TypeScript and Cryptocurrency: Building Blockchain Apps
The cryptocurrency revolution has reshaped the financial landscape, offering new opportunities and challenges. Blockchain technology underpins this digital revolution, and one of the best tools for building blockchain applications is TypeScript. In this comprehensive guide, we’ll explore how TypeScript can be leveraged to create robust and secure cryptocurrency projects.
Table of Contents
1. Why TypeScript for Blockchain Development?
Blockchain development demands precision, security, and scalability. TypeScript, a statically typed superset of JavaScript, provides several advantages for building blockchain applications:
1.1. Strong Typing for Enhanced Security
TypeScript’s static typing system allows you to catch type-related errors at compile-time, reducing the risk of runtime errors and vulnerabilities in your blockchain code. With cryptocurrencies involved, security is paramount.
1.2. Enhanced Code Maintainability
As blockchain projects grow in complexity, maintaining clean and organized code becomes crucial. TypeScript’s strong typing and support for modules and classes make it easier to manage and refactor code as your project evolves.
1.3. Intellisense and Tooling Support
TypeScript integrates seamlessly with modern IDEs and code editors, providing features like autocompletion, code navigation, and real-time error checking. This aids in faster development and debugging, crucial when dealing with financial systems.
1.4. Compatibility with Existing JavaScript Libraries
TypeScript allows you to leverage existing JavaScript libraries, giving you access to a vast ecosystem of blockchain-related tools and frameworks. This reduces development time and effort.
Now, let’s dive into the essential concepts and best practices for building blockchain applications with TypeScript.
2. Setting Up Your TypeScript Environment
Before you start coding, you’ll need to set up your TypeScript development environment. Ensure you have Node.js and npm (Node Package Manager) installed. Then, create a new TypeScript project and initialize it with npm:
bash npm init -y npm install typescript --save-dev
Next, create a tsconfig.json file in your project’s root directory to configure TypeScript:
json { "compilerOptions": { "target": "ES6", "module": "commonjs", "outDir": "./dist", "rootDir": "./src", "strict": true }, "include": ["src/**/*.ts"], "exclude": ["node_modules"] }
This configuration sets TypeScript to compile your code to ES6, which is commonly used in modern JavaScript environments. You can adjust these settings based on your project’s requirements.
3. Smart Contracts with TypeScript
In blockchain development, smart contracts are self-executing agreements with predefined rules and conditions. Ethereum, one of the most popular blockchain platforms, supports the development of smart contracts using a programming language called Solidity. However, TypeScript can also be used to write smart contracts, thanks to projects like Hardhat and ethers.js.
3.1. Installing Hardhat
Hardhat is a development environment for Ethereum that supports TypeScript out of the box. Install it globally on your system:
bash npm install -g hardhat
Create a new directory for your project and navigate to it in your terminal.
3.2. Initializing Your Hardhat Project
Initialize your Hardhat project using the following command:
bash npx hardhat
This command will guide you through the setup process and create a basic project structure for your Ethereum-based blockchain application.
3.3. Writing a Smart Contract in TypeScript
Inside your Hardhat project directory, navigate to the contracts folder. Create a new TypeScript file for your smart contract, for example, MyContract.ts. Here’s a simple example of a smart contract written in TypeScript:
typescript // MyContract.ts // SPDX-License-Identifier: MIT pragma solidity ^0.8.0; contract MyContract { uint256 public value; constructor() { value = 0; } function setValue(uint256 _newValue) public { value = _newValue; } }
This contract allows you to store and update a single integer value.
