TypeScript and Agile Development: Speed and Flexibility
In the fast-paced world of software development, agility is key. Being able to adapt to changing requirements and deliver high-quality code quickly is essential. TypeScript and Agile Development are two powerful tools that, when used in tandem, can supercharge your development process. In this blog, we’ll explore how TypeScript and Agile Development complement each other, providing the speed and flexibility you need to succeed in today’s competitive landscape.
Table of Contents
1. TypeScript: A Strong Typing System for JavaScript
1.1. What is TypeScript?
Before we dive into the Agile Development aspect, let’s briefly explore TypeScript. TypeScript is a statically typed superset of JavaScript. It’s designed to help developers catch errors early, make code more readable, and enhance productivity.
With TypeScript, you can:
Catch Errors at Compile Time: TypeScript’s static type checking ensures that common runtime errors are caught during compilation, reducing bugs and enhancing code quality.
Improve Code Readability: TypeScript adds type annotations, making your code easier to understand and maintain, especially in large codebases.
Enhance Tooling Support: TypeScript provides excellent tooling support in popular IDEs, enabling features like autocompletion, refactoring, and better documentation generation.
2. How TypeScript Boosts Agile Development
Now, let’s see how TypeScript integrates seamlessly with Agile Development methodologies to enhance speed and flexibility in your projects.
2.1. Faster Iterations
Agile methodologies emphasize frequent iterations and continuous improvement. TypeScript accelerates this process by reducing the time spent debugging and fixing runtime errors. With static type checking, you catch and address issues during development, leading to faster and smoother iterations.
typescript // Before TypeScript function add(a, b) { return a + b; } // After TypeScript function add(a: number, b: number): number { return a + b; }
In the above example, TypeScript’s type annotations clearly define the expected input and output types, reducing the chance of runtime errors during iterations.
2.2. Enhanced Collaboration
Collaboration is at the heart of Agile Development, and TypeScript promotes it by providing a common language for developers, testers, and stakeholders. With TypeScript’s type annotations, everyone involved in the project can understand the codebase more easily, leading to smoother discussions and faster decision-making.
2.3. Improved Refactoring
Agile teams often need to adapt to changing requirements. TypeScript’s refactoring tools make it easier to modify code without introducing new bugs. You can confidently make changes, knowing that TypeScript will help you catch any issues early in the development cycle.
typescript // Before Refactoring function calculateTotal(items) { // Calculate total here } // After Refactoring function calculateTotal(items: number[]): number { // Calculate total here }
TypeScript ensures that the function’s signature matches the expected input and output, reducing the risk of breaking changes during refactoring.
3. Agile Development: Embracing Change
3.1. What is Agile Development?
Agile Development is a set of methodologies and principles that prioritize flexibility, collaboration, and customer feedback. The Agile approach emphasizes:
Iterative Development: Projects are divided into small, manageable iterations, allowing for frequent updates and adjustments.
Customer-Centric: Agile teams prioritize customer feedback and adapt to changing requirements.
Collaboration: Cross-functional teams work closely together to deliver working software.
Now, let’s see how Agile Development complements TypeScript.
4. How Agile Development Enhances TypeScript Projects
4.1. Continuous Feedback
Agile methodologies encourage continuous feedback from stakeholders, including end-users. TypeScript’s strong typing helps developers deliver more reliable code, reducing the risk of critical issues reaching the end-users. This alignment ensures that the feedback process is more about improving features than fixing bugs.
4.2. Rapid Prototyping
Agile Development allows teams to quickly create prototypes or Minimum Viable Products (MVPs). TypeScript’s static typing provides a safety net during rapid development, ensuring that even prototypes are relatively bug-free. This means that you can create and test ideas more rapidly, increasing your chances of success.
4.3. Adaptation to Changing Requirements
In the Agile world, change is inevitable. TypeScript’s type system makes it easier to adapt to these changes without breaking existing functionality. The type annotations serve as documentation, helping developers understand how different parts of the codebase interact. This understanding is crucial when responding to new requirements or altering existing ones.
