TypeScript and Augmented Reality: Building Immersive Experiences
Augmented Reality (AR) has transcended the realm of science fiction to become a powerful tool for creating immersive experiences. From gaming and education to industrial applications and navigation, AR has found its way into numerous aspects of our lives. As the demand for AR experiences continues to grow, developers are seeking efficient and reliable ways to build these captivating digital overlays. TypeScript, a statically-typed superset of JavaScript, is emerging as a valuable ally in the AR development landscape. In this blog, we’ll explore how TypeScript and AR come together to craft immersive digital worlds, complete with code samples and expert insights.
Table of Contents
1. Understanding Augmented Reality
1.1. What is Augmented Reality?
Augmented Reality is a technology that superimposes digital information, such as 3D models, animations, or information overlays, onto the real world. Unlike Virtual Reality (VR), which immerses users in a completely digital environment, AR enhances the real world with digital content. This blending of the physical and digital realms creates a unique and interactive experience for users.
1.2. Types of Augmented Reality
AR can be categorized into several types based on the level of immersion and interaction:
- Marker-Based AR: This type of AR relies on predefined markers, such as QR codes or images, to trigger digital content. When a device’s camera detects these markers, it overlays the associated content on top of them.
- Markerless AR: Also known as location-based or GPS-based AR, this type uses the device’s GPS, accelerometer, and other sensors to place digital content in a specific location or context.
- Projection-Based AR: In this type, digital content is projected onto physical surfaces. For example, interactive tabletops or walls can display digital information when users interact with them.
- Recognition-Based AR: This type uses object recognition technology to identify and track real-world objects, allowing digital content to interact with them.
1.3. The Rise of AR in Everyday Life
AR has seen a significant rise in adoption across various industries:
- Gaming: Games like Pokémon Go brought AR gaming into the mainstream, allowing players to hunt for virtual creatures in real-world locations.
- Education: AR is revolutionizing education by providing immersive learning experiences. Students can explore historical sites, anatomy, or complex scientific concepts through AR apps.
- Commerce: Retailers use AR to enable customers to visualize products in their real-world environment before making a purchase decision.
- Healthcare: Surgeons can use AR to enhance their precision during surgery by overlaying medical information on a patient’s body.
- Navigation: AR-based navigation apps offer real-time directions and information overlaid on the user’s view, making navigation more intuitive.
2. TypeScript: A Brief Overview
2.1. What is TypeScript?
TypeScript is an open-source programming language developed by Microsoft. It is a strict syntactical superset of JavaScript that adds optional static typing to the language. This means you can write JavaScript code in TypeScript, but with the added benefits of type checking and enhanced tooling support.
2.2. Advantages of Using TypeScript
Why choose TypeScript for AR development? Here are some compelling advantages:
- Type Safety: TypeScript enforces strong typing, catching type-related errors during development rather than at runtime. This results in more robust and reliable code.
- Enhanced Code Quality: TypeScript encourages best practices, leading to cleaner and more maintainable code. With features like interfaces and generics, you can define clear contracts for your code.
- Improved Tooling: TypeScript offers excellent tooling support with features like intelligent code completion, code navigation, and refactoring tools. This makes development faster and more efficient.
- Ecosystem Compatibility: TypeScript is compatible with existing JavaScript libraries and frameworks, allowing you to leverage the vast JavaScript ecosystem.
2.3. TypeScript for AR Development
AR development often involves complex interactions between real-world data and digital content. TypeScript’s type system can help manage these interactions more effectively. Here’s how TypeScript fits into the AR development process:
- Type Safety in AR: In AR, precise positioning and interaction with real-world objects are critical. TypeScript’s type system ensures that you handle these operations with confidence.
- Code Maintainability: AR projects can become intricate, and maintaining code quality is essential. TypeScript’s static typing helps in documenting your code and preventing common bugs.
- Framework Compatibility: Many AR frameworks and libraries support TypeScript, making it easier to integrate with existing projects.
3. Building AR Experiences with TypeScript
3.1. Setting Up the Development Environment
Before you start building AR experiences with TypeScript, you need to set up your development environment. Here’s a basic setup:
- Node.js and npm: Install Node.js and npm (Node Package Manager) to manage dependencies and run your TypeScript code.
bash # Install Node.js and npm https://nodejs.org/
TypeScript: Install TypeScript globally using npm.
bash # Install TypeScript globally npm install -g typescript
- Code Editor: Choose a code editor that supports TypeScript, such as Visual Studio Code, which provides excellent TypeScript integration out of the box.
- AR Framework: Select an AR framework or library that supports TypeScript. Some popular options include AR.js for web-based AR and ARCore/ARKit for mobile AR.
3.2. TypeScript and AR Frameworks
Once your development environment is set up, you can start working with AR frameworks in TypeScript. These frameworks provide the tools and APIs necessary to create AR experiences. Here’s an example of using AR.js, a web-based AR framework, with TypeScript:
typescript // Import AR.js library import * as AR from 'ar.js'; // Create an AR scene const scene = new AR.Scene(); // Define a marker with a 3D model const marker = new AR.Marker('marker.patt'); const model = new AR.Model('model.gltf'); // Add the model to the marker marker.add(model); // Add the marker to the scene scene.add(marker); // Render the AR scene scene.render();
In this code snippet, we import the AR.js library, create an AR scene, define a marker, add a 3D model to it, and render the scene. TypeScript’s type checking ensures that you use the library’s API correctly.
3.3. Coding in TypeScript for AR
As you start coding your AR experience, TypeScript provides helpful features for maintaining code quality and readability:
3.3.1. Interfaces
You can use interfaces to define clear contracts for your AR objects and components. For example:
typescript interface ARMarker { id: string; pattern: string; } interface ARModel { id: string; modelUrl: string; } function createMarker(marker: ARMarker): AR.Marker { return new AR.Marker(marker.pattern); } function createModel(model: ARModel): AR.Model { return new AR.Model(model.modelUrl); }
Interfaces help document the expected structure of objects and improve code understanding.