3.4. Compiling and Deploying the Smart Contract
Compile your TypeScript smart contract using the following Hardhat command:
bash npx hardhat compile
Once compiled, you can deploy your contract to an Ethereum network of your choice. For local development, you can use Hardhat’s built-in Ethereum network. To deploy the contract, you’ll need to write a deployment script in TypeScript. Here’s an example script:
typescript // deploy.ts import { ethers } from 'hardhat'; async function main() { const [deployer] = await ethers.getSigners(); console.log(`Deploying contract with address: ${deployer.address}`); const MyContract = await ethers.getContractFactory('MyContract'); const myContract = await MyContract.deploy(); console.log(`Contract deployed to address: ${myContract.address}`); } main() .then(() => process.exit(0)) .catch(error => { console.error(error); process.exit(1); });
Run the deployment script using the following command:
bash npx hardhat run scripts/deploy.ts
Your smart contract is now deployed to the local Ethereum network.
4. Building a Cryptocurrency Wallet
A cryptocurrency wallet is a fundamental tool for interacting with blockchain networks. TypeScript can be used to build both web-based and mobile wallets.
4.1. Setting Up a Web-Based Wallet with TypeScript
For web-based wallets, you can use popular JavaScript frameworks like React and TypeScript to create a user-friendly interface. Here’s a basic example of a TypeScript-based cryptocurrency wallet using React:
4.1.1. Install Dependencies
Create a new React project with TypeScript using Create React App:
bash npx create-react-app my-crypto-wallet --template typescript
Install web3.js, a JavaScript library for interacting with Ethereum:
bash npm install web3
4.1.2. Connecting to Ethereum
In your React components, you can connect to an Ethereum network using web3.js. Here’s a simple example of connecting to the Ethereum Rinkeby test network and fetching an account’s balance:
tsx // src/components/Wallet.tsx import React, { useEffect, useState } from 'react'; import Web3 from 'web3'; const Wallet: React.FC = () => { const [account, setAccount] = useState<string>(''); const [balance, setBalance] = useState<string>(''); useEffect(() => { const loadBlockchainData = async () => { if (window.ethereum) { const web3 = new Web3(window.ethereum); const accounts = await web3.eth.getAccounts(); setAccount(accounts[0]); const ethBalance = await web3.eth.getBalance(accounts[0]); setBalance(web3.utils.fromWei(ethBalance, 'ether')); } }; loadBlockchainData(); }, []); return ( <div> <h1>Crypto Wallet</h1> <p>Account: {account}</p> <p>Balance: {balance} ETH</p> </div> ); }; export default Wallet;
This component fetches the account’s balance from the Ethereum network and displays it in a React application.
4.2. Building a Mobile Wallet with TypeScript
For mobile wallets, you can use TypeScript in combination with popular mobile app development frameworks like React Native or Flutter. Here, we’ll provide a brief overview of building a mobile wallet with React Native and TypeScript.
4.2.1. Install Dependencies
Create a new React Native project with TypeScript:
bash npx react-native init MyCryptoWallet --template react-native-template-typescript
Install web3.js for React Native:
bash npm install web3
4.2.2. Connecting to Ethereum
In a React Native component, you can connect to an Ethereum network in a similar way as in the web-based wallet example. Here’s a simplified example of fetching an account’s balance:
tsx // src/components/Wallet.tsx import React, { useEffect, useState } from 'react'; import Web3 from 'web3'; const Wallet: React.FC = () => { const [account, setAccount] = useState<string>(''); const [balance, setBalance] = useState<string>(''); useEffect(() => { const loadBlockchainData = async () => { const provider = new Web3.providers.HttpProvider('https://rinkeby.infura.io/v3/YOUR_INFURA_PROJECT_ID'); const web3 = new Web3(provider); const accounts = await web3.eth.getAccounts(); setAccount(accounts[0]); const ethBalance = await web3.eth.getBalance(accounts[0]); setBalance(web3.utils.fromWei(ethBalance, 'ether')); }; loadBlockchainData(); }, []); return ( <div> <h1>Crypto Wallet</h1> <p>Account: {account}</p> <p>Balance: {balance} ETH</p> </div> ); }; export default Wallet;
This React Native component fetches the account’s balance from the Ethereum Rinkeby test network and displays it in a mobile app.
5. Handling Cryptocurrency Transactions
A significant part of blockchain development is handling cryptocurrency transactions securely. TypeScript’s strong typing and error-checking capabilities are crucial for this task.
