Asynchronous Programming in TypeScript
In the fast-paced world of modern web development, applications are expected to be highly responsive, handle multiple tasks concurrently, and scale effortlessly. Asynchronous programming plays a pivotal role in achieving these goals. In this blog, we’ll dive deep into the world of asynchronous programming in TypeScript, understanding its importance and exploring various techniques to harness its potential. Whether you’re a beginner or an experienced developer, this comprehensive guide will provide insights and practical code samples to level up your TypeScript asynchronous programming skills.
1. What is Asynchronous Programming?
Asynchronous programming is a programming paradigm that allows tasks to execute independently of the main program flow. Instead of blocking the execution of code until a task completes, asynchronous programming enables the program to continue processing other tasks while waiting for the completion of asynchronous operations, such as file I/O, network requests, or database queries. This non-blocking nature ensures better resource utilization and enhanced application responsiveness.
2. Why Asynchronous Programming in TypeScript Matters?
In TypeScript, asynchronous programming is crucial for building performant web applications. By leveraging asynchronous techniques, you can ensure that your application doesn’t freeze or become unresponsive during time-consuming operations. Moreover, as modern applications deal with numerous concurrent tasks, asynchronous programming is essential for efficient resource utilization and scalability.
3. Callbacks: The Foundation of Asynchronous Programming
In the early days of JavaScript and TypeScript, callbacks were the primary means of handling asynchronous tasks. A callback is a function that is passed as an argument to another function and executed later when the latter has completed its task. However, handling multiple callbacks can lead to a phenomenon known as “Callback Hell,” where deeply nested callbacks make the code difficult to read and maintain.
typescript function fetchData(url: string, callback: (data: any, error?: Error) => void) { // Simulating a network request delay setTimeout(() => { if (Math.random() < 0.8) { callback({ name: "John Doe", age: 30 }); } else { callback(undefined, new Error("Failed to fetch data.")); } }, 1000); } // Using the fetchData function with a callback fetchData("https://api.example.com/data", (data, error) => { if (error) { console.error("Error:", error.message); } else { console.log("Data:", data); } });
4. Promises: A Step Towards Cleaner Asynchronous Code
Promises were introduced as a solution to the issues posed by nested callbacks. A Promise represents the eventual completion (or failure) of an asynchronous operation, and it allows us to attach callback functions to handle the results when the Promise is fulfilled or rejected.
typescript function fetchData(url: string): Promise<any> { return new Promise((resolve, reject) => { // Simulating a network request delay setTimeout(() => { if (Math.random() < 0.8) { resolve({ name: "John Doe", age: 30 }); } else { reject(new Error("Failed to fetch data.")); } }, 1000); }); } // Using the fetchData function with Promises fetchData("https://api.example.com/data") .then((data) => console.log("Data:", data)) .catch((error) => console.error("Error:", error.message));
5. Introducing Async/Await: Simplifying Asynchronous Code
Async/Await is a syntactic improvement over Promises, making asynchronous code more readable and resembling synchronous code. It allows developers to write asynchronous code that looks like regular synchronous code, making it easier to understand and maintain.
typescript function fetchData(url: string): Promise<any> { return new Promise((resolve, reject) => { // Simulating a network request delay setTimeout(() => { if (Math.random() < 0.8) { resolve({ name: "John Doe", age: 30 }); } else { reject(new Error("Failed to fetch data.")); } }, 1000); }); } // Using the fetchData function with Async/Await async function getData() { try { const data = await fetchData("https://api.example.com/data"); console.log("Data:", data); } catch (error) { console.error("Error:", error.message); } } getData();
6. Throttling and Debouncing Asynchronous Tasks
Throttling and debouncing are techniques used to control the rate at which certain asynchronous tasks are executed, especially when they are triggered frequently.
6.1 Avoiding Overwhelmed APIs with Throttling
Throttling limits the number of times a function is executed within a specific time interval. It ensures that the function is called at a controlled rate, preventing an API from being overwhelmed with too many requests.
typescript function throttle(func: (...args: any[]) => void, delay: number): (...args: any[]) => void { let isThrottled = false; return function (...args: any[]) { if (!isThrottled) { isThrottled = true; func(...args); setTimeout(() => { isThrottled = false; }, delay); } }; } // Usage of throttle to limit the frequency of API calls const throttledFetchData = throttle(fetchData, 1000); // This function will be called at most once every second throttledFetchData("https://api.example.com/data"); throttledFetchData("https://api.example.com/data");
6.2 Debouncing: Reducing Unnecessary Calls
Debouncing prevents a function from being executed until a certain amount of time has passed since the last time it was invoked. This is particularly useful when dealing with events that trigger frequent calls (e.g., window resize or keystrokes).
