with Ruby, covering essential concepts, tools, and code samples to help you become proficient in data extraction.
Table of Contents
1. Understanding Web Scraping
Web scraping refers to the process of automatically extracting data from websites. It involves sending HTTP requests to target websites, parsing the HTML content, and extracting the relevant data. Web scraping has numerous applications, such as competitive analysis, market research, content aggregation, and more. However, it’s essential to use web scraping responsibly and ethically, respecting website owners’ terms of service and privacy policies.
2. Setting Up Ruby Environment
Before diving into web scraping with Ruby, let’s ensure you have the necessary tools installed. First, make sure you have Ruby installed on your system. You can download the latest version from the official Ruby website. Additionally, we recommend using a package manager like RubyGems to manage your Ruby libraries. Once Ruby is set up, install the required libraries for web scraping: Nokogiri and HTTParty.
3. Exploring Nokogiri
Nokogiri is a powerful gem for parsing HTML and XML documents. It allows us to navigate through the document using CSS and XPath selectors, making it easy to locate and extract specific data. To get started with Nokogiri, install the gem and require it in your Ruby script.
ruby # Install Nokogiri gem gem install nokogiri ruby # Require Nokogiri in your Ruby script require 'nokogiri'
4. Making HTTP Requests with HTTParty
To scrape data from websites, we need to fetch the web pages first. Ruby’s HTTParty gem simplifies the process of making HTTP requests and handling responses. It supports various HTTP methods like GET, POST, PUT, DELETE, etc. Install the gem and require it in your script.
ruby # Install HTTParty gem gem install httparty ruby # Require HTTParty in your Ruby script require 'httparty'
5. Scraping Static Websites
Let’s start with a straightforward example of scraping data from a static website. For illustration purposes, we’ll extract the titles of articles from a blog’s homepage.
ruby require 'nokogiri' require 'httparty' url = 'https://exampleblog.com' response = HTTParty.get(url) html = Nokogiri::HTML(response.body) titles = html.css('h2.title') titles.each do |title| puts title.text.strip end
In this example, we use Nokogiri to parse the HTML content and CSS selectors to target the article titles. The HTTParty gem helps us make a GET request to the blog’s homepage and retrieve the HTML.
6. Handling Dynamic Websites
While the above example works well for static websites, many modern websites use JavaScript to render content dynamically. Traditional HTTP requests won’t retrieve the dynamically generated data, so we need an alternative approach.
One solution is using a headless browser like Watir or Selenium, which can interact with the webpage as a user would, allowing us to access the dynamically generated content.
ruby require 'watir' browser = Watir::Browser.new url = 'https://exampledynamic.com' browser.goto(url) # Wait for dynamic content to load sleep(5) # Extract the data content = browser.element(css: '.content').text puts content browser.close
In this code snippet, we use Watir to launch a headless browser, navigate to the target URL, and wait for the dynamic content to load before extracting and printing it.
7. Handling Pagination
Many websites split their content across multiple pages, requiring pagination handling during web scraping. We can use a loop to iterate through all pages and extract data.
ruby require 'nokogiri' require 'httparty' base_url = 'https://examplepagination.com/page/' page_number = 1 loop do url = "#{base_url}#{page_number}" response = HTTParty.get(url) html = Nokogiri::HTML(response.body) articles = html.css('div.article') break if articles.empty? articles.each do |article| # Extract and process data from each article puts article.css('h2.title').text.strip puts article.css('p.content').text.strip puts '---' end page_number += 1 end
In this example, we iterate through each page by incrementing the page_number variable until we find an empty list of articles, signifying the end of pagination.
8. Handling Authentication
Some websites require users to log in before accessing certain data. To scrape authenticated pages, we need to include authentication details in our HTTP requests.
ruby require 'httparty' # Set authentication details username = 'your_username' password = 'your_password' # Perform login request to get authentication cookies login_url = 'https://examplelogin.com/login' response = HTTParty.post(login_url, body: { username: username, password: password }) cookies = response.headers['set-cookie'] # Use cookies to access authenticated pages data_url = 'https://examplelogin.com/protected_data' response = HTTParty.get(data_url, headers: { 'Cookie' => cookies }) puts response.body
In this code, we make a POST request with the login credentials to obtain authentication cookies. We then include these cookies in subsequent requests to access the authenticated data.
9. Avoiding Overloading Servers
Web scraping can put a strain on the target website’s servers, potentially leading to server issues or IP blocks. To avoid overloading servers, implement polite scraping practices:
- Add delays between requests to avoid rapid-fire requests.
- Use randomized user-agent headers to mimic different browsers and devices.
- Monitor response codes and limit the number of retries in case of errors.
Conclusion:
Web scraping with Ruby opens up a world of possibilities for data extraction and automation. In this blog, we’ve explored the fundamentals of web scraping, essential tools like Nokogiri and HTTParty, and various techniques for handling static and dynamic websites, pagination, and authentication. Remember to scrape responsibly, respecting website policies and terms of service, and use web scraping ethically for valuable insights and automation. Armed with the knowledge and code samples provided here, you can now embark on your web scraping journey and unleash the potential of data extraction with Ruby. Happy scraping!
Table of Contents
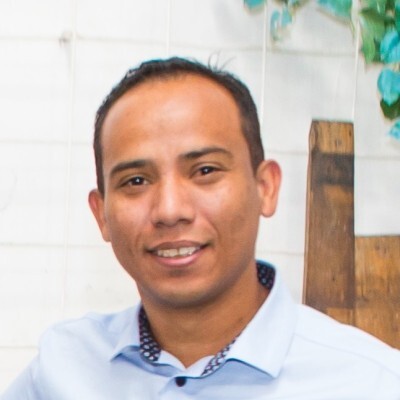
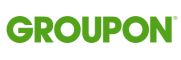