5.1. Creating a Transaction in TypeScript
To create a cryptocurrency transaction, you need to use a library like web3.js to interact with the Ethereum network. Here’s an example of sending Ether from one account to another in TypeScript:
typescript import Web3 from 'web3'; async function sendEther(senderAddress: string, receiverAddress: string, privateKey: string, amount: number) { const providerUrl = 'https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'; // Use the Ethereum network of your choice const web3 = new Web3(new Web3.providers.HttpProvider(providerUrl)); // Unlock the sender's account await web3.eth.accounts.wallet.add(privateKey); // Create a transaction object const transaction = { from: senderAddress, to: receiverAddress, value: web3.utils.toWei(amount.toString(), 'ether'), }; // Send the transaction const receipt = await web3.eth.sendTransaction(transaction); console.log(`Transaction hash: ${receipt.transactionHash}`); }
In this example, we create a TypeScript function that sends Ether from one account to another. It unlocks the sender’s account using a private key, creates a transaction object, and sends the transaction to the Ethereum network.
5.2. Error Handling and Security
TypeScript’s static typing helps catch errors early in the development process, reducing the risk of transaction errors that could lead to financial losses. Additionally, handling sensitive information like private keys requires utmost security measures, which TypeScript can assist in implementing.
6. Testing Your Blockchain Application
Testing is a critical aspect of blockchain development to ensure the reliability and security of your applications. TypeScript makes it easier to write robust tests for your blockchain code.
6.1. Unit Testing with TypeScript
You can use TypeScript in combination with testing frameworks like Mocha and Chai to write unit tests for your blockchain smart contracts. Here’s a basic example:
typescript import { expect } from 'chai'; import { ethers } from 'hardhat'; describe('MyContract', function () { it('Should set the value', async function () { const MyContract = await ethers.getContractFactory('MyContract'); const myContract = await MyContract.deploy(); await myContract.setValue(42); const value = await myContract.value(); expect(value).to.equal(42); }); });
In this example, we use TypeScript to write a unit test for the setValue function of the MyContract smart contract.
6.2. Integration and End-to-End Testing
For web-based or mobile wallets, you can also write integration and end-to-end tests in TypeScript using frameworks like Jest or Detox for React Native. These tests help ensure that the entire application, including user interactions, works as expected.
Conclusion
TypeScript is a powerful language for building blockchain applications in the world of cryptocurrency. Its strong typing, code maintainability, and tooling support make it an excellent choice for developing secure and efficient blockchain solutions. Whether you’re creating smart contracts, cryptocurrency wallets, or handling transactions, TypeScript’s capabilities can help you navigate the complex and rapidly evolving landscape of cryptocurrency development. Start harnessing the potential of TypeScript today to build the next generation of blockchain applications.
In this comprehensive guide, we’ve covered essential topics such as setting up your TypeScript environment, creating smart contracts, building cryptocurrency wallets, handling transactions, and testing your blockchain application. Armed with this knowledge, you’re ready to embark on your journey into the exciting world of TypeScript and cryptocurrency development. Happy coding!
Are you interested in exploring more about TypeScript and cryptocurrency development? Let us know in the comments below!
Table of Contents
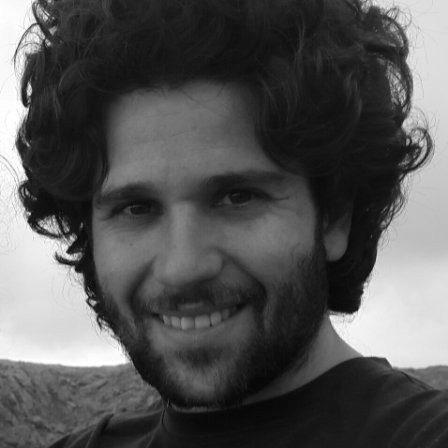
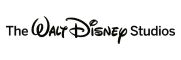