5. Bringing It All Together: A Case Study
Let’s take a real-world example to see how TypeScript and Agile Development work together in practice. Imagine a team tasked with building an e-commerce website.
Sprint 1: Setting Up the Project
In the first sprint, the team sets up the project and defines the initial data structures and components. TypeScript helps identify potential issues early.
typescript // Define the Product interface interface Product { id: number; name: string; price: number; }
Sprint 2: Implementing Product Listing
In this sprint, the team focuses on building the product listing page. TypeScript ensures that the data flowing through the components is of the correct type, reducing runtime errors.
typescript // ProductListing.tsx import React from 'react'; interface ProductListingProps { products: Product[]; } const ProductListing: React.FC<ProductListingProps> = ({ products }) => { return ( <div> {products.map(product => ( <div key={product.id}> <h3>{product.name}</h3> <p>${product.price}</p> </div> ))} </div> ); };
Sprint 3: Adding a Shopping Cart
During this sprint, the team adds a shopping cart feature. TypeScript ensures that the cart functions work correctly and that the types of items in the cart are well-defined.
typescript // ShoppingCart.tsx import React from 'react'; interface ShoppingCartProps { items: Product[]; } const ShoppingCart: React.FC<ShoppingCartProps> = ({ items }) => { return ( <div> <h2>Shopping Cart</h2> <ul> {items.map(item => ( <li key={item.id}>{item.name} - ${item.price}</li> ))} </ul> </div> ); };
Sprint 4: User Authentication
In this sprint, the team adds user authentication. TypeScript ensures that user-related data is handled securely and that the authentication process is well-typed.
typescript // AuthService.ts class AuthService { async login(username: string, password: string): Promise<User | null> { // Authentication logic here } }
Throughout these sprints, Agile Development principles guide the team, allowing them to adapt to changing requirements, gather feedback, and deliver value to the customer in small, frequent increments. TypeScript’s static typing keeps the codebase robust and helps avoid costly bugs.
6. Challenges and Considerations
While TypeScript and Agile Development are a powerful combination, it’s essential to consider potential challenges:
6.1. Learning Curve
Adopting TypeScript may require developers to learn new concepts and syntax. Agile teams need to allocate time for training and gradual adoption.
6.2. Balance Between Flexibility and Rigidity
Striking the right balance between adapting to change (Agile) and enforcing strict typing (TypeScript) can be challenging. Teams must carefully consider when and where to apply TypeScript’s type annotations.
6.3. Tooling and Ecosystem
Ensure that the tools and libraries you use are compatible with TypeScript. While TypeScript has excellent support, some third-party packages may require additional configuration.
Conclusion
TypeScript and Agile Development are a dynamic duo that can significantly enhance your software development projects. TypeScript’s strong typing system catches errors early, improves code quality, and enhances collaboration. Agile Development methodologies prioritize flexibility, customer feedback, and rapid iterations.
By combining TypeScript and Agile Development, your team can deliver high-quality software faster, adapt to changing requirements, and respond to customer feedback more effectively. While there may be challenges along the way, the benefits of this synergy are well worth the effort.
So, embrace TypeScript, embrace Agile, and supercharge your development process to meet the demands of today’s software landscape.
Remember, in the ever-evolving world of technology, agility and adaptability are your most potent weapons.
Table of Contents
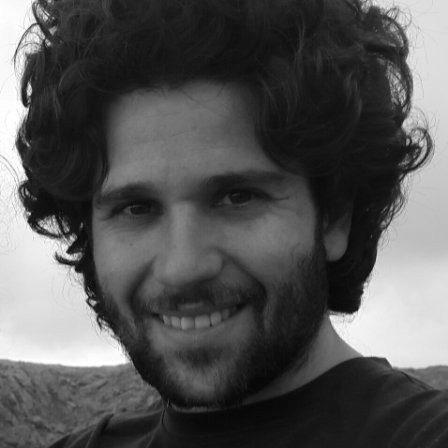
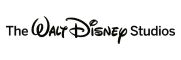