TypeScript and Robotic Process Automation: Automating Tasks
In an era defined by automation and efficiency, Robotic Process Automation (RPA) stands out as a game-changer in streamlining repetitive tasks. But what if we told you there’s a way to take your RPA endeavors to the next level? Enter TypeScript, a statically typed superset of JavaScript that brings a new level of precision and reliability to your automation efforts. In this blog, we’ll explore how TypeScript can supercharge your RPA initiatives by automating tasks with finesse.
Table of Contents
1. Understanding Robotic Process Automation (RPA)
Before we dive into TypeScript’s role in RPA, let’s establish a clear understanding of RPA itself.
1.1. What is Robotic Process Automation (RPA)?
Robotic Process Automation (RPA) refers to the use of software robots or “bots” to automate rule-based, repetitive tasks within business processes. These bots mimic human actions in a digital environment, making them ideal for tasks such as data entry, form filling, and report generation.
RPA has gained immense popularity across industries due to its ability to enhance operational efficiency, reduce errors, and free up human resources for more value-added tasks. However, to achieve these benefits, RPA solutions must be reliable and precise.
1.2. The Need for Precision in RPA
In the world of RPA, precision is paramount. Bots must interact with various applications, websites, and systems with high accuracy to avoid errors and costly mistakes. A minor deviation in a bot’s behavior can lead to data corruption, compliance issues, or operational disruptions.
This is where TypeScript comes into play.
2. TypeScript: The Powerhouse Behind RPA Precision
TypeScript, developed by Microsoft, is a statically typed superset of JavaScript. Its primary goal is to enhance the developer experience by catching type-related errors at compile time rather than runtime, thus making your code more predictable and reliable.
2.1. Advantages of TypeScript in RPA
2.1.1. Type Safety
In RPA development, the ability to catch errors early in the development process is invaluable. TypeScript enforces strict type checking, which means that you can identify and rectify potential issues before they even make it to the bot’s runtime environment.
typescript // TypeScript code sample function add(a: number, b: number): number { return a + b; } const result = add(5, '10'); // Error: Argument of type '"10"' is not assignable to parameter of type 'number'.
In the example above, TypeScript prevents the addition of a string and a number, highlighting the error during development rather than at runtime.
2.1.2. Intelligent Code Completion
TypeScript’s integrated development environment (IDE) support provides intelligent code completion and suggestions. This feature can significantly speed up RPA development by reducing the time spent searching for correct function names, method parameters, and variable types.
2.1.3. Enhanced Documentation
TypeScript encourages developers to provide comprehensive type annotations and documentation. In the context of RPA, this means that your bot code becomes self-explanatory, making it easier for your team to understand, maintain, and collaborate on automation projects.
2.1.4. Improved Refactoring
RPA projects often evolve and require frequent updates. TypeScript simplifies the process of refactoring your codebase, ensuring that changes in one part of your automation workflow don’t inadvertently break other components.
3. TypeScript in Action: Building an RPA Bot
Let’s put TypeScript’s advantages to the test by building a simple RPA bot that automates a common task: extracting data from a website.
typescript import puppeteer from 'puppeteer'; async function scrapeWebsite(url: string): Promise<string> { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto(url); const data = await page.evaluate(() => { const title = document.querySelector('h1')?.textContent; const paragraph = document.querySelector('p')?.textContent; return { title, paragraph }; }); await browser.close(); return JSON.stringify(data); } const websiteData = await scrapeWebsite('https://example.com'); console.log(websiteData);
In this example, we use TypeScript with Puppeteer, a headless browser automation library, to scrape data from a website. TypeScript ensures that we pass the correct types and handle potential errors gracefully.
4. Real-World Applications of TypeScript in RPA
Now that we’ve seen how TypeScript can enhance precision and reliability in RPA, let’s explore some real-world applications of this powerful combination.
4.1. Data Entry Automation
RPA bots are often used to automate data entry tasks, such as updating customer records in a CRM system. TypeScript’s type safety and intelligent code completion make it easier to develop bots that can handle diverse data formats and ensure accurate data entry.
4.2. Financial Reporting
In the finance industry, RPA is employed to generate financial reports, reconcile transactions, and validate data across multiple systems. TypeScript’s strong typing helps prevent data discrepancies and errors in financial calculations.
4.3. HR Onboarding
Automating HR onboarding processes, including document generation, employee record updates, and communication with new hires, can be error-prone without TypeScript. TypeScript ensures that the automation workflow runs smoothly, reducing HR department workload.
4.4. Quality Assurance Testing
RPA can be used for quality assurance testing, where bots perform repetitive testing tasks across various devices and platforms. TypeScript’s robust error-checking capabilities help ensure that the testing results are accurate and reliable.
5. Best Practices for Using TypeScript in RPA
To make the most of TypeScript in your RPA projects, consider the following best practices:
5.1. Comprehensive Type Definitions
Invest time in creating comprehensive type definitions for your automation scripts. Well-defined types make your code more self-explanatory and help catch errors early.
5.2. Regular Testing
Perform thorough testing of your RPA bots with different scenarios and edge cases. TypeScript’s type checking is a valuable tool, but real-world testing is essential to validate your automation.
5.3. Version Control
Use version control systems like Git to track changes in your TypeScript-based RPA projects. This allows you to roll back to previous versions if issues arise and facilitates collaboration among team members.
5.4. Documentation
Document your RPA projects extensively. Describe the purpose of your bots, the data they handle, and any potential limitations. TypeScript’s support for inline documentation makes this process easier.
Conclusion
Robotic Process Automation (RPA) has revolutionized how businesses handle repetitive tasks, but precision and reliability are essential for its success. TypeScript, with its type safety, intelligent code completion, and documentation support, is the perfect companion for RPA development.
By integrating TypeScript into your RPA projects, you can automate tasks with confidence, knowing that your bots will perform with accuracy and efficiency. Whether you’re automating data entry, financial reporting, HR processes, or quality assurance testing, TypeScript empowers your RPA efforts to reach new heights.
So, as you embark on your journey to supercharge your RPA initiatives, remember that TypeScript is your ally in achieving automation excellence. It’s time to let the bots do the repetitive work while you focus on innovation and growth. Happy automating!
Table of Contents
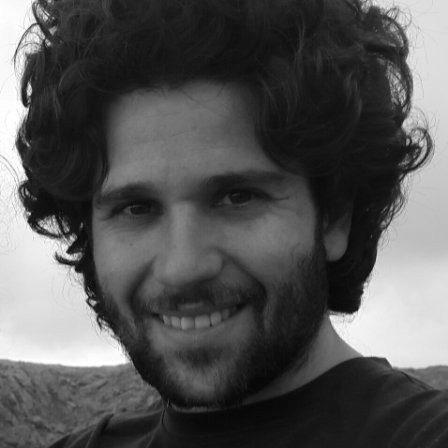
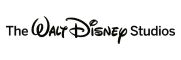