TypeScript and Edge Computing: Processing Data Locally
In an era driven by data, the need for efficient and responsive processing is ever-growing. Edge computing has emerged as a solution that brings computation closer to the data source, reducing latency and enhancing real-time decision-making. TypeScript, a statically typed superset of JavaScript, proves to be an ideal companion for developing edge computing applications. In this blog, we will delve into the world of TypeScript and edge computing, exploring key concepts, benefits, and practical code examples.
Table of Contents
1. Understanding Edge Computing
1.1. What Is Edge Computing?
Edge computing is a distributed computing paradigm that brings computation and data storage closer to the source of data generation. Unlike traditional cloud computing, where data is sent to centralized data centers for processing, edge computing processes data locally, often on devices or servers located at the “edge” of the network. This proximity to data sources reduces latency, improves response times, and enhances overall system performance.
Edge computing is particularly valuable in scenarios where low latency and real-time processing are critical, such as:
1.1.1. Internet of Things (IoT) Applications
Sensors, cameras, and devices generate vast amounts of data that need to be processed locally for real-time decision-making.
1.1.2. Autonomous Vehicles
Edge computing enables vehicles to make split-second decisions based on sensor data without relying on a distant data center.
1.1.3. Industrial Automation
Manufacturing processes benefit from edge computing for precise control and monitoring without network delays.
2. The Role of TypeScript in Edge Computing
TypeScript, known for its strong type system and robust tooling, is an excellent choice for developing edge computing applications. Here’s how TypeScript plays a crucial role in this context:
2.1. Enhanced Reliability
TypeScript’s static typing helps catch errors at compile-time, reducing the likelihood of runtime issues, which is crucial in edge computing where reliability is paramount.
2.2. Tooling Support
TypeScript’s ecosystem offers powerful tools like Visual Studio Code with intelligent autocompletion, debugging, and refactoring capabilities, making development more efficient.
2.3. Code Maintainability
TypeScript’s strict type checking makes code more maintainable, especially in complex edge computing scenarios where code quality is vital.
3. Getting Started with TypeScript and Edge Computing
Let’s dive into the practical aspects of using TypeScript for edge computing. In this section, we’ll walk you through setting up a simple edge computing project and processing data locally using TypeScript.
3.1. Prerequisites
Before we begin, ensure that you have the following prerequisites installed:
- Node.js and npm (Node Package Manager): You can download them from https://nodejs.org/.
- Creating a TypeScript Project
Initialize a Node.js project:
Open your terminal and navigate to your project folder. Run the following command to create a package.json file:
bash npm init -y
Install TypeScript:
Install TypeScript globally by running the following command:
bash npm install -g typescript
Create a TypeScript configuration file:
Run the following command to generate a tsconfig.json file with default settings:
bash tsc --init
Writing Your First TypeScript Code:
Create a file named app.ts in your project directory and add the following code:
typescript // app.ts const message: string = "Hello, Edge Computing!"; console.log(message);
Compile TypeScript to JavaScript:
Compile your TypeScript code into JavaScript using the following command:
bash tsc
This will generate an app.js file in the same directory.
Run Your JavaScript Code:
You can now execute your JavaScript code:
bash node app.js
You should see the output: “Hello, Edge Computing!”
Congratulations! You’ve set up a basic TypeScript project. Now, let’s explore how TypeScript can be leveraged for edge computing.
4. Processing Data Locally with TypeScript
Edge computing often involves collecting data from sensors or devices and processing it locally. Let’s create a simple example of processing sensor data using TypeScript.
4.1. Simulate Sensor Data
To simulate sensor data, we’ll generate random temperature readings. Install the faker package to help with this:
bash npm install faker
Now, modify your app.ts file:
typescript // app.ts import * as faker from 'faker'; function generateTemperature(): number { return faker.datatype.number({ min: -10, max: 40 }); } const temperatureData: number[] = []; for (let i = 0; i < 10; i++) { temperatureData.push(generateTemperature()); } console.log("Sensor Data:", temperatureData);
In this code, we’re using the faker package to generate random temperature readings and storing them in an array.
