Deep Dive into TypeScript: Advanced Features Explored
TypeScript has gained immense popularity among developers due to its ability to enhance JavaScript with static typing and advanced features. While many developers are familiar with the basics of TypeScript, there are several advanced features that can take your coding experience to the next level. In this blog post, we will take a deep dive into TypeScript and explore its advanced features, including generics, conditional types, mapped types, and more. Let’s unlock the full potential of TypeScript!
1. Generics: The Power of Reusability
1.1. Introduction to Generics
Generics in TypeScript provide a way to create reusable code components that can work with a variety of data types. With generics, you can define functions, classes, and interfaces that are parameterized over types. This allows you to write flexible and type-safe code that can be used with different data types without sacrificing type checking.
1.2. Generic Functions
A generic function is a function that can operate on a range of data types. You define the generic type parameter using angle brackets (“< >”) before the function name. For example:
typescript function identity<T>(arg: T): T { return arg; } let result = identity<number>(42); // result has type number
1.3. Generic Classes
Similar to generic functions, TypeScript allows you to create generic classes. You can define a generic type parameter for the class, which can be used in methods, properties, and constructor parameters. Here’s an example:
typescript class Box<T> { private value: T; constructor(value: T) { this.value = value; } getValue(): T { return this.value; } } let box = new Box<number>(42); // box is an instance of Box<number> let value = box.getValue(); // value has type number
1.4. Generic Constraints
Sometimes you may want to restrict the types that can be used with generics. TypeScript allows you to apply constraints on generic type parameters using the extends keyword. This ensures that only certain types that satisfy the constraint can be used. Here’s an example:
typescript interface Lengthy { length: number; } function getLength<T extends Lengthy>(arg: T): number { return arg.length; } let len = getLength("hello"); // len has type number
1.5. Advanced Generics Techniques
TypeScript’s generics offer many advanced techniques, such as conditional types, mapped types, and using type parameters in combination with keyof. These techniques enable you to create more expressive and powerful code. By leveraging these advanced features, you can build highly reusable and flexible components.
2. Conditional Types: Making Types More Dynamic
2.1. Introduction to Conditional Types
Conditional types in TypeScript allow you to define types based on conditions. They provide the ability to create types that change dynamically depending on the types of other values. This enables you to write more flexible and precise type definitions.
2.2. Basic Conditional Types
A basic conditional type consists of a condition, a true branch, and a false branch. The condition can be any type, and the true and false branches can be different types. Here’s an example:
typescript type Check<T> = T extends string ? boolean : number; let value1: Check<"hello"> = true; // value1 has type boolean let value2: Check<42> = 42; // value2 has type number
2.3. Conditional Type Inference
Conditional types can be used to infer types based on other types. This is particularly useful when working with utility types like ReturnType or InstanceType. Here’s an example:
typescript function createInstance<T>(ctor: new () => T): T { return new ctor(); } class MyClass { // ... } let instance = createInstance(MyClass); // instance has type MyClass
2.4. Advanced Conditional Types
Advanced conditional types can be used to perform complex type transformations. You can use conditional type inference, type mapping, and recursive conditional types to achieve powerful type manipulations. These techniques allow you to create sophisticated type definitions tailored to your specific needs.
3. Mapped Types: Transforming Types Dynamically
3.1. Introduction to Mapped Types
Mapped types in TypeScript enable you to transform existing types into new types by iterating over their properties. They allow you to add, modify, or remove properties from an existing type, creating a transformed version of it. This provides a powerful way to create new types based on existing ones.
3.2. Readonly and Partial Mapped Types
Two commonly used mapped types are Readonly and Partial. Readonly<T> creates a new type where all properties of T are read-only, while Partial<T> creates a new type where all properties of T are optional. Here’s an example:
typescript interface Person { name: string; age: number; } type ReadonlyPerson = Readonly<Person>; type PartialPerson = Partial<Person>;
3.3. Key Remapping and Conditional Mapped Types
Mapped types also allow you to remap property names and apply conditional transformations based on property types. This provides a way to create more fine-grained transformations of types. Here’s an example:
typescript type PersonWithOptionalName = { [K in keyof Person]: K extends "name" ? string | undefined : Person[K] }; let person: PersonWithOptionalName = { name: "John", age: 25, };
3.4. Advanced Mapped Types
Mapped types offer advanced techniques such as using template literal types and infer to create complex type transformations. By combining these techniques with conditional types and generics, you can build highly expressive and precise type definitions.