3.3.2. Enums
Enums are useful for defining a set of named constants. In AR development, you might use enums to represent different states or modes:
typescript enum ARMode { Idle, Tracking, Interactive, ... }
Enums make your code more self-explanatory and help prevent accidental value assignments.
3.3.3. Generics
Generics allow you to write flexible and reusable code. In AR, you may encounter scenarios where you need to work with various data types. Generics help you achieve this while maintaining type safety:
typescript function getObjectById<T>(id: string): T | undefined { // Logic to fetch the object by ID // ... }
Generics enable you to create functions that work with different types without sacrificing type safety.
4. TypeScript Best Practices for AR
While TypeScript offers many advantages for AR development, it’s essential to follow best practices to make the most of this powerful combination.
4.1. Type Safety and Code Quality
- Use Strong Typing: Leverage TypeScript’s static typing to define clear interfaces and types for your AR objects, improving code reliability.
- Avoid Any Type: Minimize the use of the any type, as it weakens type safety. Instead, use generics or type unions to handle dynamic data.
- Type Documentation: Add meaningful comments and documentation to your types and interfaces to enhance code understandability.
4.2. Debugging and Testing
- Debugging Tools: Make use of TypeScript-friendly debugging tools in your code editor to identify and fix issues efficiently.
- Unit Testing: Write unit tests for critical AR components to ensure they behave as expected, especially in complex AR interactions.
- Mock Data: Use mock data and fixtures for testing to simulate AR scenarios without relying on physical devices.
4.3. Managing State in AR Applications
- State Management: Implement a robust state management solution to handle the dynamic nature of AR applications. Libraries like Redux or Mobx can help manage state effectively.
- Lifecycle Management: Understand the lifecycle of AR components and objects, and clean up resources properly to prevent memory leaks.
- Performance Optimization: Optimize performance by minimizing unnecessary updates and rendering, especially in AR applications running on resource-constrained devices.
5. Case Study: TypeScript-Powered AR App
5.1. Concept and Planning
Let’s explore a case study of building an AR app using TypeScript. Suppose we’re creating an educational AR app that allows users to explore the solar system. Users can point their device at the sky to see detailed information about planets, constellations, and other celestial objects.
Requirements:
- Real-time tracking of the user’s location and orientation.
- Precise positioning of celestial objects in the sky.
- Interactive information overlays for each celestial object.
5.52. Development Process
- Setting Up the Environment: We set up our development environment with TypeScript, AR.js, and a 3D model library for the celestial objects.
- Designing AR Markers: We design markers that represent the celestial objects. These markers trigger the display of 3D models and information overlays.
- Creating AR Components: Using TypeScript, we create components for tracking the user’s location and orientation, positioning celestial objects, and rendering information overlays.
- Testing and Debugging: We thoroughly test our AR app on various devices, ensuring that it works smoothly and accurately. TypeScript helps us catch potential issues during development.
- Optimizing Performance: We optimize the app’s performance to provide a seamless AR experience, especially on mobile devices.
5.3. Key Takeaways
- TypeScript’s static typing and tooling support made the development process smoother and reduced the likelihood of runtime errors.
- Interfaces and enums helped us define clear contracts for AR objects and states.
- Unit testing with TypeScript allowed us to identify and fix issues early in the development cycle.
6. Future Trends and Challenges
6.1. WebAR and TypeScript
Web-based Augmented Reality (WebAR) is an exciting trend that allows users to experience AR directly through web browsers, eliminating the need for app installations. TypeScript’s compatibility with web technologies positions it as an ideal choice for WebAR development.
6.2. Challenges in AR Development
While TypeScript streamlines many aspects of AR development, challenges still exist:
- Device Compatibility: AR experiences must work seamlessly on a variety of devices, each with its capabilities and limitations.
- Performance: AR apps demand significant processing power. Ensuring smooth performance, especially on mobile devices, remains a challenge.
- Complex Interactions: Building complex interactions between the real world and digital content requires careful planning and development.
6.3. Preparing for the Future
To thrive in the ever-evolving AR landscape, developers should stay updated with the latest advancements and trends in both AR and TypeScript. This includes exploring new AR frameworks, leveraging TypeScript’s evolving features, and participating in the AR developer community.
Conclusion
The synergy between TypeScript and Augmented Reality is a powerful force in creating immersive experiences. TypeScript’s type safety, code quality enhancements, and compatibility with AR frameworks make it a valuable choice for AR development. By following best practices and staying attuned to emerging trends, developers can unlock the full potential of TypeScript and AR, crafting captivating and interactive digital worlds that bridge the gap between the real and virtual. As AR continues to enrich various aspects of our lives, the possibilities for TypeScript-powered AR experiences are boundless, promising a future of innovation and wonder.
Table of Contents
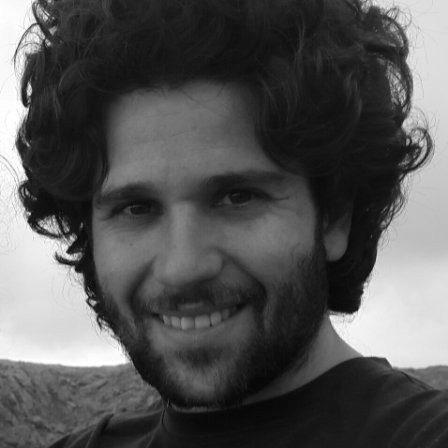
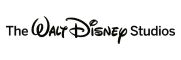