TypeScript and AR/VR Development: Bridging the Virtual Gap
The world of technology is advancing at an unprecedented pace, with one of the most exciting frontiers being Augmented Reality (AR) and Virtual Reality (VR). These immersive technologies have the potential to transform the way we interact with the digital world, blurring the line between reality and virtuality. TypeScript, a statically typed superset of JavaScript, has emerged as a powerful tool for developers in this space, helping bridge the gap between the physical and the virtual. In this blog, we’ll explore how TypeScript is revolutionizing AR/VR development, enabling developers to create more robust, efficient, and engaging virtual experiences.
Table of Contents
1. Understanding TypeScript: A Brief Overview
1.1. What Is TypeScript?
TypeScript is a statically typed language developed by Microsoft. It is built on top of JavaScript and adds static typing to the language, making it more reliable and easier to maintain. TypeScript code is transpiled into JavaScript, which means it can run on any platform that supports JavaScript.
1.2. Why TypeScript for AR/VR?
When it comes to AR/VR development, TypeScript offers several advantages:
- Type Safety: TypeScript provides a level of type safety that JavaScript lacks. This is crucial for AR/VR applications where performance and reliability are paramount.
- Tooling Support: TypeScript is supported by a wide range of development tools, including IDEs like Visual Studio Code. This makes it easier for developers to write, debug, and maintain code.
- Scalability: AR/VR applications often involve complex logic and interactions. TypeScript’s strong typing system helps manage the complexity and scale of these applications.
- Readability: TypeScript’s syntax and strong typing make code more readable and self-documenting, which is essential for collaboration in large development teams.
- Community and Ecosystem: TypeScript has a thriving community and a vast ecosystem of libraries and frameworks, making it an ideal choice for AR/VR development.
2. Building the Foundation: TypeScript and 3D Graphics
AR/VR experiences heavily rely on 3D graphics to create immersive environments. TypeScript seamlessly integrates with popular 3D libraries and frameworks, such as Three.js, Babylon.js, and A-Frame, making it an excellent choice for building the foundation of your AR/VR project.
Example: Using TypeScript with Three.js
Let’s take a look at a simple example of how TypeScript can be used with Three.js to create a 3D scene:
typescript import * as THREE from 'three'; // Create a scene const scene = new THREE.Scene(); // Create a camera const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); camera.position.z = 5; // Create a renderer const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.body.appendChild(renderer.domElement); // Create a cube const geometry = new THREE.BoxGeometry(); const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 }); const cube = new THREE.Mesh(geometry, material); scene.add(cube); // Animation loop const animate = () => { requestAnimationFrame(animate); // Rotate the cube cube.rotation.x += 0.01; cube.rotation.y += 0.01; renderer.render(scene, camera); }; animate();
In this example, we’ve used TypeScript to set up a basic Three.js scene with a rotating cube. TypeScript’s type checking ensures that we’re using Three.js objects and methods correctly, reducing the likelihood of runtime errors.
3. Managing State and Interactivity
AR/VR applications often involve complex state management and user interactions. TypeScript’s strong typing and object-oriented features are a great fit for handling these challenges.
Example: State Management with TypeScript
Consider a scenario where you want to manage the state of a virtual car in an AR simulation. TypeScript can help you define a clear and type-safe state structure:
typescript // Define the car state interface CarState { speed: number; position: { x: number; y: number; z: number; }; isEngineOn: boolean; } // Initialize the car state const car: CarState = { speed: 0, position: { x: 0, y: 0, z: 0 }, isEngineOn: false, }; // Update the car state function accelerate(car: CarState, amount: number): CarState { if (car.isEngineOn) { car.speed += amount; } return car; } function turnOnEngine(car: CarState): CarState { car.isEngineOn = true; return car; } // Usage car = turnOnEngine(car); car = accelerate(car, 10);
In this example, TypeScript ensures that we’re working with the correct properties and types when managing the car’s state. This level of type safety is invaluable in complex AR/VR applications where state management is critical.
4. Enhancing User Interfaces with TypeScript
User interfaces in AR/VR applications play a pivotal role in providing immersive experiences. TypeScript can be leveraged to build rich and interactive UIs.
