Exploring Ruby’s Cloud Computing Capabilities: Scaling and Distributed Computing
In the ever-evolving landscape of software development, the demand for scalable and distributed computing solutions is at an all-time high. With cloud computing platforms becoming increasingly popular, developers are constantly seeking ways to harness their power to build applications that can handle high traffic loads, scale dynamically, and distribute tasks efficiently.
Table of Contents
Ruby, a dynamic and versatile programming language known for its simplicity and productivity, might not be the first choice that comes to mind when thinking about cloud computing. However, Ruby’s ecosystem has evolved over the years, and it now offers a plethora of tools, libraries, and frameworks that can help you take full advantage of the cloud.
In this blog post, we’ll delve into Ruby’s cloud computing capabilities, exploring how you can use it to scale your applications and distribute computing tasks effectively. We’ll cover various aspects, including:
1. Understanding Cloud Computing
Before we dive into Ruby’s capabilities in the cloud, let’s briefly understand what cloud computing is and why it’s essential in modern software development.
1.1. What Is Cloud Computing?
Cloud computing is the delivery of computing services over the internet. These services include storage, databases, servers, networking, software, analytics, and more. Instead of owning and maintaining physical hardware and software, organizations and developers can access these services on-demand from cloud providers like Amazon Web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure.
1.2. Why Cloud Computing Matters
Cloud computing offers several advantages, such as:
- Scalability: Easily scale your resources up or down to meet demand.
- Cost-Efficiency: Pay only for what you use, reducing capital expenditures.
- Flexibility: Access a wide range of services without the burden of managing hardware.
- Reliability: Cloud providers offer high uptime guarantees and disaster recovery options.
- Global Reach: Deploy applications and services worldwide with ease.
Now that we have a basic understanding of cloud computing, let’s explore how Ruby can leverage these benefits.
2. Scaling with Ruby
One of the primary challenges in modern application development is scaling to meet increased demand. Ruby, with its elegant syntax and rich ecosystem, offers several tools and techniques to scale applications effectively.
2.1. Load Balancing
Load balancing is a crucial component of scaling applications. It involves distributing incoming traffic across multiple servers to prevent overload and ensure high availability. Ruby developers can take advantage of load balancing techniques using libraries like Nginx or by utilizing cloud services that offer load balancing as a feature.
Here’s a basic example of how you can set up load balancing using Nginx:
nginx http { upstream backend { server app_server1; server app_server2; server app_server3; } server { listen 80; location / { proxy_pass http://backend; } } }
In this example, incoming requests are distributed among three application servers, improving performance and fault tolerance.
2.2. Auto-Scaling with Ruby on Rails
Ruby on Rails, a popular web application framework, can take advantage of auto-scaling features offered by cloud providers. AWS Elastic Beanstalk, for instance, allows you to deploy Ruby on Rails applications and automatically scale resources based on traffic.
ruby # config/puma.rb workers Integer(ENV['WEB_CONCURRENCY'] || 2) threads_count = Integer(ENV['MAX_THREADS'] || 5) threads threads_count, threads_count preload_app! rackup DefaultRackup port ENV['PORT'] || 3000 environment ENV['RACK_ENV'] || 'development' on_worker_boot do # Worker specific setup ActiveSupport.on_load(:active_record) do config = ActiveRecord::Base.configurations[Rails.env] || Rails.application.config.database_configuration[Rails.env] config['pool'] = ENV['DB_POOL'] || 5 ActiveRecord::Base.establish_connection(config) end end
By configuring your Rails application correctly, you can ensure it scales seamlessly with changing traffic patterns.
2.3. Using Caching for Improved Performance
Caching is another essential technique for improving application performance and reducing server load. Ruby provides libraries like Redis and Memcached for caching data.
Here’s an example of using Redis caching in a Ruby application:
ruby # Gemfile gem 'redis' # config/application.rb config.cache_store = :redis_store, 'redis://localhost:6379/0/cache', { expires_in: 90.minutes }
By caching frequently accessed data, you can reduce the load on your database and speed up your application.