typescript function debounce(func: (...args: any[]) => void, delay: number): (...args: any[]) => void { let timeoutId: NodeJS.Timeout; return function (...args: any[]) { clearTimeout(timeoutId); timeoutId = setTimeout(() => func(...args), delay); }; } // Usage of debounce to handle search input events const searchHandler = debounce((query: string) => { // Perform search operation with the provided query }, 500); // This function will be called only once, 500ms after the last call searchHandler("typescript"); searchHandler("asynchronous");
7. Concurrent Asynchronous Tasks with Promise.all
Promise.all allows multiple asynchronous tasks to be executed concurrently, waiting for all of them to complete before proceeding.
7.1 Running Multiple Tasks in Parallel
typescript function fetchUserData(): Promise<any> { return fetchData("https://api.example.com/user"); } function fetchPostsData(): Promise<any> { return fetchData("https://api.example.com/posts"); } async function fetchAllData() { try { const [userData, postsData] = await Promise.all([fetchUserData(), fetchPostsData()]); console.log("User Data:", userData); console.log("Posts Data:", postsData); } catch (error) { console.error("Error:", error.message); } } fetchAllData();
7.2 Handling Errors in Promise.all
typescript async function fetchAllData() { try { const [userData, postsData] = await Promise.all([fetchUserData(), fetchPostsData()]); console.log("User Data:", userData); console.log("Posts Data:", postsData); } catch (errors) { for (const error of errors) { console.error("Error:", error.message); } } } fetchAllData();
8. Dealing with Asynchronous Iterations
Asynchronous iteration is a technique to handle collections of Promises or asynchronous data, allowing you to iterate over them sequentially or concurrently.
8.1 The Role of for-await-of
The for-await-of loop is used to iterate over asynchronous iterators, allowing us to work with asynchronous data in a synchronous-looking manner.
typescript async function fetchUserData(userId: number): Promise<any> { return fetchData(`https://api.example.com/user/${userId}`); } const userIds = [1, 2, 3, 4, 5]; async function fetchAllUsersData() { for await (const userId of userIds) { const userData = await fetchUserData(userId); console.log("User Data:", userData); } } fetchAllUsersData();
8.2 Combining with Promise.all for Advanced Use Cases
Combining for-await-of with Promise.all can lead to more advanced use cases, such as concurrent fetching of multiple users’ data.
typescript async function fetchAllUsersData() { const userPromises = userIds.map((userId) => fetchUserData(userId)); for await (const userData of Promise.all(userPromises)) { console.log("User Data:", userData); } } fetchAllUsersData();
9. Asynchronous APIs and Fetching Data in TypeScript
Fetching data from external APIs is a common scenario in web development. TypeScript provides various tools to handle asynchronous API calls effectively.
9.1 Making HTTP Requests with Fetch API
The Fetch API is a modern way to make asynchronous HTTP requests in the browser, and it returns a Promise that resolves to the Response object.
typescript async function fetchData(url: string): Promise<any> { const response = await fetch(url); if (!response.ok) { throw new Error(`Failed to fetch data: ${response.status} ${response.statusText}`); } return response.json(); } // Using the fetchData function with Fetch API async function getData() { try { const data = await fetchData("https://api.example.com/data"); console.log("Data:", data); } catch (error) { console.error("Error:", error.message); } } getData();
9.2 Handling Responses with Promises and Async/Await
typescript async function fetchData(url: string): Promise<any> { const response = await fetch(url); if (!response.ok) { throw new Error(`Failed to fetch data: ${response.status} ${response.statusText}`); } return response.json(); } // Using the fetchData function with Promises fetchData("https://api.example.com/data") .then((data) => console.log("Data:", data)) .catch((error) => console.error("Error:", error.message)); // Using the fetchData function with Async/Await async function getData() { try { const data = await fetchData("https://api.example.com/data"); console.log("Data:", data); } catch (error) { console.error("Error:", error.message); } } getData();
10. Working with Asynchronous Libraries in TypeScript
Asynchronous libraries can enhance the capabilities of your TypeScript projects. Here, we explore some popular libraries and how to leverage them.
10.1 Understanding Async Libraries
Asynchronous libraries provide additional utilities and tools to simplify common asynchronous operations, making them more convenient and easier to manage.
10.2 Utilizing Popular Asynchronous Libraries
typescript // Using Async.js for concurrent tasks import async from "async"; const tasks = [ (callback) => fetchData("https://api.example.com/data1", callback), (callback) => fetchData("https://api.example.com/data2", callback), (callback) => fetchData("https://api.example.com/data3", callback), ]; async.parallel(tasks, (error, results) => { if (error) { console.error("Error:", error.message); } else { console.log("Results:", results); } });
11. Handling Asynchronous Errors Gracefully
Error handling is a critical aspect of asynchronous programming. Proper error handling ensures that your application remains stable and provides meaningful feedback to users.