Example: Creating a VR Menu
Let’s create a simple VR menu using TypeScript and A-Frame:
typescript import 'aframe'; import { Entity, Scene } from 'aframe-react'; import React from 'react'; const VrMenu: React.FC = () => { return ( <Scene> <Entity primitive="a-camera"> <Entity primitive="a-plane" color="blue" width="2" height="1" position="0 0 -5" onClick={() => console.log('Button clicked!')} > <Entity text="value: Click me" position="0 0 0" scale="0.5 0.5 0.5" /> </Entity> </Entity> </Scene> ); }; export default VrMenu;
Here, we’ve used TypeScript in conjunction with A-Frame to create a VR menu. TypeScript ensures that event handlers, properties, and components are correctly typed, making the code more robust.
5. Optimizing Performance with TypeScript
Performance is a critical aspect of AR/VR development, as these applications often require real-time rendering and interactions. TypeScript can help optimize code for better performance.
Example: Optimizing AR Marker Detection
Consider an AR application that uses markers for object recognition. TypeScript can assist in writing efficient code for marker detection:
typescript // Define a marker interface interface Marker { id: number; position: { x: number; y: number; z: number; }; } // Function to detect markers function detectMarkers(markers: Marker[], cameraPosition: { x: number; y: number; z: number }) { const detectedMarkers: Marker[] = []; for (const marker of markers) { // Calculate the distance between the marker and the camera const distance = Math.sqrt( Math.pow(cameraPosition.x - marker.position.x, 2) + Math.pow(cameraPosition.y - marker.position.y, 2) + Math.pow(cameraPosition.z - marker.position.z, 2) ); // If the marker is within a certain range, consider it detected if (distance < 2) { detectedMarkers.push(marker); } } return detectedMarkers; }
In this example, TypeScript helps optimize marker detection by ensuring that the marker data and camera position have the correct types. This can lead to significant performance improvements in AR applications.
6. Challenges and Future Developments
While TypeScript offers numerous benefits for AR/VR development, it’s essential to acknowledge the challenges and ongoing developments in this field:
- Complexity: AR/VR development is inherently complex due to the integration of hardware, 3D graphics, and real-time interactions. TypeScript helps manage this complexity but does not eliminate it entirely.
- Performance: Achieving high-performance AR/VR experiences remains a challenge, especially on mobile devices. Developers must continually optimize their code to deliver smooth interactions.
- Cross-Platform Compatibility: Ensuring that AR/VR applications work seamlessly across different devices and platforms is a persistent challenge. TypeScript’s cross-compilation to JavaScript can help, but it’s not a silver bullet.
- WebXR and WebAssembly: The WebXR API and WebAssembly are evolving to improve AR/VR capabilities in web browsers. TypeScript is likely to play a significant role in this evolution.
Conclusion
As technology continues to advance, TypeScript is becoming an indispensable tool for AR/VR development. Its strong typing, tooling support, and ecosystem make it well-suited for creating immersive and performant virtual experiences. By embracing TypeScript, developers can bridge the gap between the virtual and physical worlds, ushering in a new era of AR/VR innovation.
If you’re passionate about creating immersive experiences, TypeScript is a skill worth mastering. It empowers you to push the boundaries of what’s possible in the world of AR and VR, bringing your wildest virtual dreams to life. So, dive in, experiment, and be part of the exciting future of AR/VR development. The virtual gap is waiting for you to bridge it.
Table of Contents
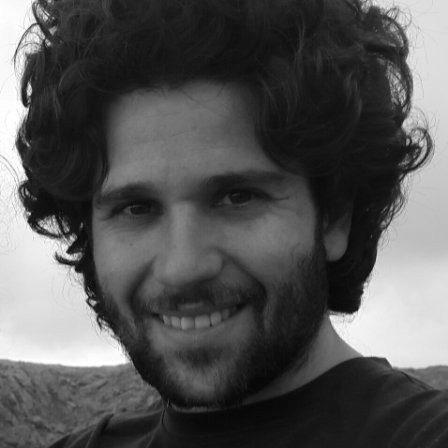
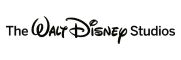