3. Distributed Computing with Ruby
Distributed computing involves breaking down complex tasks into smaller subtasks and distributing them across multiple machines or nodes. Ruby provides tools and libraries to simplify distributed computing, making it easier to manage and execute tasks across a network.
- Resque: A Distributed Queue
- Resque is a popular Ruby library for creating background jobs and managing task queues. It’s built on top of Redis and allows you to distribute and process tasks asynchronously.
Here’s a simple example of using Resque to enqueue and process a job:
ruby # Enqueue a job Resque.enqueue(MyJob, arg1, arg2) # Define the job class MyJob @queue = :my_queue def self.perform(arg1, arg2) # Your task logic here end end
Resque simplifies the process of distributing and managing background tasks, ensuring they run efficiently and reliably.
3.1. Apache Kafka for Event Streaming
Event streaming is a crucial part of many distributed systems. Apache Kafka, while not a Ruby-specific tool, can be integrated with Ruby applications to enable event-driven architectures.
Here’s a code snippet demonstrating how to produce and consume messages with the ruby-kafka gem:
ruby require 'kafka' kafka = Kafka.new(seed_brokers: ['kafka-broker1:9092', 'kafka-broker2:9092']) # Produce a message producer = kafka.producer producer.produce('my-topic', 'Hello, Kafka!') # Consume messages consumer = kafka.consumer(group_id: 'my-group') consumer.subscribe('my-topic') consumer.each_message do |message| puts "Received message: #{message.value}" end
By leveraging Apache Kafka, you can build highly scalable and event-driven systems that can handle large volumes of data and distribute tasks effectively.
4. Real-World Use Cases
To further illustrate Ruby’s cloud computing capabilities, let’s explore some real-world use cases where Ruby shines in scaling and distributed computing scenarios.
4.1. E-commerce Platforms
E-commerce websites often experience varying levels of traffic throughout the day. Ruby on Rails, combined with cloud-based auto-scaling, can handle the spikes in traffic during sales events or promotions while efficiently scaling down during quieter periods. Additionally, background job processing libraries like Resque can help manage order processing and inventory updates asynchronously.
4.2. Social Media Analytics
Analyzing social media data in real-time requires a robust distributed computing solution. Ruby can be used to build data processing pipelines that ingest, analyze, and visualize social media data streams. By leveraging cloud-based data storage and processing services, Ruby applications can scale to handle large volumes of data efficiently.
4.3. IoT Data Processing
Internet of Things (IoT) devices generate vast amounts of data that need to be processed and analyzed in real-time. Ruby’s distributed computing capabilities, coupled with cloud-based IoT platforms, enable developers to build applications that can ingest and process IoT data streams, making sense of sensor data from various devices.
Conclusion
Ruby, often associated with simplicity and elegance, may not be the first choice for cloud computing and distributed systems. However, as we’ve explored in this blog post, Ruby offers a range of tools, libraries, and frameworks that can be leveraged to scale applications and distribute computing tasks effectively.
Whether you’re building e-commerce platforms, social media analytics tools, or IoT data processing applications, Ruby can be a viable choice for harnessing the power of the cloud and achieving scalability and reliability.
As the landscape of cloud computing continues to evolve, Ruby remains a valuable tool in the hands of developers who seek to create innovative and scalable solutions.
So, if you’re a Ruby enthusiast, don’t be deterred by the notion that Ruby can’t handle the cloud. With the right knowledge and tools, you can unlock its full potential in the world of distributed and scalable computing. Happy coding!
Table of Contents
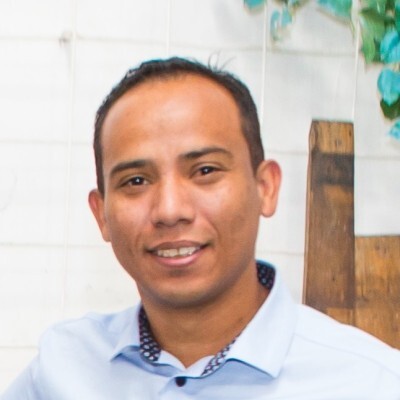
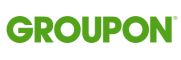