11.1 Global Error Handling in Asynchronous Code
typescript // Using Promise.catch for global error handling async function fetchData(url: string): Promise<any> { return fetch(url).then((response) => { if (!response.ok) { throw new Error(`Failed to fetch data: ${response.status} ${response.statusText}`); } return response.json(); }); } // Global error handler for unhandled Promise rejections process.on("unhandledRejection", (error) => { console.error("Unhandled Promise Rejection:", error.message); }); // Using the fetchData function with Async/Await async function getData() { try { const data = await fetchData("https://api.example.com/data"); console.log("Data:", data); } catch (error) { console.error("Error:", error.message); } } getData();
11.2 Using Try-Catch for Localized Error Handling
typescript async function getData() { try { const data = await fetchData("https://api.example.com/data"); console.log("Data:", data); } catch (error) { console.error("Error:", error.message); // Handle the error locally if necessary } }
12. Leveraging Asynchronous Programming for Scalability
Asynchronous programming can significantly impact the scalability and performance of your applications.
12.1 Asynchronous Processing in CPU-Intensive Tasks
typescript // CPU-intensive task: Finding the sum of large numbers function sumOfLargeNumbers(numbers: number[]): number { return numbers.reduce((sum, num) => sum + num, 0); } async function performTaskAsync() { const largeNumbers = Array.from({ length: 100000 }, (_, index) => index); const result = await new Promise<number>((resolve) => { setImmediate(() => resolve(sumOfLargeNumbers(largeNumbers))); }); console.log("Result:", result); } performTaskAsync();
12.2 Scaling Server-Side Operations with Asynchronous Techniques
typescript // Example of using Async/Await with Express.js import express from "express"; const app = express(); app.get("/data", async (req, res) => { try { const data = await fetchData("https://api.example.com/data"); res.json(data); } catch (error) { res.status(500).json({ error: error.message }); } }); app.listen(3000, () => { console.log("Server is running on port 3000"); });
13. Best Practices for Asynchronous Programming in TypeScript
Following best practices ensures that your asynchronous code is maintainable, reliable, and efficient.
13.1 Avoiding Nested Callbacks and Promises
typescript // Avoiding nested callbacks and Promises using Async/Await async function fetchData(): Promise<any> { const data1 = await fetchFirstData(); const data2 = await fetchSecondData(); const data3 = await fetchThirdData(); // ... rest of the code }
13.2 Handling Memory Leaks and Resource Management
typescript // Managing resources properly to avoid memory leaks async function fetchData(): Promise<any> { const resource = acquireResource(); try { const data = await fetchResourceData(resource); console.log("Data:", data); } finally { releaseResource(resource); } }
13.3 Ensuring Consistent Error Handling
typescript // Consistent error handling using Async/Await async function fetchData(url: string): Promise<any> { try { const response = await fetch(url); if (!response.ok) { throw new Error(`Failed to fetch data: ${response.status} ${response.statusText}`); } return response.json(); } catch (error) { console.error("Error:", error.message); throw error; } }
Conclusion
Asynchronous programming is a fundamental aspect of modern TypeScript development, enabling the creation of more responsive and scalable applications. We explored various techniques, from callbacks and Promises to Async/Await, and learned how to handle errors gracefully and ensure code quality through best practices. With this knowledge, you are now equipped to leverage the power of asynchronous programming to build efficient and robust TypeScript applications.
Asynchronous programming in TypeScript opens up a world of possibilities, making it easier to handle time-consuming tasks, manage resources efficiently, and create applications that can scale to meet the demands of the modern web. Embrace asynchronous programming in TypeScript, and watch your applications soar to new heights of performance and responsiveness!
Table of Contents
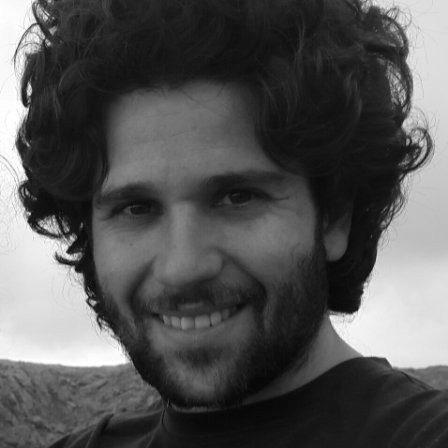
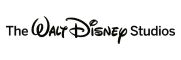