4. Type Guards: Narrowing Down Types
4.1. Introduction to Type Guards
Type guards in TypeScript allow you to narrow down the type of a value within a conditional block. They provide a way to perform runtime checks that refine the type of a variable based on certain conditions. This enables you to write safer and more precise code.
4.2. typeof and instanceof Type Guards
The typeof and instanceof operators can be used as type guards in TypeScript. The typeof operator checks the runtime type of a value, while the instanceof operator checks if an object is an instance of a particular class. Here’s an example:
typescript function processValue(value: string | number) { if (typeof value === "string") { // value has type string here } else if (typeof value === "number") { // value has type number here } }
4.3. User-Defined Type Guards
You can also create your own user-defined type guards in TypeScript. A user-defined type guard is a function that returns a type predicate. It allows you to perform custom checks and refine the type of a value based on your own conditions. Here’s an example:
typescript function isString(value: unknown): value is string { return typeof value === "string"; } function processValue(value: unknown) { if (isString(value)) { // value has type string here } }
4.4. Advanced Type Guard Techniques
TypeScript provides advanced techniques for type guarding, such as discriminated unions and exhaustive type checking. These techniques allow you to handle complex type scenarios and ensure that all possible types are accounted for in your code.
5. Decorators: Customizing Your Types
5.1. Introduction to Decorators
Decorators are a powerful feature in TypeScript that allow you to customize classes, methods, properties, and parameters at design time. Decorators use the @ symbol and can be applied to different parts of your code to add metadata, modify behavior, or provide additional functionality.
5.2. Class Decorators
Class decorators are applied to classes and can modify their behavior or add metadata. They receive the class constructor as their target and can perform actions such as modifying the prototype or adding static properties. Here’s an example:
typescript function logClassName(constructor: Function) { console.log(`Class name: ${constructor.name}`); } @logClassName class MyClass { // ... }
5.3. Method and Property Decorators
Method and property decorators are applied to methods and properties within a class. They receive the class prototype, the method or property name, and a property descriptor. They can be used to modify behavior or add metadata to specific methods or properties. Here’s an example:
typescript function logMethod(target: any, propertyKey: string, descriptor: PropertyDescriptor) { console.log(`Method called: ${propertyKey}`); } class MyClass { @logMethod myMethod() { // ... } }
5.4. Parameter Decorators
Parameter decorators are applied to method or constructor parameters within a class. They receive the class prototype, the method or constructor name, and the parameter index. They can be used to modify behavior or add metadata to specific parameters. Here’s an example:
typescript function logParameter(target: any, methodName: string, parameterIndex: number) { console.log(`Parameter at index ${parameterIndex} called in ${methodName}`); } class MyClass { myMethod(@logParameter param: string) { // ... } }
5.5. Advanced Decorator Usage
Decorators can be combined and used in complex scenarios to achieve powerful effects. You can create decorators that take arguments, compose decorators, or create decorator factories. These advanced decorator techniques give you fine-grained control over your code’s behavior and functionality.
Conclusion
TypeScript offers a wide range of advanced features that can greatly enhance your development experience. By understanding and leveraging concepts such as generics, conditional types, mapped types, type guards, and decorators, you can write more expressive, reusable, and type-safe code. As you continue to explore TypeScript’s advanced features, you’ll discover new ways to unlock the full potential of this powerful language. Happy coding!
Table of Contents
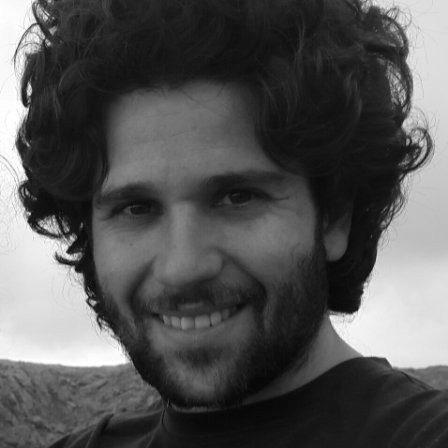
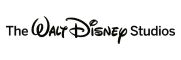