4.2. Analyze Sensor Data
Now, let’s perform some analysis on the sensor data. We’ll calculate the average temperature:
typescript // app.ts (continued) function calculateAverageTemperature(data: number[]): number { const sum = data.reduce((acc, temperature) => acc + temperature, 0); return sum / data.length; } const averageTemperature = calculateAverageTemperature(temperatureData); console.log("Average Temperature:", averageTemperature.toFixed(2));
This code defines a function calculateAverageTemperature that computes the average temperature from the sensor data array.
4.3. Real-Time Decision-Making
In an edge computing scenario, real-time decision-making is crucial. For instance, we might want to trigger an alert when the temperature exceeds a certain threshold. Let’s add this logic:
typescript // app.ts (continued) const temperatureThreshold = 30; function checkTemperatureThreshold(data: number[]): void { const aboveThreshold = data.some((temperature) => temperature > temperatureThreshold); if (aboveThreshold) { console.log("Temperature exceeds threshold. Alert!"); } else { console.log("Temperature within acceptable range."); } } checkTemperatureThreshold(temperatureData);
Here, we define a threshold of 30°C and check if any temperature reading exceeds this threshold. If so, we trigger an alert.
5. Running the Edge Computing Simulation
Compile your TypeScript code to JavaScript:
bash tsc
Run your JavaScript code:
bash node app.js
You should see the simulated sensor data, the average temperature, and whether the temperature exceeds the threshold.
6. Benefits of TypeScript in Edge Computing
Now that we’ve explored a simple edge computing example using TypeScript, let’s discuss the key benefits of TypeScript in this context:
6.1. Strong Typing
TypeScript’s static typing helps catch type-related errors at compile-time, reducing the risk of runtime failures in edge computing applications where reliability is crucial.
6.2. Improved Code Quality
TypeScript encourages writing clean, organized, and self-documenting code. This is particularly important in edge computing projects where code maintainability is essential.
6.3. Tooling Support
TypeScript’s rich ecosystem includes powerful tools like Visual Studio Code, which offers features like autocompletion and intelligent debugging, enhancing developer productivity.
6.4. Enhanced Safety
In edge computing, safety is paramount. TypeScript’s type system and strict checking provide an extra layer of safety, reducing the chance of vulnerabilities.
6.5. Scalability
TypeScript’s modularity and support for modern JavaScript features make it well-suited for building scalable edge computing applications that can evolve with changing requirements.
Conclusion
Edge computing is revolutionizing the way we process data, bringing real-time processing closer to data sources. TypeScript, with its strong typing, tooling support, and code quality benefits, is an excellent choice for developing edge computing applications. As demonstrated in our example, TypeScript empowers developers to build reliable, maintainable, and safe edge computing solutions that can handle the challenges of processing data locally.
In a world where data-driven decisions are critical, TypeScript and edge computing make a powerful combination, enabling businesses and developers to stay ahead in the rapidly evolving landscape of technology.
Start exploring TypeScript for edge computing today and unlock the potential of processing data locally with efficiency and precision. Your journey to responsive and reliable edge computing applications begins here!
Table of Contents
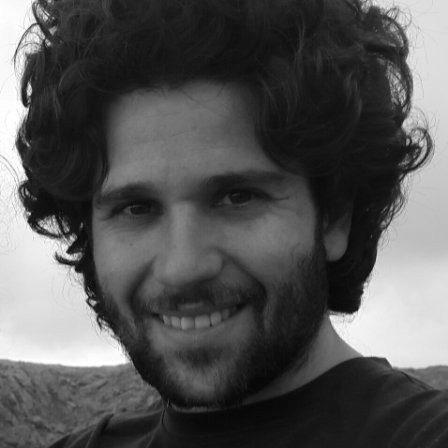
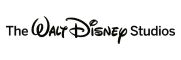